This article walks you through 2 examples of adding a gradient border to a Container in Flutter.
The Solution
Even though Flutter doesn’t provide a direct way to implement gradient borders, we can do it ourselves with a few lines of code. The trick here is to place a Container inside a bigger Container that has a gradient color background. Let’s see how we apply this technique through the following examples.
Example: Border with Gradient
Preview
This example creates 2 boxes. The first one has a linear gradient border and the second one has a sweep gradient border:

The Code
Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Padding(
padding: const EdgeInsets.all(15),
child: Column(
children: [
// Linear Gradient Border
Container(
width: 220,
height: 120,
alignment: Alignment.center,
decoration: BoxDecoration(
gradient: LinearGradient(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
colors: [
Colors.purple.shade900,
Colors.purple.shade100,
])),
child: Container(
width: 200,
height: 100,
color: Colors.white,
alignment: Alignment.center,
child: const Text('Linear Gradient'),
),
),
const SizedBox(
height: 30,
),
// Sweep Gradient Border
Container(
width: 320,
height: 220,
alignment: Alignment.center,
decoration: const BoxDecoration(
gradient: SweepGradient(
colors: [
Colors.red,
Colors.yellow,
Colors.orange,
Colors.redAccent
],
tileMode: TileMode.mirror,
)),
child: Container(
width: 300,
height: 200,
color: Colors.amber,
alignment: Alignment.center,
child: const Text('Sweep Gradient'),
),
),
],
),
),
);
Example: Border with Gradient and Radius (Rounded Corners)
Preview
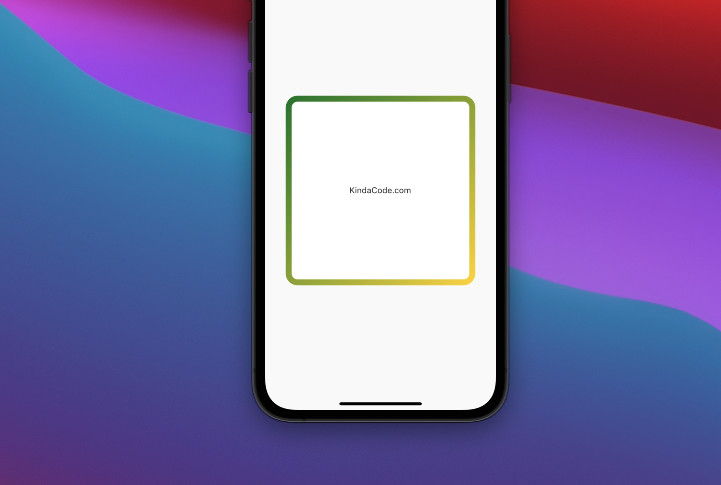
The Code
Container(
width: 320,
height: 320,
alignment: Alignment.center,
decoration: BoxDecoration(
borderRadius: const BorderRadius.all(Radius.circular(20)),
gradient: LinearGradient(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
colors: [
Colors.green.shade800,
Colors.yellow.shade600,
])),
child: Container(
width: 300,
height: 300,
decoration: const BoxDecoration(
borderRadius: BorderRadius.all(Radius.circular(10)),
color: Colors.white,
),
alignment: Alignment.center,
child: const Text('KindaCode.com'),
),
),
Conclusion
You’ve learned a simple technique to implement gradient borders in Flutter. This knowledge will be very helpful for you in building more attractive and engaging user interfaces. If you’d like to explore more about the gradient stuff and other awesome things in the realm of Flutter, take a look at the following articles:
- Flutter Gradient Text Examples
- Flutter: 2 Ways to Create Gradient Background Buttons
- Flutter: Set gradient background color for the entire screen
- 2 Ways to Create Flipping Card Animation in Flutter
- Flutter DecoratedBoxTransition example
- Hero Widget in Flutter: A Practical Guide
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.