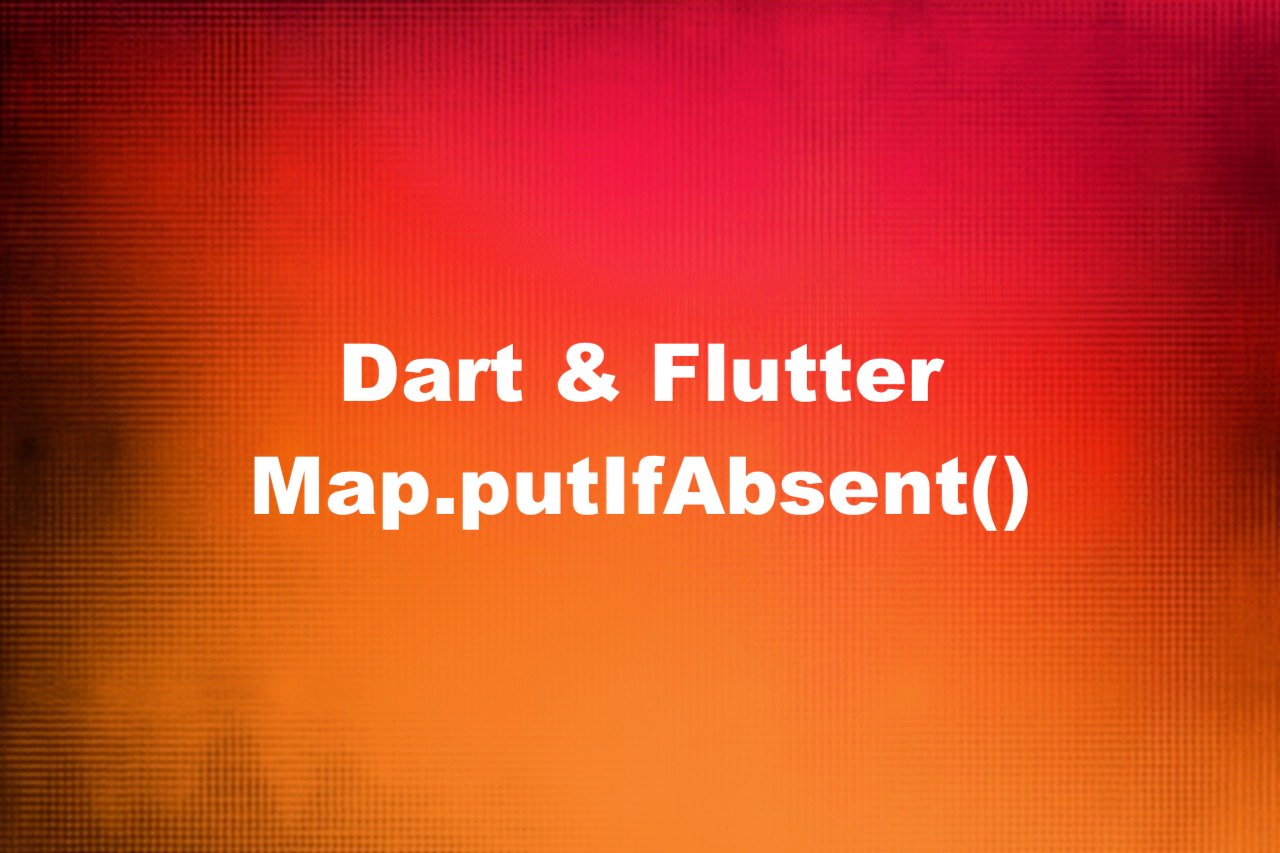
This concise article is about the Map.putIfAbsent()
method in the Dart programming language.
The Fundamentals
The purpose of the Map.putIfAbsent()
method is to look up the value of a key in a map or add a new entry if it isn’t there. This is useful when you want to initialize a map entry lazily, that is, only when it is needed. For instance, you can use this method to create a map that counts the occurrences of each word in a list of words, without having to check if the word already exists in the map or not.
Syntax:
V putIfAbsent(K key, V ifAbsent())
Where:
key
: The key to look up or add in the map.ifAbsent
: A function that returns the value to be associated with the key if it is not present in the map.
The return value is the value associated with the key, either the existing one or the new one returned by ifAbsent
.
Examples
Below are three practical examples that demonstrate how useful the Map.putIfAbsent()
method is. They are arranged in order from basic to advanced, from simple to complex.
Basic usage
In this example, we’ll create a simple map and use putIfAbsent()
to add a new key-value pair if the key doesn’t already exist in the map.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
var map = {'key1': 'value1', 'key2': 'value2'};
if (kDebugMode) {
print(map); // Outputs: {key1: value1, key2: value2}
}
String updateValue = map.putIfAbsent('key3', () => 'value3');
if (kDebugMode) {
print(map); // Outputs: {key1: value1, key2: value2, key3: value3}
print(updateValue); // Outputs: value3
}
}
Counting words
In this example, we use the Map.putIfAbsent() method to create a map that counts the occurrences of each word in a list of words. We iterate over the list and use the word as the key and a function that returns 1 as the ifAbsent
parameter. If the word already exists in the map, we increment its value by 1. Otherwise, we add a new entry with the value 1.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
// A list of words
var words = [
'wolf',
'dinosaurs',
'kindacode.com',
'wolf',
'wolf',
'wolf',
'kindacode.com'
];
// A map to store the word counts
var wordCounts = <String, int>{};
// Iterate over the words
for (var word in words) {
// Look up or add the word in the map
wordCounts.putIfAbsent(word, () => 1);
// Increment the word count by 1
wordCounts[word] = wordCounts[word]! + 1;
}
// Print the word counts
if (kDebugMode) {
print(wordCounts);
}
}
Output:
{wolf: 5, dinosaurs: 2, kindacode.com: 3}
Caching data
This example uses the Map.putIfAbsent()
method to create a cache that stores the results of some expensive computation. We’ll define a function that takes an input and returns a result. We’ll also define a map that acts as a cache. Whenever we need to compute a result for an input, we first check if it is already in the cache. If not, we use the Map.putIfAbsent()
method to add a new entry with the input as the key and a function that calls the computation as the ifAbsent
parameter. This way, we avoid repeating the computation for the same input.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
void main() {
// A function that performs some expensive computation
int compute(int input) {
debugPrint('Computing for $input...');
// Some dummy computation
return input * input + input;
}
// A map to store the cached results
var cache = <int, int>{};
// A function that returns the result for an input, using cache if possible
int getResult(int input) {
// Check if the result is already in cache
if (cache.containsKey(input)) {
debugPrint('Using cached result for $input...');
return cache[input]!;
}
// Otherwise, use putIfAbsent to add a new entry in cache
debugPrint('Adding new result for $input...');
return cache.putIfAbsent(input, () => compute(input));
}
// Test the getResult function
debugPrint(getResult(10)
.toString()); // Adding new result for 10... Computing for 10... 110
debugPrint(getResult(20)
.toString()); // Adding new result for 20... Computing for 20... 420
debugPrint(getResult(10).toString()); // Using cached result for 10... 110
debugPrint(getResult(30)
.toString()); // Adding new result for 30... Computing for 30... 930
}
Afterword
This article covered everything you need to know about the Map.putIfAbsent()
method. It indeed is a useful and convenient method that can simplify some common tasks involving maps. It can help you avoid unnecessary checks and reduce code duplication when you want to look up or add a key-value pair in a map. It can also help you to improve performance by lazily initializing the values only when they are needed.
If you’d like to explore more cool and interesting stuff in Dart and Flutter, take a look at the following articles:
- Using Dio to fetch data in Flutter (with example)
- Dart: Remove Consecutive White Spaces from a String (3 Ways)
- Flutter & Dart: Convert a String into a List and vice versa
- Flutter: Get the Width & Height of a Network Image
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
- Flutter: Make a simple Color Picker from scratch
- Flutter: Caching Network Images for Big Performance gains
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.