This practical article walks you through a couple of examples that demonstrate how to make rounded-corners text fields in Flutter.
Using OutlineInputBorder borderRadius
You can create a rounded TextField by setting the borderRadius property of the OutlineInputBorder class to BorderRadius.circular(double radius), like this:
TextField(
decoration: InputDecoration(
hintText: 'KindaCode.com',
contentPadding: const EdgeInsets.all(15),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(30))),
onChanged: (value) {
// do something
},
),
Screenshot:
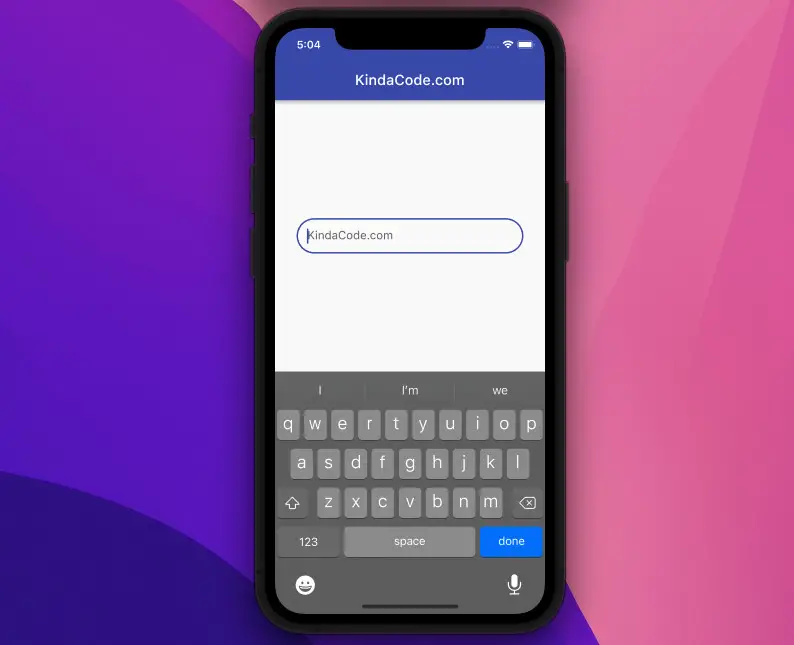
The full source code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Padding(
padding: const EdgeInsets.all(30),
child: Center(
child: TextField(
decoration: InputDecoration(
hintText: 'KindaCode.com',
contentPadding: const EdgeInsets.all(15),
border: OutlineInputBorder(
borderRadius: BorderRadius.circular(30))),
onChanged: (value) {
// do something
},
),
),
),
);
}
}
Wrap TextField inside a Container
Our strategy is to remove TextField’s border and wrap it inside a Container with circular BorderRadius (this Container itself should be wrapped by a Center widget or another Container with alignment property). The advantage of this approach is that you can easily adjust the width and height of the TextFiled by setting the width and height of the parent Container.
Screenshots:

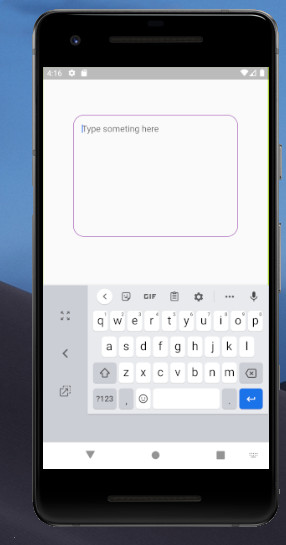
Single-line rounded TextField
Center(
child: Container(
width: 300,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20),
border: Border.all(
width: 1, color: Colors.purple, style: BorderStyle.solid)),
child: TextField(
decoration: const InputDecoration(
hintText: 'Type someting here',
contentPadding: EdgeInsets.all(15),
border: InputBorder.none),
onChanged: (value) {
// Do something
},
),
),
),
Multi-line rounded TextField
Center(
child: Container(
width: 300,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20),
border: Border.all(
width: 1, color: Colors.purple, style: BorderStyle.solid)),
child: TextField(
minLines: 10,
maxLines: 20,
decoration: const InputDecoration(
hintText: 'Type someting here',
contentPadding: EdgeInsets.all(15),
border: InputBorder.none),
onChanged: (value) {},
),
),
),
Afterword
You’ve learned more than one technique to implement rounded corners TextFields in Flutter. This knowledge will be helpful when you want to build more attractive user interfaces. If you’d like to explore more new and exciting things in Flutter, take a look at the following articles:
- Flutter: SliverGrid example
- Flutter TextField: Styling labelText, hintText, and errorText
- Create a Custom NumPad (Number Keyboard) in Flutter
- Flutter: GridPaper example
- Flutter: Creating Custom Back Buttons
- Using Provider for State Management in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.