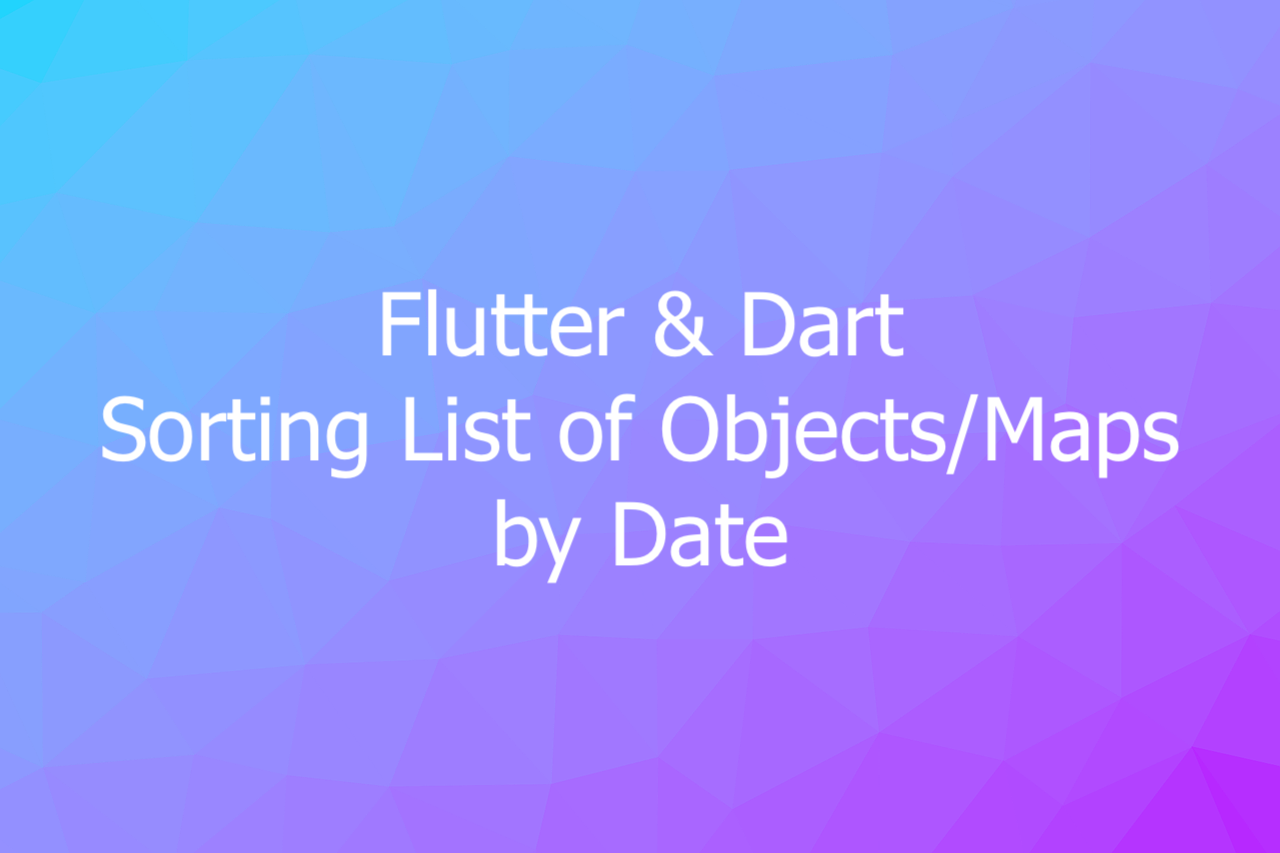
This practical, code-centric article will show you how to sort a list of objects/maps by date in Flutter. The point is to use the sort() method of the List class and pass a custom comparison function that uses the compareTo() method of the DateTime class:
myList.sort ((a, b) {
// for ascending order
return a.date.compareTo (b.date);
// for descending order
// return b.date.compareTo (a.date);
});
For more clarity, see the following complete examples.
Sorting a List of Objects by Date
Let’s say you have a list of users. The information of each user is stored in an object, and your boss asks you to reorder these users by their join date. The example below will show you to solve this:
// this class is used to create User objects
class User {
final String name;
final String email;
final DateTime joinDate;
User({required this.name, required this.email, required this.joinDate});
@override
String toString() {
return 'User{name: $name, email: $email, joinDate: $joinDate}';
}
}
void main() {
// list of users
List<User> users = [
User(
name: 'Jack',
email: '[email protected]',
joinDate: DateTime(2022, 6, 1)),
User(
name: 'John',
email: '[email protected]',
joinDate: DateTime(2020, 1, 1)),
User(
name: 'Jane',
email: '[email protected]',
joinDate: DateTime(2021, 5, 12)),
User(
name: 'Jill',
email: '[email protected]',
joinDate: DateTime(2023, 7, 1))
];
// sort by joinDate: ascending
final sortedUsersAsc = users.map((user) => user).toList()
..sort((a, b) => a.joinDate.compareTo(b.joinDate));
// sort by joinDate: descending
final sortedUsersDesc = users.map((user) => user).toList()
..sort((a, b) => b.joinDate.compareTo(a.joinDate));
print('Sorted by joinDate: ascending');
sortedUsersAsc.forEach((user) => print(user.toString()));
print('Sorted by joinDate: descending');
sortedUsersDesc.forEach((user) => print(user.toString()));
}
Output:
Sorted by joinDate: ascending
User{name: John, email: [email protected], joinDate: 2020-01-01 00:00:00.000}
User{name: Jane, email: [email protected], joinDate: 2021-05-12 00:00:00.000}
User{name: Jack, email: [email protected], joinDate: 2022-06-01 00:00:00.000}
User{name: Jill, email: [email protected], joinDate: 2023-07-01 00:00:00.000}
Sorted by joinDate: descending
User{name: Jill, email: [email protected], joinDate: 2023-07-01 00:00:00.000}
User{name: Jack, email: [email protected], joinDate: 2022-06-01 00:00:00.000}
User{name: Jane, email: [email protected], joinDate: 2021-05-12 00:00:00.000}
User{name: John, email: [email protected], joinDate: 2020-01-01 00:00:00.000}
Sorting a List of Maps by Date
In this example, the information about a user is stored in a map instead of an object. Besides that, the join date of a user is a string, not a DateTime object. Therefore, we will need to parse it into a DateTime object first using the DateTime.parse() or DateTime.tryParse() method before performing the comparison.
The code:
void main() {
final List<Map<String, dynamic>> users = [
{
"name": "Jack",
"email": "[email protected]",
"joinDate": "2023-06-01",
},
{
"name": "John",
"email": "[email protected]",
"joinDate": "2020-01-01",
},
{
"name": "Jane",
"email": "[email protected]",
"joinDate": "2021-05-12",
},
{
"name": "Jill",
"email": "[email protected]",
"joinDate": "2023-07-01",
}
];
// sort users by joinDate: ascending
final sortedUsersAsc = users.map((user) => user).toList()
..sort((a, b) =>
DateTime.parse(a["joinDate"]).compareTo(DateTime.parse(b["joinDate"])));
// sort users by joinDate: descending
final sortedUsersDesc = users.map((user) => user).toList()
..sort((a, b) =>
DateTime.parse(b["joinDate"]).compareTo(DateTime.parse(a["joinDate"])));
print("Sorted users by ascending joinDate:");
print(sortedUsersAsc);
print("Sorted users by descending joinDate:");
print(sortedUsersDesc);
}
Output:
Sorted users by ascending joinDate:
[
{name: John, email: [email protected], joinDate: 2020-01-01},
{name: Jane, email: [email protected], joinDate: 2021-05-12},
{name: Jack, email: [email protected], joinDate: 2023-06-01},
{name: Jill, email: [email protected], joinDate: 2023-07-01}
]
Sorted users by descending joinDate:
[
{name: Jill, email: [email protected], joinDate: 2023-07-01},
{name: Jack, email: [email protected], joinDate: 2023-06-01},
{name: Jane, email: [email protected], joinDate: 2021-05-12},
{name: John, email: [email protected], joinDate: 2020-01-01}
]
That’s it. Further reading:
- Working with ReorderableListView in Flutter
- Flutter: Adding a Border to an Icon Button (2 Approaches)
- Flutter StreamBuilder: Tutorial & Examples (Updated)
- Working with Timer and Timer.periodic in Flutter
- Using GetX to make GET/POST requests in Flutter
- Flutter & Dart: Get a list of dates between 2 given dates
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.
There is definately a lot to find out about this subject. I like all the points you made
Great information shared.. really enjoyed reading this post thank you author for sharing this post .. appreciated
Hi there to all, for the reason that I am genuinely keen of reading this website’s post to be updated on a regular basis. It carries pleasant stuff.
I am truly thankful to the owner of this web site who has shared this fantastic piece of writing at at this place.
naturally like your web site however you need to take a look at the spelling on several of your posts. A number of them are rife with spelling problems and I find it very bothersome to tell the truth on the other hand I will surely come again again.