This short and concise article shows you how to style the label text, hint text, and error text of a TextField (or TextFormField) widget in Flutter.
Note: Everything in the following examples is the same if you replace TextField with TextFormField.
Table of Contents
labelText
You can customize the label text by configuring the labelStyle property of the InputDecoration class.
Example:
Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: const Padding(
padding: EdgeInsets.all(30.0),
child: Center(
child: TextField(
decoration: InputDecoration(
labelText: 'label text',
labelStyle: TextStyle(
color: Colors.red,
fontSize: 22,
fontStyle: FontStyle.italic,
decoration: TextDecoration.underline)),
),
),
),
);
Output:
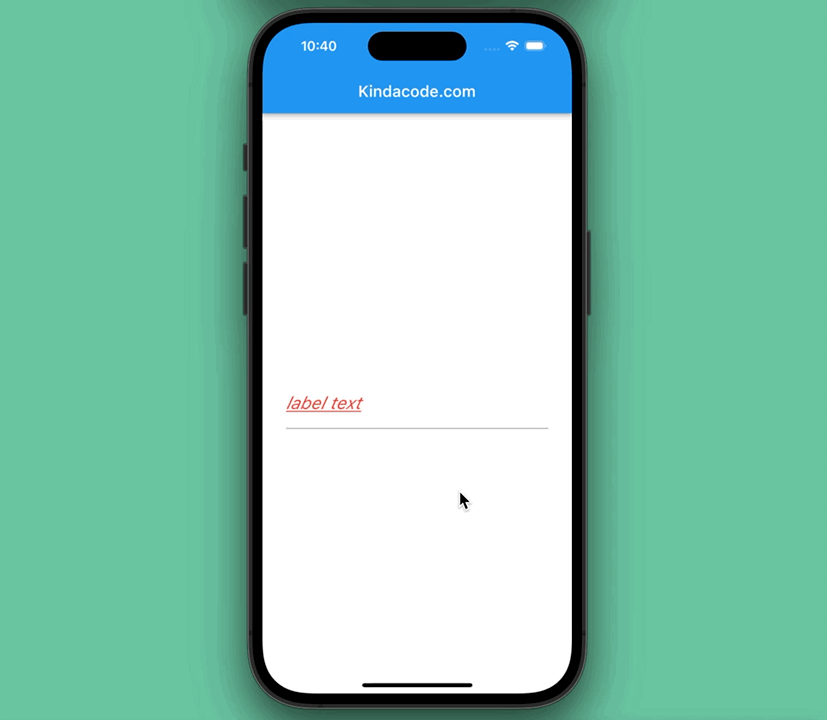
hintText
In order to style the hint text, you can manipulate the hintStyle property of the InputDecoration class.
Example:
Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: const Padding(
padding: EdgeInsets.all(30.0),
child: Center(
child: TextField(
decoration: InputDecoration(
hintText: 'hint text',
hintStyle: TextStyle(
color: Colors.purple,
fontSize: 24,
fontStyle: FontStyle.italic,
decoration: TextDecoration.underline)),
),
),
),
);
Output:
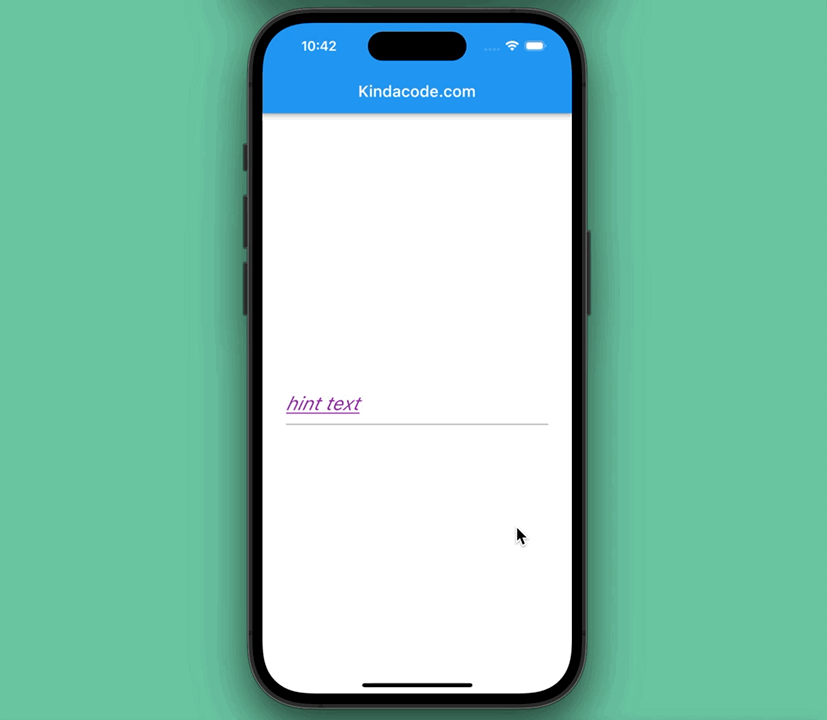
errorText
The error text of a text field can be styled by setting the errorStyle property of the InputDecoration class. In addition, you can control the max lines of the error text with the errorMaxLines argument.
Example:
TextField(
decoration: InputDecoration(
errorText: 'Something went wrong. Please re-check your input',
errorMaxLines: 3,
errorStyle: TextStyle(
color: Colors.red,
fontSize: 24,
fontWeight: FontWeight.bold,
fontStyle: FontStyle.italic,
decoration: TextDecoration.lineThrough
)
),
),
Output:
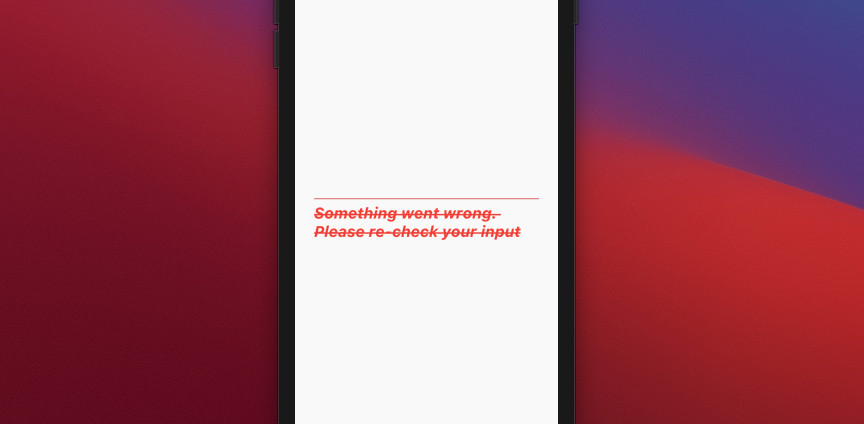
Conclusion
We’ve walked through a few examples of styling label text, hint text, and error text of a TextField widget in Flutter. Continue learning more interesting things by taking a look at the following articles:
- How to set width, height, and padding of TextField in Flutter
- Flutter: Show/Hide Password in TextField/TextFormField
- Flutter: Creating an Auto-Resize TextField
- Working with dynamic Checkboxes in Flutter
- Flutter and Firestore Database: CRUD example
- Most Popular Packages for State Management in Flutter
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.