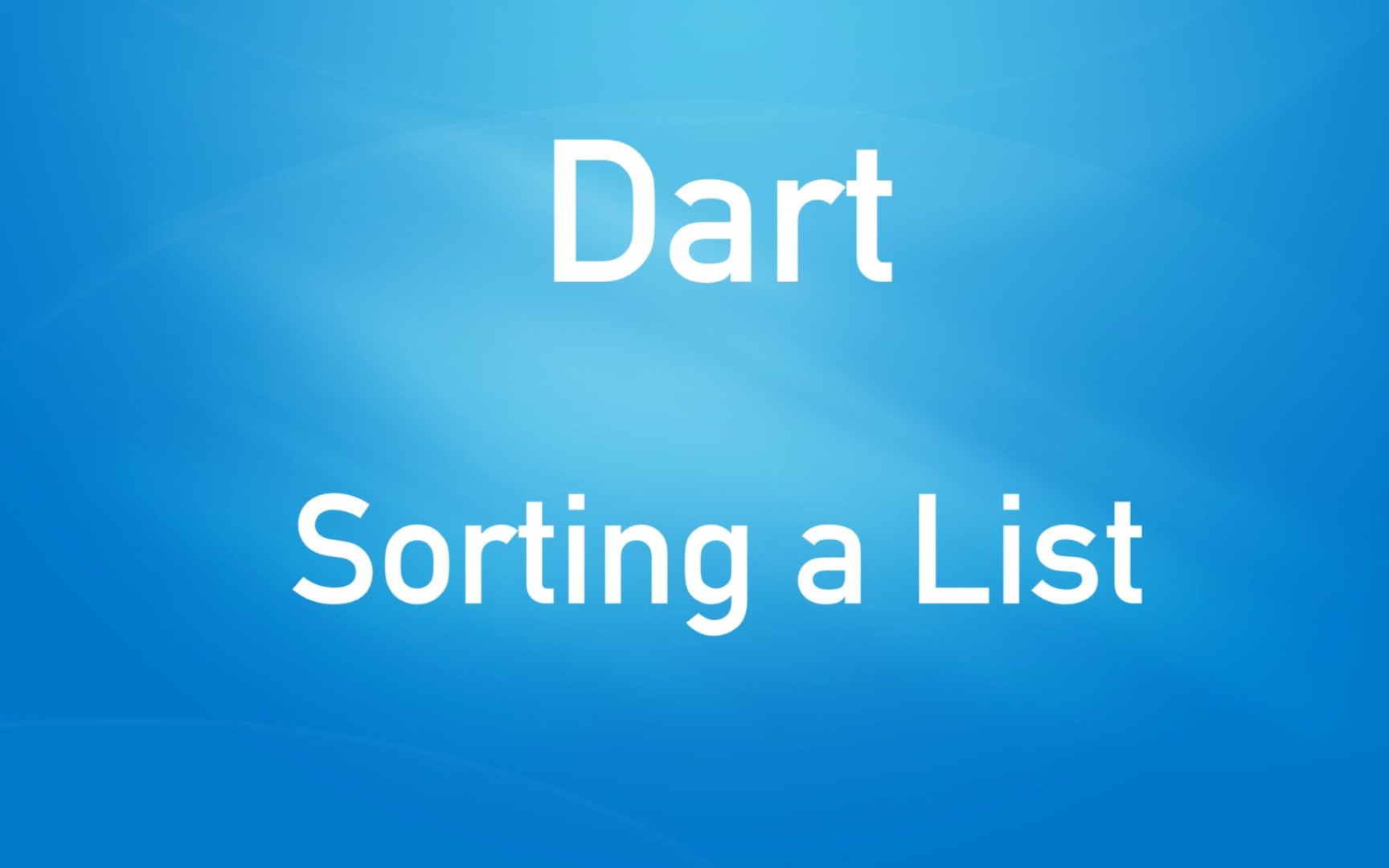
In this article, we’ll walk through a couple of examples of sorting lists in Dart (and Flutter as well), a common task that you may have to deal with in the vast majority of your projects. The examples are in order from easy to advanced, from simple to complex, and cover most scenarios you might encounter in real life.
Sorting a List of Numbers
Using the sort() method helps us to quickly sort a list of numbers in ascending or descending order. Note that this method does not return a new list but manipulates the original list.
The code:
import 'package:flutter/foundation.dart';
void main() {
List<double> myNumbers = [1, 2.5, 3, 4, -3, 5.4, 7];
myNumbers.sort();
if (kDebugMode) {
print('Ascending order: $myNumbers');
print('Descending order ${myNumbers.reversed}');
}
}
Output:
Ascending order: [-3.0, 1.0, 2.5, 3.0, 4.0, 5.4, 7.0]
Descending order (7.0, 5.4, 4.0, 3.0, 2.5, 1.0, -3.0)
Sorting a List of Strings
This example is similar to the first one. We use the sort() method to sort the string elements of a list in alphabetical order (from A to Z or from Z to A).
The code:
import 'package:flutter/foundation.dart';
void main() {
List<String> myFruits = [
'apple',
'coconut',
'banana',
'strawberry',
'water melon',
'pear',
'plum'
];
myFruits.sort();
if (kDebugMode) {
print('Ascending order (A to Z): $myFruits');
print('Descending order (Z to A): ${myFruits.reversed}');
}
}
Output:
Ascending order (A to Z): [apple, banana, coconut, pear, plum, strawberry, water melon]
Descending order (Z to A): (water melon, strawberry, plum, pear, coconut, banana, apple)
Sorting a List of Maps
Sorting a list of maps is a little bit more complex. We need to use the sort() method and the compareTo() method.
The small program below sorts a list of dummy products in ascending and descending orders of sale price.
The code:
import 'package:flutter/foundation.dart';
void main() {
List<Map<String, dynamic>> myProducts = [
{"name": "Shoes", "price": 100},
{"name": "Pants", "price": 50},
{"name": "Book", "price": 10},
{"name": "Lamp", "price": 40},
{"name": "Fan", "price": 200}
];
// Selling price from low to high
myProducts.sort((a, b) => a["price"].compareTo(b["price"]));
// Selling price from high to low
myProducts.sort((a, b) => b["price"].compareTo(a["price"]));
if (kDebugMode) {
print('Low to hight in price: $myProducts');
print('High to low in price: $myProducts');
}
}
Output:
Low to hight in price: [{name: Book, price: 10}, {name: Lamp, price: 40}, {name: Pants, price: 50}, {name: Shoes, price: 100}, {name: Fan, price: 200}]
High to low in price: [{name: Fan, price: 200}, {name: Shoes, price: 100}, {name: Pants, price: 50}, {name: Lamp, price: 40}, {name: Book, price: 10}]
Sorting a List of Objects
Similar to sorting a list of maps, you can sort a list of objects by the property values with the sort() and compareTo() methods.
Let’s say your app has gained some users and you want to sort them by age. The program below will help you.
The code:
import 'package:flutter/foundation.dart';
class User {
String name;
int age;
User(this.name, this.age);
@override
toString() {
return ('$name - $age years old \n');
}
}
void main() {
List<User> users = [
User('Plumber', 10),
User('John Doe', 50),
User('Pipi', 4),
User('Invisible Man', 31),
User('Electric Man', 34)
];
// Age from small to large
users.sort((a, b) => a.age.compareTo(b.age));
debugPrint('Age ascending order: ${users.toString()}');
// Age from large to small
users.sort((a, b) => b.age.compareTo(a.age));
debugPrint('Age descending order: ${users.toString()}');
}
Output:
Age ascending order: [Pipi - 4 years old
, Plumber - 10 years old
, Invisible Man - 31 years old
, Electric Man - 34 years old
, John Doe - 50 years old
]
Age descending order: [John Doe - 50 years old
, Electric Man - 34 years old
, Invisible Man - 31 years old
, Plumber - 10 years old
, Pipi - 4 years old
]
Sorting a List using the Comparable abstract class
This example does what the previous example does but with a different methodology. We extend the Comparable abstract class and override the compareTo() method. The sorting logic is moved into the User class.
The code:
import 'package:flutter/foundation.dart';
class User extends Comparable {
String name;
int age;
User(this.name, this.age);
@override
toString() {
return ('$name - $age years old \n');
}
@override
int compareTo(other) {
// age < other.age
if (age < other.age) {
return -1;
}
// age > other.age
if (age > other.age) {
return 1;
}
// age == other.age
return 0;
}
}
void main() {
List<User> users = [
User('Plumber', 10),
User('John Doe', 50),
User('Pipi', 4),
User('Invisible Man', 31),
User('Electric Man', 34)
];
// Age from small to large
users.sort();
debugPrint('Age ascending order: ${users.toString()}');
}
Output:
Age ascending order: [Pipi - 4 years old
, Plumber - 10 years old
, Invisible Man - 31 years old
, Electric Man - 34 years old
, John Doe - 50 years old
The advantage of this approach is that you don’t have to rewrite the sort algorithm many times, but the downside is that it sometimes makes your code more confusing and redundant.
Conclusion
We’ve gone over a few examples of sorting a list in Dart, and you’ve learned more than one technique to get the job done. Keep the ball rolling and continue learning more exciting stuff by taking a look at the following articles:
- Dart: Extract a substring from a given string (advanced)
- A Complete Guide to Underlining Text in Flutter
- Flutter and Firestore Database: CRUD example
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Removing duplicate items from a list in Dart
- Flutter AnimatedList – Tutorial and Examples
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.
Thank you