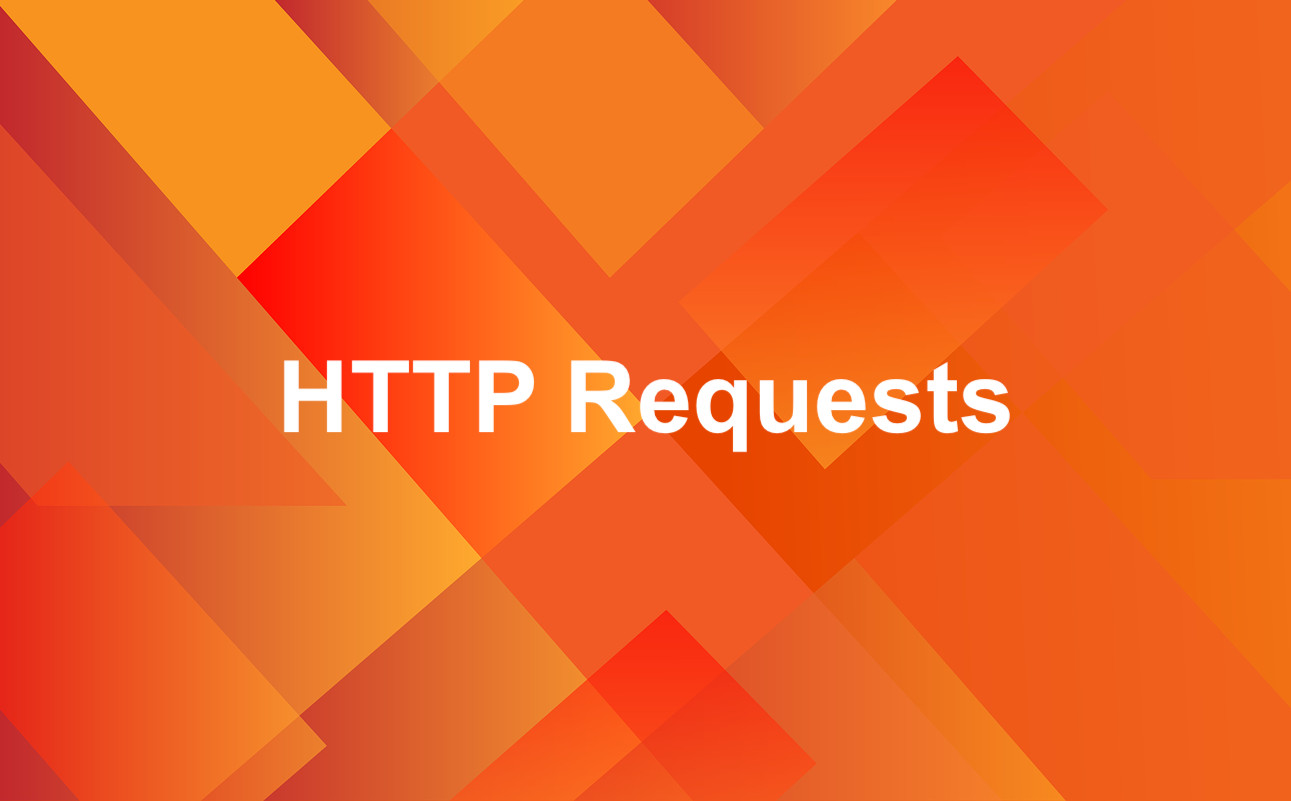
This article walks you through a list of the best open-source packages that can help you (and your team) a lot when making HTTP requests in Flutter applications. Without any further ado, let’s explore the things that matter.
Http
- Pub likes: 7.3k+
- GitHub stars: 1k+
- License: BSD-3-Clause
- Written in: Dart
- Links: Pub page | GitHub repo | Official docs
This package is published by the Dart team currently the most-liked HTTP packages on pub.dev. It provides a high-level API that can make your life much easier when dealing with network tasks. You can install it by running the following command:
flutter pub add http
Sample usage:
import 'package:http/http.dart' as http;
void sendPostRequest() async {
final url = Uri.parse('https://test.kindacode.com/api/v3/');
final response = await http.post(url,
body: {
'email': '[email protected]',
'name': 'Mr Happy'
}
);
print('Response status: ${response.statusCode}');
print('Response body: ${response.body}');
}
The plugin supports retrying requests. You can use the RetryClient class to retry failing requests:
import 'package:http/http.dart' as http;
import 'package:http/retry.dart';
Future<void> bootstrap() async {
final client = RetryClient(http.Client());
try {
print(await client.read(Uri.parse('http://test.kindacode.com/api/v3/')));
} finally {
client.close();
}
}
Dio
- Pub likes: 6.6k+
- GitHub stars: 12.2k+
- License: MIT
- Written in: Dart
- Links: Pub page | GitHub repo | API reference
Dio is the fastest-growing HTTP package in the Flutter ecosystem. It’s super powerful and simple to use. The package brings us many extremely useful features:
- Global Configuration
- Interceptors
- FormData
- Request Cancellation
- Retrying Requests
- File Downloading
- Timeout
- Https certificate verification
- Http2
You can install Dio by running:
flutter pub add dio
And use it without writing much code:
import 'package:dio/dio.dart';
void getData() async {
try {
var response = await Dio().get('http://www.example.com');
print(response);
} catch (e) {
print(e);
}
}
Dio has several extensions:
- dio_cookie_manager: For working with cookie
- dio_http2_adapter: For http2 stuff
Retrofit
- Pub likes: 1.6k+
- GitHub stars: 1k+
- License: MIT
- Written in: Dart, Ruby, Swift, Kotlin, Python
- Links: Pub page | GitHub repo | API reference
Retrofit is a type of conversion dio client generator. It lets you write code with @Something like decorators in TypeScript. For example:
@JsonSerializable()
class MyClass {}
@PATCH("your-api-endpoint")
Future<Task> updateTaskPart(
@Path() String id, @Body() Map<String, dynamic> map);
@PUT("your-api-endpoint")
Future<Task> updateTask(@Path() String id, @Body() Task task);
@DELETE("your-api-endpoint")
Future<void> deleteTask(@Path() String id);
@POST("your-api-endpoint")
Future<Task> createTask(@Body() Task task);
For more details, please see retrofit’s docs.
Chopper
- Pub likes: 755+
- GitHub stars: 700+
- License: MIT
- Written in: Dart, Shell
- Links: Pub page | GitHub repo | API reference
Similar to Retrofit, Chopper is another HTTP client generator for Dart and Flutter. You will need to install chopper, chopper_generator, and build_runner. For more detailed information about using Chopper, please see its official documentation.
GetX (aka Get)
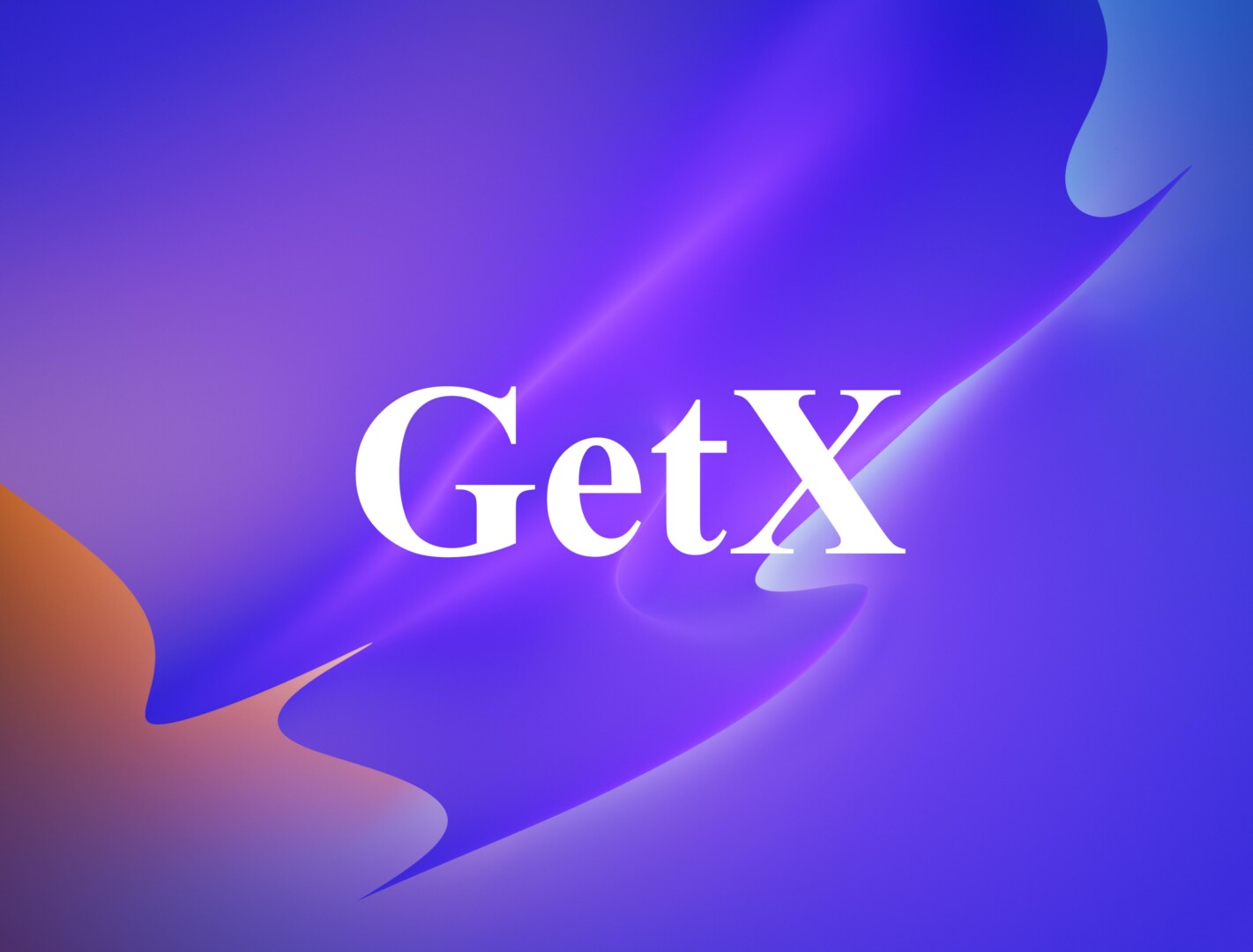
- Pub likes: 14k+
- GitHub stars: 9.8k+
- License: MIT
- Written in: Dart, C++, CMake, HTML, Shell
- Links: Pub page | GitHub repo | Docs
GetX is a comprehensive library that provides a wide range of features, including state management, navigation and routing, and network communication (HTTP & WebSocket). If you only care about making HTTP requests, only things related to this feature are compiled so that the size of your app will be optimized. What you need to do is to create an instance of the GetConnect class provided by GetX, then call the get/post/put/delete method, like so:
void _sendGetRequest() async {
final _connect = GetConnect();
final response =
await _connect.get('https://jsonplaceholder.typicode.com/posts/1');
if (kDebugMode) {
print(response.body);
}
}
See detailed tutorials about GetX:
- Using GetX to make GET/POST requests in Flutter
- Flutter: Uploading Files with GetConnect (GetX)
- Using GetX (Get) for Navigation and Routing in Flutter
- Using GetX (Get) for State Management in Flutter
Wrapping Up
We’ve gone over the list of most-liked HTTP client packages for Flutter. If you’d like to gain more knowledge about Flutter and explore more new and fascinating stuff to work better with the framework, take a look at the following articles:
- Creating Masonry Layout in Flutter with Staggered Grid View
- Flutter & Hive Database: CRUD Example
- How to encode/decode JSON in Flutter
- Sorting Lists in Dart and Flutter (5 Examples)
- Flutter and Firestore Database: CRUD example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.
Please, NEVER use GetX. Never.
Why ?
– getx makes you learn getx, not flutter – getx is to flutter what wordpress is to web development – getx is a very large library but has very little documentation – getx makes you avoid learning a crucial part of flutter (the buildcontext) – getx makes you learn bad… Read more »
at work,which network Request libraries is often used ?
http. Indeed
fwef