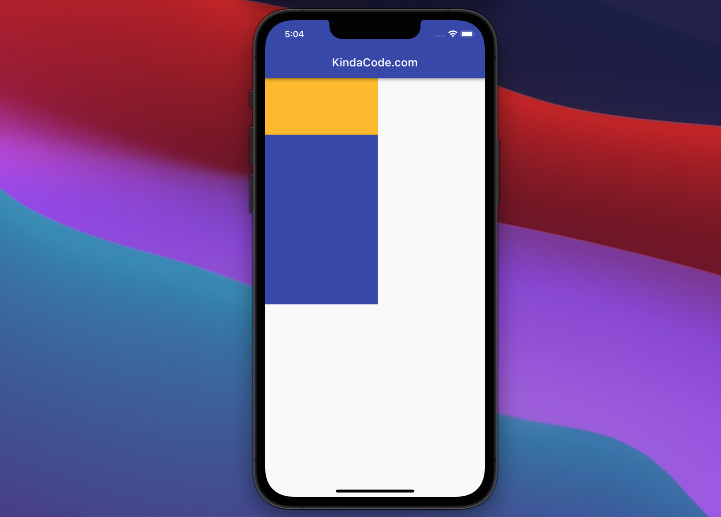
IntrinsicWidth is a built-in widget in Flutter that is used to size its child to the child’s maximum intrinsic width. The constraints that IntrinsicWidth passes to its child will adhere to the parent’s constraints.
Overview
Constructor:
IntrinsicWidth({
Key? key,
double? stepWidth,
double? stepHeight,
Widget? child
})
If stepWidth is null or 0.0 the child’s width will be the same as its maximum intrinsic width. Otherwise, stepWidth forces its child’s width to be a multiple of this value. For example, if stepWidth is 100 and the child’s maximum intrinsic width is 150, the child’s width can be 100 or 200.
If stepHeight is null or 0.0 then the child’s height will not be constrained. Otherwise, stepHeight sets the child’s height to be a multiple of this value. For example, if stepHeight is 150 and the child’s maximum intrinsic height is 200, the child’s height will be 150 or 300.
Example
Preview
The app in the screenshot on the left uses an IntrinsicWidth while the one on the right doesn’t. You can clearly see the difference:

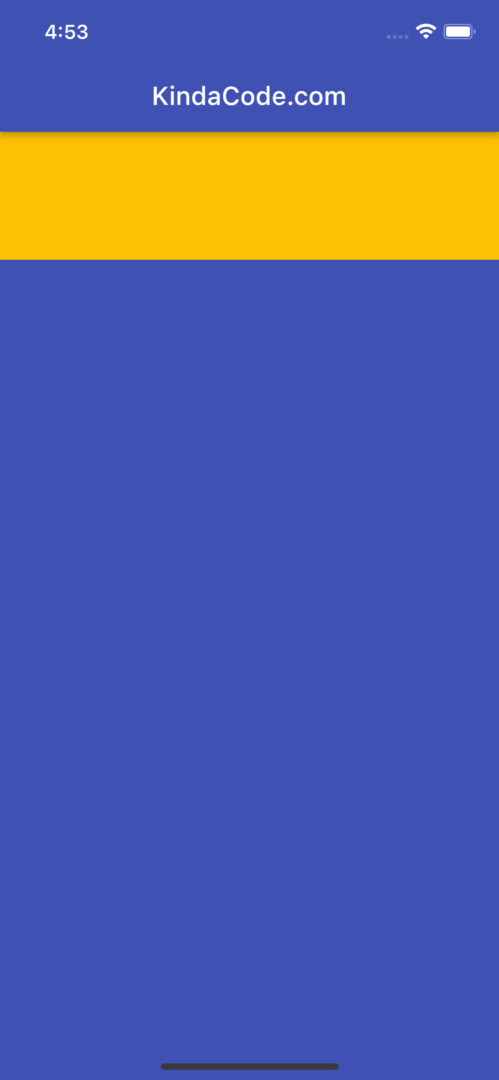
Code & Explanation
With IntrinsicWidth:
Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: IntrinsicWidth(
stepWidth: 100,
stepHeight: 400,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container(
width: 100,
height: 100,
color: Colors.amber,
),
Expanded(
child: Container(
color: Colors.indigo,
width: 200,
height: 300,
),
),
],
),
),
);
stepWidth is set to 100. In the first screenshot, we could see that the width of the amber box is 200 (200 = 100 x 2). The width of the indigo box is 200 (200 = 100 x 2). The sum of the heights of these two boxes is 400, equal to the stepHeight passed to the IntrinsicWidth widget.
Without IntrinsicWidth:
Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
Container(
width: 100,
height: 100,
color: Colors.amber,
),
Expanded(
child: Container(
color: Colors.indigo,
width: 200,
height: 300,
),
),
],
),
);
As you could see, the amber container expands to the maximum width of the screen even if its width is set to 100. Almost the same, the indigo box occupies all the remaining space.
Conclusion
You’ve learned how the IntrinsicWidth widget works and how you can implement it. This widget might be expensive in performance so you should avoid using it when not really necessary. If you’d like to explore more new and exciting stuff about Flutter, take a look at the following articles:
- Flutter: Adding a Gradient Border to a Container (2 Examples)
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
- Flutter: SizedOverflowBox example
- Flutter & SQLite: CRUD Example
- Using GetX (Get) for State Management in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.