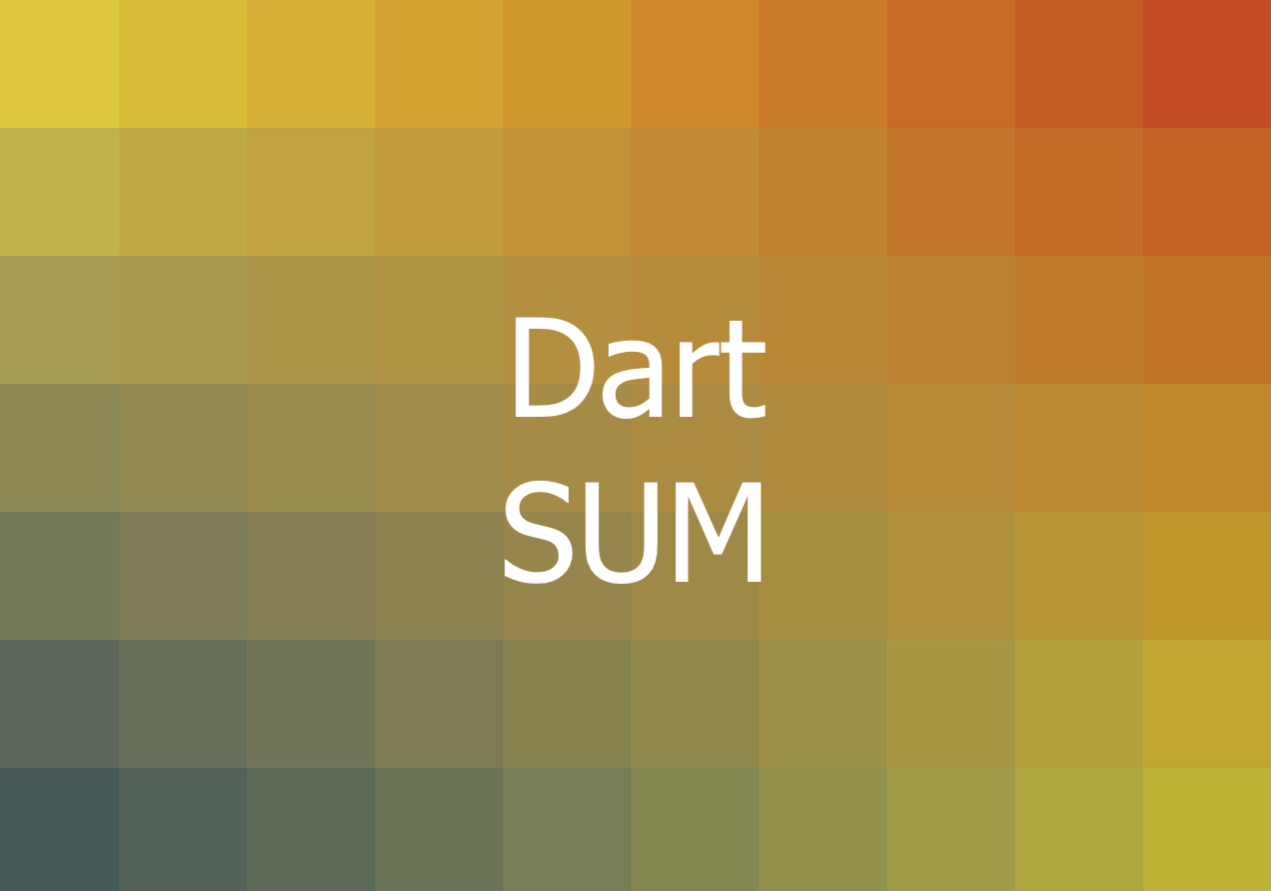
This practical, example-centric article walks you through 5 different approaches to calculating the sum of a list of numbers in Dart (and Flutter as well). No need to delay, let’s start right away and write some code.
Using fold() method
Example:
// kindacode.com
void main() {
List<double> numbers = [1, 2, 3, 4, 5, 0.5, -1.5, 0.25, -0.25];
double sum = numbers.fold(0, (a, b) => a + b);
print(sum);
}
Output:
14.0
The fold() method (of the List class) is a higher-order function that applies a given function to each element in a collection and accumulates the results. In the example above, we pass an anonymous function that simply adds the two arguments (the accumulator and the current value) together.
Using a For loop
For loop is a popular flow control that has been around for a long time in most programming languages, including Dart. This is also a powerful tool that helps us calculate many things, including finding the sum of the elements in a given list.
Example:
// kindacode.com
void main() {
final myListofNumbers = [1, 2, 3, 4, 5, 6.4, 7.1, -4, -1.5];
var sum = 0.0;
for (var i = 0; i < myListofNumbers.length; i++) {
sum += myListofNumbers[i];
}
print(sum);
}
Output:
23.0
In this approach (which is a little bit longer than other approaches mentioned in this article), we use a for loop to iterate over the list of numbers (both integers and doubles) and add each element to a variable named sum.
Using the reduce() method
Example:
// kindacode.com
void main() {
final numbers = [1, 2, 3, 4.4, -1.2, 5, 6, 7, 8, 9];
var sum = numbers.reduce((a, b) => a + b);
print(sum);
}
Output:
44.2
The reduce() method is similar to the fold() method, but it doesn’t take an initial value as its first argument. Instead, it uses the first element in the collection as the initial value and applies the given function to the remaining elements. In this case, we pass the same function as in the fold() example.
Using the sum getter from the collection package
Example:
// kindacode.com
import 'package:collection/collection.dart';
void main() {
final numbers = [100, 50, 0, 25, 1, 90.8];
var sum = numbers.sum;
print(sum);
}
Output:
266.8
At the first glance, this may seem the shortest approach. But in fact, we need to add this line:
'package:collection/collection.dart';
So, some developers prefer using other approaches to this one.
Using a for-each loop
Example:
// kindacode.com
void main() {
final numbers = [5, 10, 15, 20, 25];
var sum = 0;
numbers.forEach((number) {
sum += number;
});
print(sum);
}
Output:
75
In this approach, we use a for-each loop to iterate over the list and add each element to the sum variable.
Conclusion
We’ve explored 5 different ways to find the sum of numeric elements in a given list in Dart. Which one do you like the most? Please share your thoughts by leaving comments.
If you are not tired and want to learn more new things about Flutter and Dart, take a look at the following articles:
- Sorting Lists in Dart and Flutter (5 Examples)
- Dart: Calculating Variance and Standard Deviation
- How to Create a Sortable ListView in Flutter
- Flutter: 2 Ways to Create an Onboarding Flow (Intro Slider)
- 2 Ways to Create Typewriter Effects in Flutter
- 2 Ways to Get a Random Item from a List in Dart (and Flutter)
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.