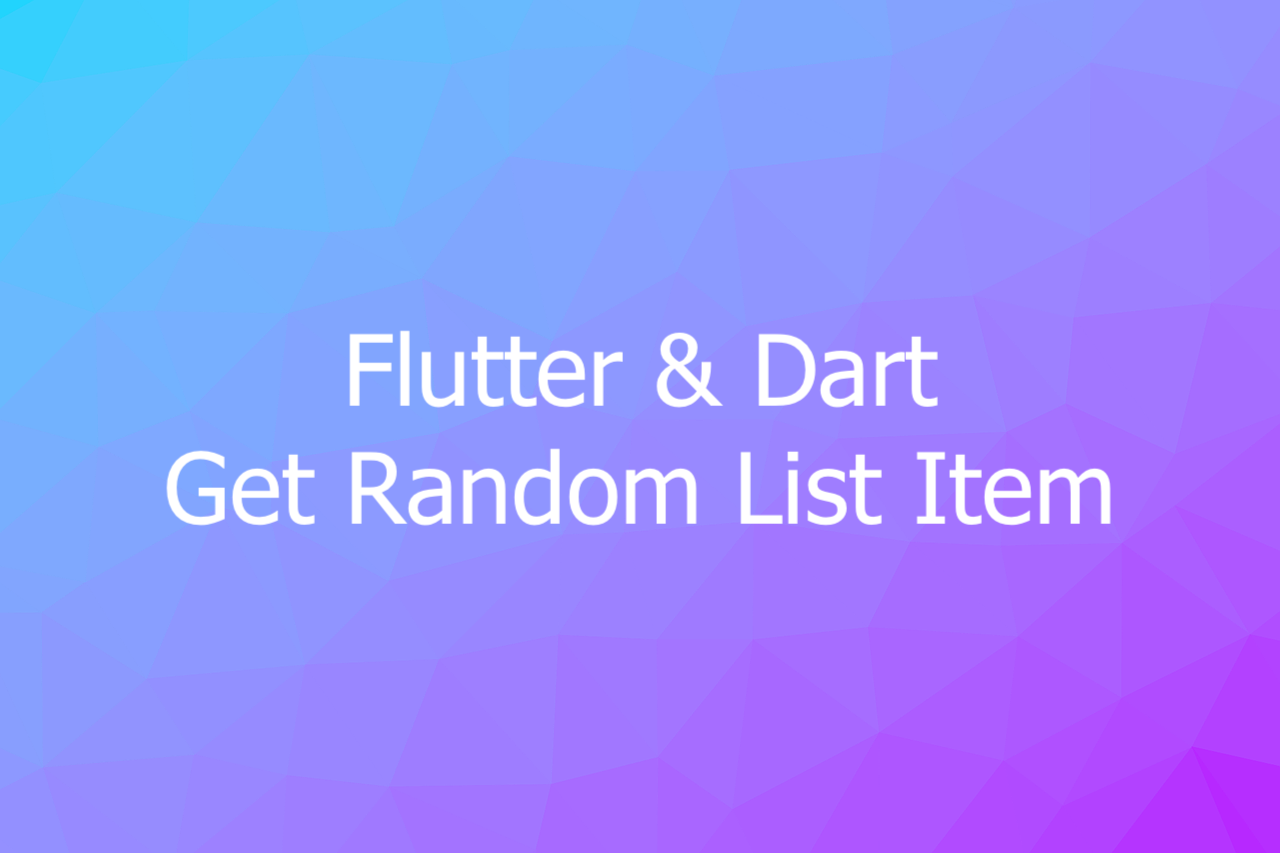
When developing apps with Flutter and Dart, there might be cases where you want to retrieve a random item from a given list (for example, an online shop wants to give a random gift to their customer; or a teacher wants to choose a random question to test students). There is no built-in function or method specifically designed for this task. However, we can easily solve it in more than one way with a few lines of code.
Method #1
In this method, we will randomly change the position of the items in the list by using the shuffle() method, then get the first one.
Example:
// kindacode.com
void main() {
List<String> colors = ['red', 'green', 'blue', 'yellow', 'orange'];
colors.shuffle();
var randomColor = colors[0];
print(randomColor);
}
Method #2
Here’s what we’ll do:
- Use Random.nextInt() to generate a random index number (in the range from 0 to list.length – 1)
- Use the random index to retrieve the corresponding list element
Example:
// kindacode.com
import 'dart:math';
void main() {
var choices = ['a', 'b', 'c', 'd', 'e'];
int randomIndex = Random().nextInt(choices.length);
var randomChoice = choices[randomIndex];
print(randomChoice);
}
The output can be ‘a’, ‘b’, ‘c’, ‘d’, or ‘e’.
You can explore more interesting stuff related to the randomness in Flutter through the following articles:
- Flutter & Dart: 3 Ways to Generate Random Strings
- 3 Ways to create Random Colors in Flutter
- Flutter/Dart: Generate Random Numbers between Min and Max
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.