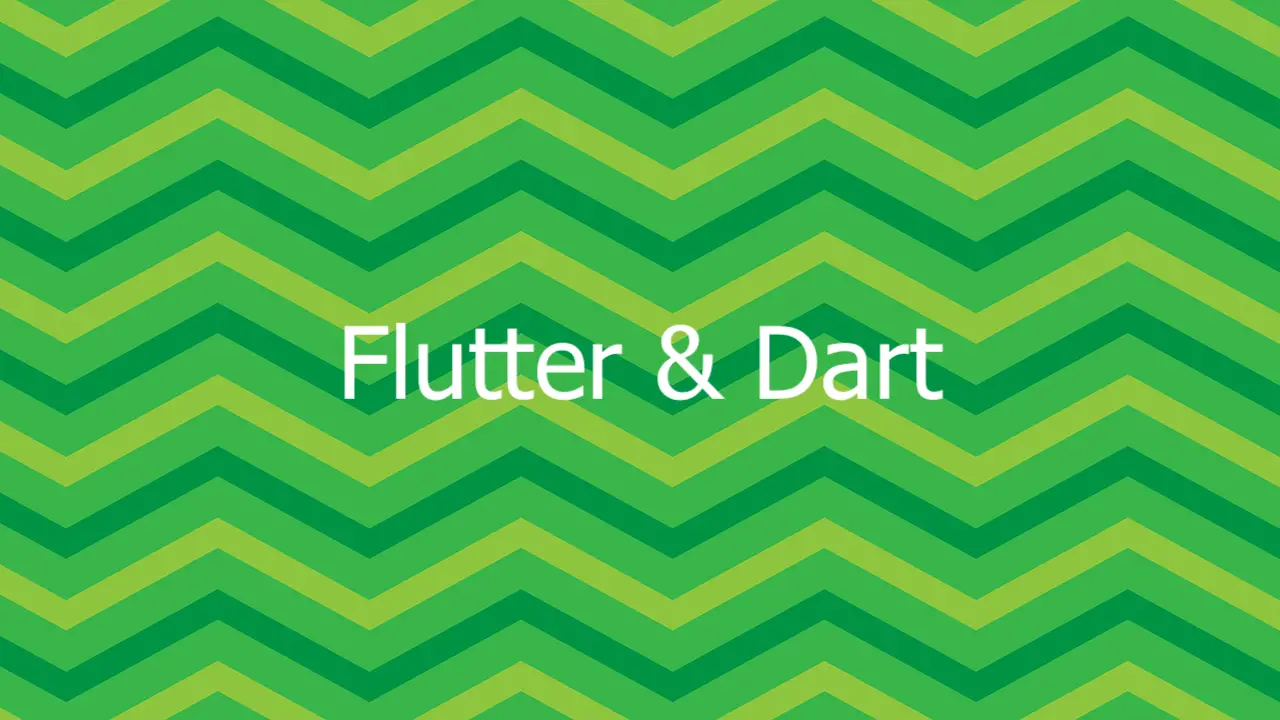
When developing applications with Flutter and Dart, there might be situations where you need to extract a list of dates between two given dates. This concise and straightforward article will show you a couple of different ways to do so.
Using a For loop
In this example, we’ll define a function named getDaysInBetween that takes 2 arguments, startDate and endDate, and returns a list of dates between them (including the bounds):
// kindacode.com
// main.dart
// define a fuction to get days in between two dates
List<DateTime> getDaysInBetween(DateTime startDate, DateTime endDate) {
List<DateTime> days = [];
for (int i = 0; i <= endDate.difference(startDate).inDays; i++) {
days.add(startDate.add(Duration(days: i)));
}
return days;
}
// try it out
void main() {
DateTime startDate = DateTime(2023, 4, 1);
DateTime endDate = DateTime(2023, 4, 10);
List<DateTime> days = getDaysInBetween(startDate, endDate);
// print the result without time
days.forEach((day) {
print(day.toString().split(' ')[0]);
});
}
Output:
2023-04-01
2023-04-02
2023-04-03
2023-04-04
2023-04-05
2023-04-06
2023-04-07
2023-04-08
2023-04-09
2023-04-10
See also: Ways to Format DateTime in Flutter
Using List.generate()
You can get a list of dates between two given dates by using the List.generate() method and pass the number of days between the start and end date as the length parameter.
Example:
// kindacode.com
// main.dart
// define a fuction to get days in between two dates
List<DateTime> getDaysInBetween(DateTime startDate, DateTime endDate) {
final daysToGenerate = endDate.difference(startDate).inDays + 1;
return List.generate(daysToGenerate, (i) => startDate.add(Duration(days: i)));
}
// try it out
void main() {
DateTime startDate = DateTime(2023, 4, 1);
DateTime endDate = DateTime(2023, 4, 5);
List<DateTime> days = getDaysInBetween(startDate, endDate);
// print the result without time
days.forEach((day) {
print(day.toString().split(' ')[0]);
});
}
Output:
2023-04-01
2023-04-02
2023-04-03
2023-04-04
2023-04-05
That’s it. Further reading:
- How to add/remove a duration to/from a date in Dart
- How to Subtract two Dates in Flutter & Dart
- Flutter Stream.periodic: Tutorial & Example
- Flutter StreamBuilder: Tutorial & Examples (Updated)
- 2 ways to convert DateTime to time ago in Flutter
- Working with Cupertino Date Picker in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.