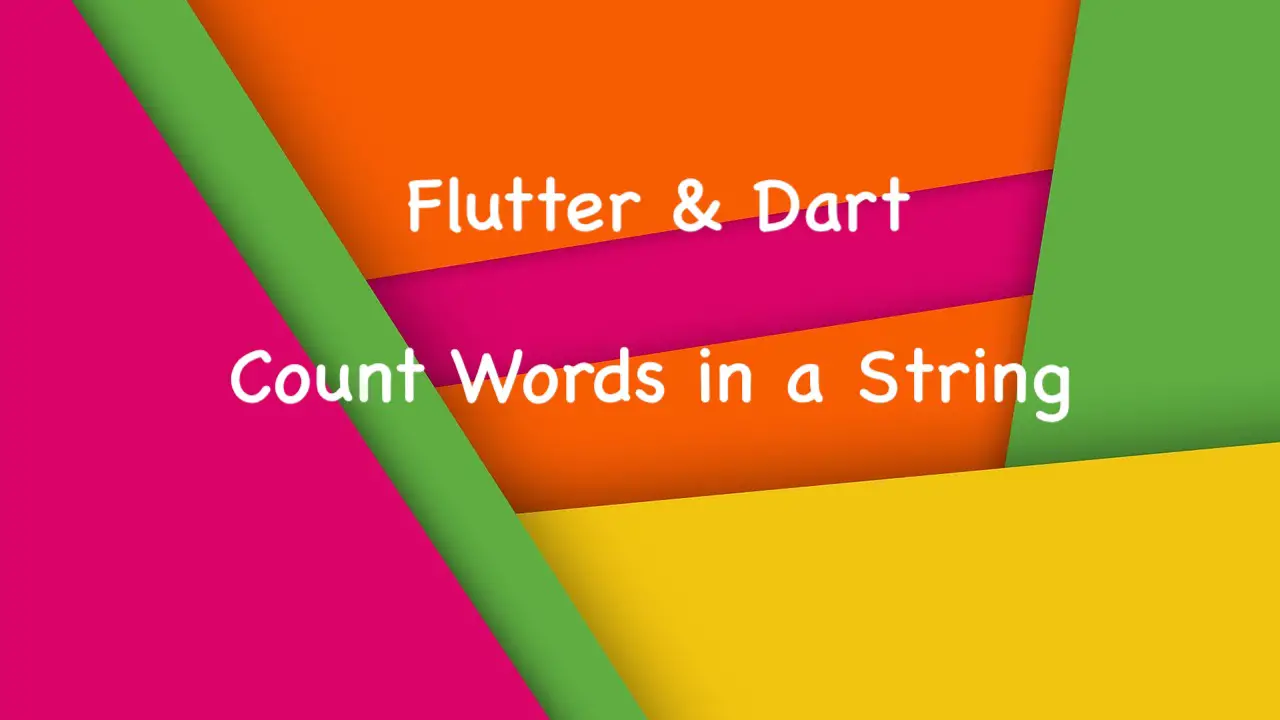
This short post shows you 2 ways to count the number of words in a given string in Dart (and Flutter as well).
Using the split() method
We can use the split()
method to return the list of the substrings between the white spaces in the given string.
Example:
// KindaCode.com
import 'package:flutter/foundation.dart';
void main() {
const String s = 'Kindacode.com is a website about programming.';
final List l = s.split(' ');
if (kDebugMode) {
print(l.length);
}
}
Output:
6
Using Regular Expressions
Using a regular expression is a little bit more complicated but it gives you more flexibility.
Example:
// KindaCode.com
import 'package:flutter/foundation.dart';
void main() {
const String s =
'Kindacode.com is a website about programming. Under_score en-dash em-dash and some special words.';
final RegExp regExp = RegExp(r"[\w-._]+");
final Iterable matches = regExp.allMatches(s);
final int count = matches.length;
if (kDebugMode) {
print(count);
}
}
Output:
13
Continue exploring more about Flutter and Dart:
- Displaying Subscripts and Superscripts in Flutter
- Flutter & Dart: Regular Expression Examples
- Sorting Lists in Dart and Flutter (5 Examples)
- Dart: Convert Map to Query String and vice versa
- Dart: Capitalize the First Letter of Each Word in a String
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.