This article lists the most popular packages used for state management in Flutter applications that contain multiple screens. The top 2 criteria for us to include a package on this list are the number of stars it receives from GitHub users and the number of likes it receives on pub.dev.
GetX (aka Get)
- GitHub stars: 9,8k+
- Likes (on pub.dev): 13.8k+
- Written in: Dart, C++, CMake
- License: MIT
- Links: GitHub repo | Pub
The package combines high-performance state management, intelligent dependency injection, and route management quickly and practically. Although it provides a multitude of features, each of these features is in separate containers and is only started after use. If you only use State Management, only State Management will be compiled. If you only use routes, nothing from the state management will be compiled.

The package is focused on performance and minimum consumption of resources. It doesn’t use Streams or ChangeNotifier. GetX has two different state managers: the simple state manager (GetBuilder) and the reactive state manager (GetX/Obx). It turns reactive programming into something quite simple:
- You won’t need to create StreamControllers.
- You won’t need to create a StreamBuilder for each variable
- You will not need to create a class for each state.
- You will not need to create a get for an initial value.
- You will not need to use code generators
You can learn more about GetX in the following tutorials:
- Using GetX (Get) for State Management in Flutter
- Using GetX (Get) for Navigation and Routing in Flutter
- Using GetX to make GET/POST requests in Flutter
- Flutter: Uploading Files with GetConnect (GetX)
Provider
- GitHub stars: 5k+
- Pub likes: 9.6k+
- Written in: Dart
- License: MIT
- Links: GitHub repo | Pub

This package has been known and used widely for a long time. It is loved for its simplicity, neatness, and ease of learning by Flutter beginners. The package gives you:
- simplified allocation/disposal of resources
- lazy-loading
- a largely reduced boilerplate over making a new class every time
- dev tools friendly
- a common way to consume these InheritedWidgets
- increased scalability for classes with a listening mechanism that grows exponentially in complexity
The Provider package also uses concepts that are applicable to every other approach. You can see a detailed guide on implementing Provider in action in this article.
Flutter BloC
- GitHub stars: 11.3k+
- Pub likes: 6.6k+
- Written in: Dart, TypeScript, Kotlin, CMake, C++
- License: MIT
- Links: GitHub repo | Pub
BloC makes it easy to implement the Business Logic Component design pattern, which separates presentation from business logic.

A Cubit is a class that extends BlocBase and can be extended to manage any type of state. Cubit requires an initial state which will be the state before emit has been called. The current state of a cubit can be accessed via the state getter, and the state of the cubit can be updated by calling emit with a new state.
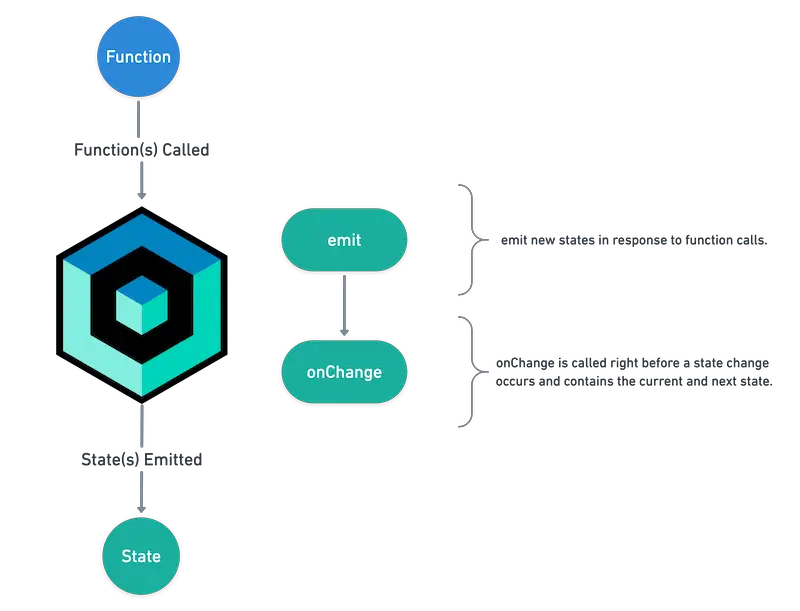
State changes in cubit begin with predefined function calls, which can use the emit method to output new states. onChange is called on each state change and contains the current and next state.
Riverpod
- GitHub stars: 5.6k+
- Likes: 3.2k+
- Written in: Dart, Javascript
- Links: GitHub repo | Pub (riverpod) | Pub (flutter_riverpod)
This package gives you a simple way to access the state from anywhere in your application.

It’s a state-management library that catches programming errors at compile time rather than at runtime, removes nesting for listening/combining objects, and ensures that your code is testable.
Two related packages that you can use instead are flutter_riverpod (recommended) and hooks_riverpod (using riverpod with hooks to reduce a lot of boilerplate code).
Get It (completely different from GetX)
- GitHub stars: 1.2k+
- Likes: 3.8k+
- Written in: Dart
- Links: GitHub repo | Pub
The name of this package is GetIt, and it’s published by Flutter Community (an organization aimed at providing a central place for community-made Flutter packages and content to live), not to be confused with the Get library above.

GetIt is a simple direct Service Locator that allows you to decouple the interface from a concrete implementation and to access the concrete implementation from everywhere in your application. It can be used instead of InheritedWidget or Provider to access objects, for example, from your UI:
- Accessing service objects like REST API clients or databases so that they can easily be mocked.
- Accessing View/AppModels/Managers/BLoCs from Flutter Views.
Mobx
- GitHub stars: 2.3k+
- Likes: 1.2k+
- Written in: Dart
- License: MIT
- Links: GitHub repo | Pub
MobX is a state management library that makes it simple to connect the reactive data of your application with the UI. This wiring is completely automatic and feels very natural. As the application developer, you focus purely on what reactive data needs to be consumed in the UI (and elsewhere) without worrying about keeping the two in sync.
The diagram below describes the core concept of MobX:

It’s not really magic, but it does have some smarts around what is being consumed (observables) and where (reactions) and automatically tracks it for you. When the observables change, all reactions are re-run.
Recap
We’ve walked through the most popular open-source packages used for state management in Flutter. You may wonder why Redux doesn’t get a place on the list. The fact is that it isn’t often used in Flutter as it is in React or React Native.
Flutter’s wonderful, and there’re many things to learn about it. Don’t stop moving and keep yourself on the rail by taking a look at the following articles:
- Understanding Typedefs (Type Aliases) in Dart and Flutter
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Flutter and Firestore Database: CRUD example
- Flutter: ValueListenableBuilder Example
- Flutter StatefulBuilder example
- Flutter AnimatedList – Tutorial and Examples
- Flutter SliverList – Tutorial and Example
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.
Thank you for your article and I think there’s also another solution that can be considered on the list: https://pub.dev/packages/states_rebuilder