This is a short guide to default parameter values for a function in Flutter (and Dart as well).
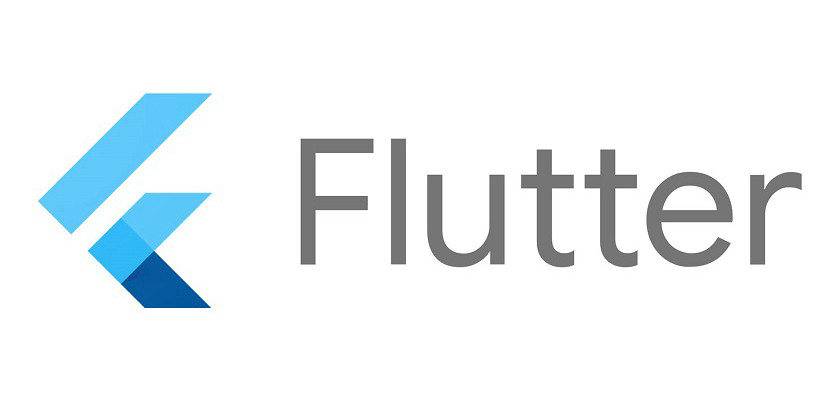
Default function parameters allow formal parameters to be initialized with default values if no value is passed.
Syntax:
functionName({
param1 = defaultValue1,
param2 = defaultValue2,
...
paramN = defaultValueN
}){
/* */
}
If you forget the curly brackets, you’ll see the following error message:
Error: Non-optional parameters can't have a default value.
Try removing the default value or making the parameter optional.
Example
The code:
// kindacode.com
// main.dart
import "package:flutter/foundation.dart";
void productInfo({
String name = 'Unknown',
double price = 0.00,
String color = 'Blue',
String description = 'This is a fictional product',
}) {
if (kDebugMode) {
print('Product Name: $name');
print('Product price: $price');
print('Product color: $color');
print('Introduction: $description');
}
}
void main() {
// Only provide name
productInfo(name: 'KindaCode.com');
// Only provide name and color
productInfo(name: 'A Novel', color: 'Amber');
// All params are passed
productInfo(
name: 'A Phone',
price: 99.99,
color: 'Silver',
description: 'A strong smartphone');
}
Output:
Product Name: KindaCode.com
Product price: 0.0
Product color: Blue
Introduction: This is a fictional product
Product Name: A Novel
Product price: 0.0
Product color: Amber
Introduction: This is a fictional product
Product Name: A Phone
Product price: 99.99
Product color: Silver
Introduction: A strong smartphone
That’s it. Happy coding, and have a nice day. If you’d like to learn more about Dart and Flutter, take a look at the following articles:
- Dart: Capitalize the First Letter of Each Word in a String
- Dart: Calculate the Sum of all Values in a Map
- Dart regex to validate US/CA phone numbers
- How to Iterate through a Map in Flutter & Dart
- Using GetX (Get) for Navigation and Routing in Flutter
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
You can also take a tour around our Flutter topic page or Dart topic page for the latest tutorials and examples.