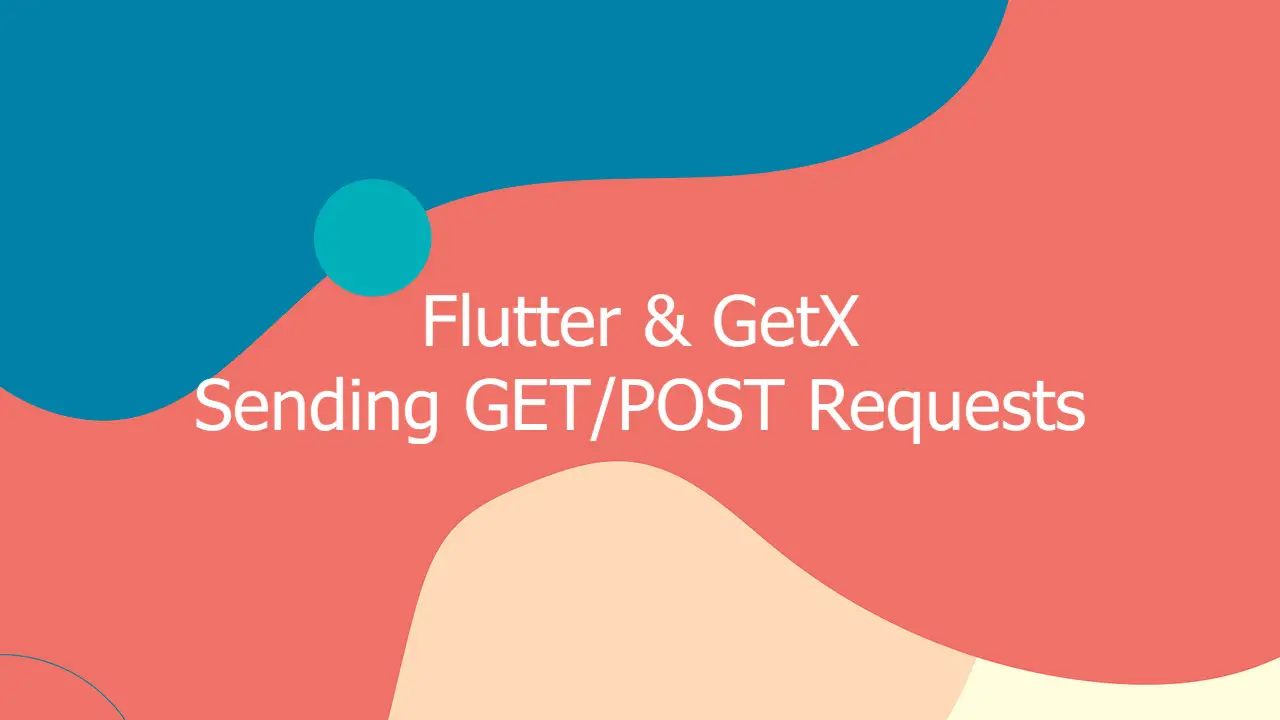
GetX is a massively functional and open-source Flutter library that is the most popular these days, with over 10,000 likes on pub.dev. Although GetX provides a wide range of features, each feature is contained in a separate container, and only the ones that you use in your application are compiled. Therefore, it does not increase your app size unnecessarily. This short article shows you how to make POST and GET requests with the GetConnect class provided by the library. You will be surprised because the amount of code to write is extremely small.
Here’re the steps:
1. To get started, you need to install GetX by performing the following command:
flutter pub add get
2. Then import it into your Dart code:
import 'package:get/get.dart';
3. The next step is to create an instance of the GetConnect class:
final _connect = GetConnect();
4. Sending a Get request to a mocking API endpoint like so (thanks to the Typi Code team for this):
void _sendGetRequest() async {
final response =
await _connect.get('https://jsonplaceholder.typicode.com/posts/1');
if (kDebugMode) {
print(response.body);
}
}
5. Making Post requests:
void _sendPostRequest() async {
final response = await _connect.post(
'https://jsonplaceholder.typicode.com/posts',
{
'title': 'one two three',
'body': 'four five six seven',
'userId': 1,
},
);
if (kDebugMode) {
print(response.body);
}
}
Full Example:
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter/foundation.dart';
import 'package:get/get.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const KindaCodeDemo(),
);
}
}
class KindaCodeDemo extends StatefulWidget {
const KindaCodeDemo({Key? key}) : super(key: key);
@override
State<KindaCodeDemo> createState() => _KindaCodeDemoState();
}
class _KindaCodeDemoState extends State<KindaCodeDemo> {
// Initialize an instance of GetConnect
final _connect = GetConnect();
// send Get request
// and get the response
void _sendGetRequest() async {
final response =
await _connect.get('https://jsonplaceholder.typicode.com/posts/1');
if (kDebugMode) {
print(response.body);
}
}
// send Post request
// You can somehow trigger this function, for example, when the user clicks on a button
void _sendPostRequest() async {
final response = await _connect.post(
'https://jsonplaceholder.typicode.com/posts',
{
'title': 'one two three',
'body': 'four five six seven',
'userId': 1,
},
);
if (kDebugMode) {
print(response.body);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('KindaCode.com')),
body: Padding(
padding: const EdgeInsets.all(30),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
ElevatedButton(
onPressed: _sendGetRequest,
child: const Text('Send GET Request')),
ElevatedButton(
onPressed: _sendPostRequest,
child: const Text('Send POST Request')),
],
),
),
);
}
}
When running the code, you’ll have an app like this:
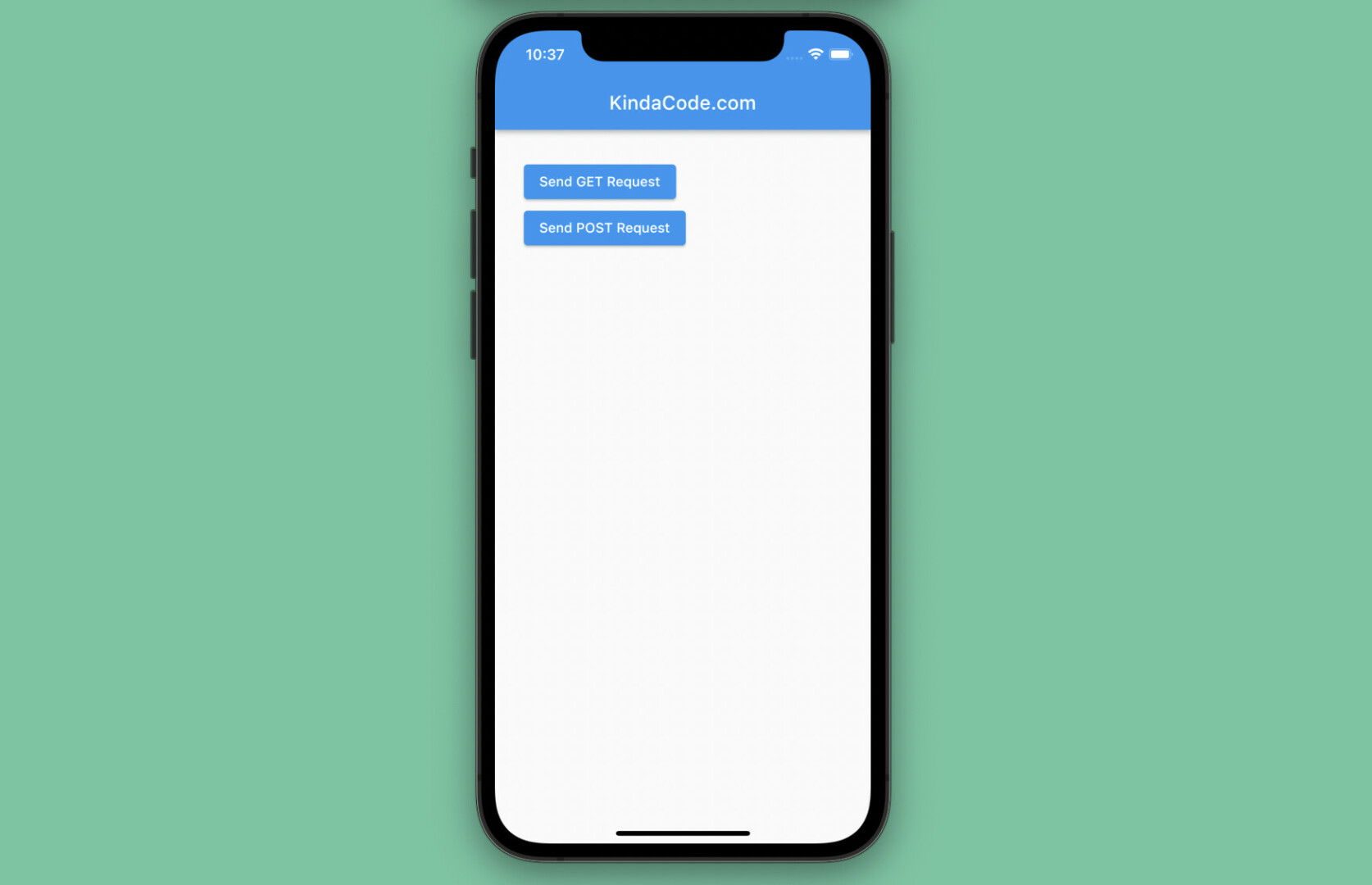
Press the 2 buttons and check your terminal to see the output. Here’s mine:
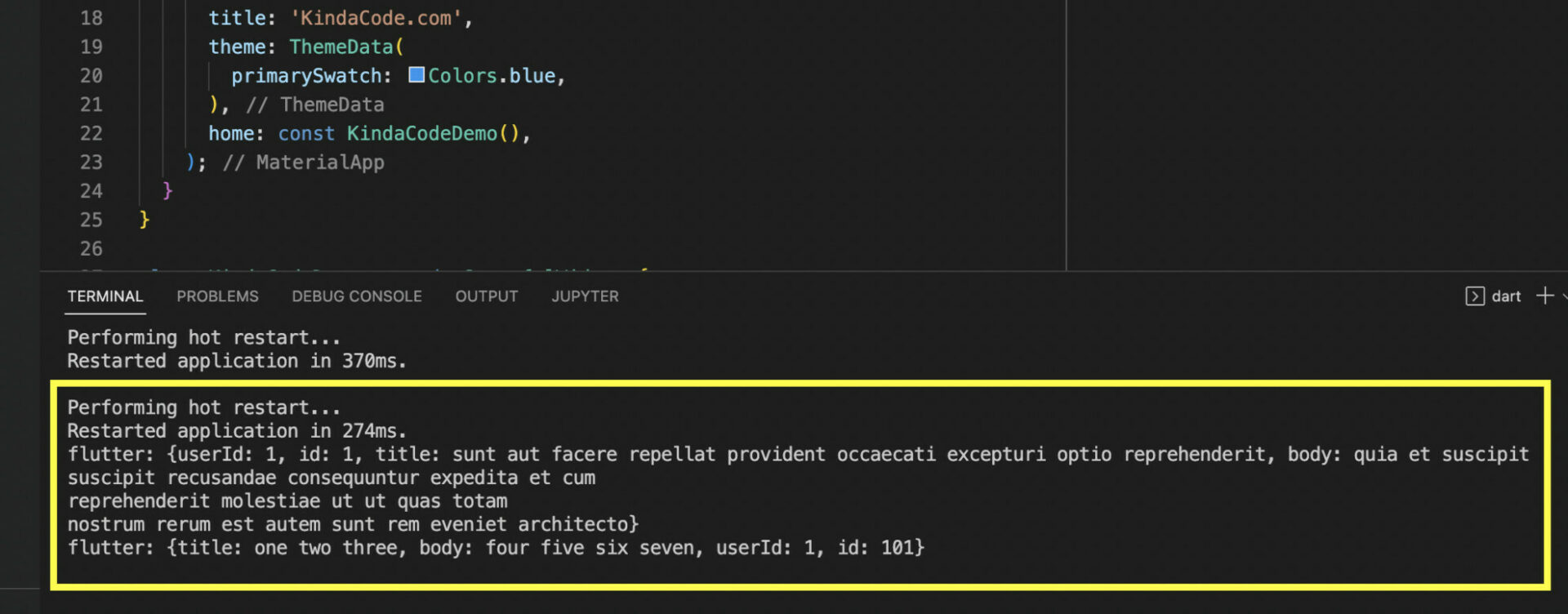
That’s it. Further reading about GetX and network stuff in Flutter by taking a look at the following articles:
- Flutter: Uploading Files with GetConnect (GetX)
- Using GetX (Get) for Navigation and Routing in Flutter
- Using GetX (Get) for State Management in Flutter
- 2 Ways to Fetch Data from APIs in Flutter
- Best Libraries for Making HTTP Requests in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.