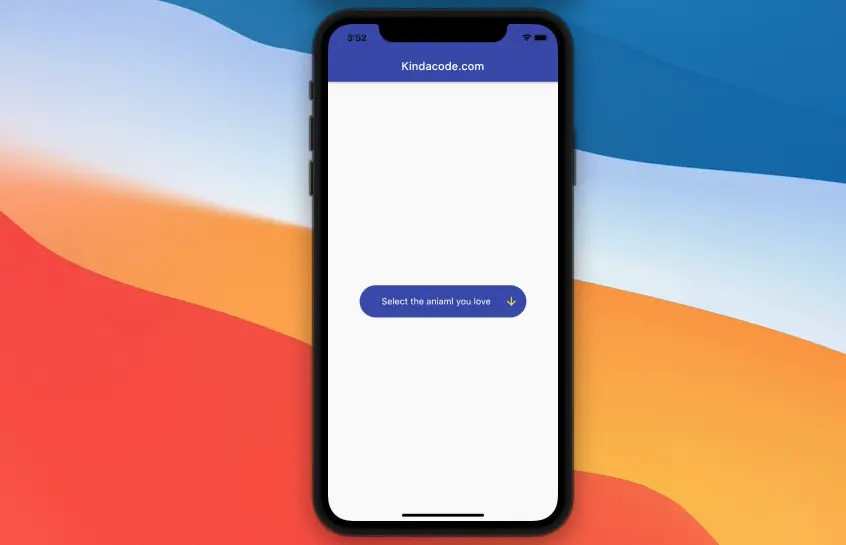
This article walks you through an end-to-end complete example of implementing the DropdownButton
widget in Flutter.
Overview
A Quick Note
When a DropdownButton
widget gets pressed, a menu with items will show up and let the user select one item from them. The selected item will be displayed on the button when the menu disappears.
The items of the DropdownButton
are implemented by using a list of DropdownMenuItem
widgets:
DropdownButton<T>(
value: T?,
items: List<DropdownMenuItem<T>>?,
)
The type of the value
and the type specialized for each DropdownMenuItem
must be the same.
Setting the Width for a Dropdown Button
You can set the width for a DropdownButton
by setting its isExpanded
parameter to true
and wrapping it inside a fixed-size Container
or SIzedBox
.
Hiding the underline
Below are two different ways to remove the underline of a DropdownButton
.
1. Set the underline
parameter to an empty Container
:
underline: Container()
2. Place the DropdownButton
widget inside a DropdownButtonHideUnderline
widget:
DropdownButtonHideUnderline(
child: DropdownButton(/* ... */),
)
Words might be boring and confusing. The example below will help you get a better understanding.
The Example
Preview
The demo app we are going to make contains a rounded dropdown button with white hint text. Its width is 300px and its underline is invisible.
The dropdown menu is amber and its text is dark. The selected item will have a custom text style, thanks to selectedItemBuilder
.
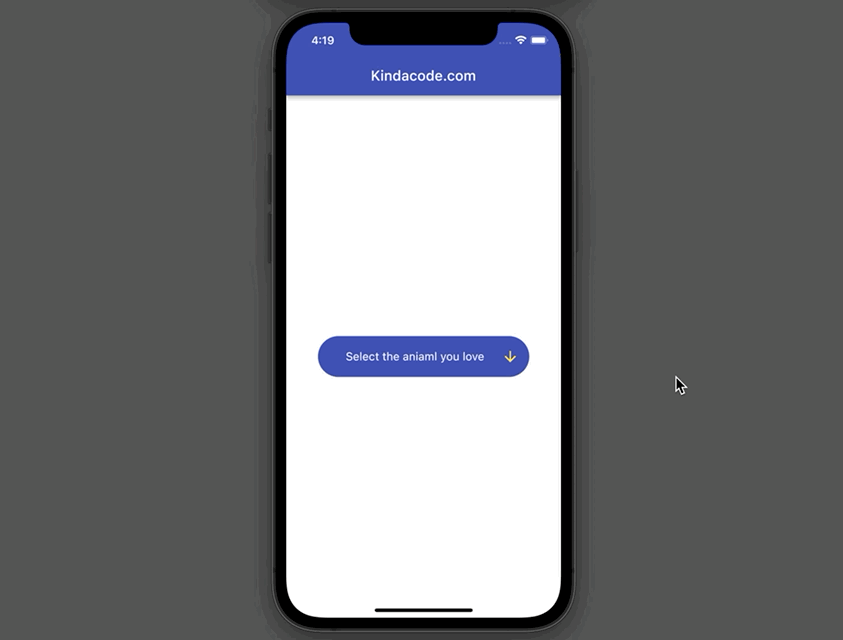
The Complete Code
The full source code in main.dart
with explanations:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const HomePage());
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// define a list of options for the dropdown
final List<String> _animals = ["Dog", "Cat", "Crocodile", "Dragon"];
// the selected value
String? _selectedAnimal;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: Container(
padding: const EdgeInsets.symmetric(vertical: 5, horizontal: 15),
width: 300,
decoration: BoxDecoration(
color: Theme.of(context).primaryColor,
borderRadius: BorderRadius.circular(30)),
child: DropdownButton<String>(
value: _selectedAnimal,
onChanged: (value) {
setState(() {
_selectedAnimal = value;
});
debugPrint("You selected $_selectedAnimal");
},
hint: const Center(
child: Text(
'Select the aniaml you love',
style: TextStyle(color: Colors.white),
)),
// Hide the default underline
underline: Container(),
// set the color of the dropdown menu
dropdownColor: Colors.amber,
icon: const Icon(
Icons.arrow_downward,
color: Colors.yellow,
),
isExpanded: true,
// The list of options
items: _animals
.map((e) => DropdownMenuItem(
value: e,
child: Container(
alignment: Alignment.centerLeft,
child: Text(
e,
style: const TextStyle(fontSize: 18),
),
),
))
.toList(),
// Customize the selected item
selectedItemBuilder: (BuildContext context) => _animals
.map((e) => Center(
child: Text(
e,
style: const TextStyle(
fontSize: 18,
color: Colors.amber,
fontStyle: FontStyle.italic,
fontWeight: FontWeight.bold),
),
))
.toList(),
),
),
),
);
}
}
Conclusion
We’ve explored the fundamentals of the DropdownButton
and DropdownMenuItem
widget in Flutter. If you’d like to learn more new and interesting things in Flutter and Dart, take a look at the following articles:
- 2 Ways to Create Flipping Card Animation in Flutter
- Flutter: Making a Dropdown Multiselect with Checkboxes
- Flutter: How to Make Spinning Animation without Plugins
- Using Cascade Notation in Dart and Flutter
- Sorting Lists in Dart and Flutter (5 Examples)
- Flutter: Add a Search Field to an App Bar (2 Approaches)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.