
This succinct, straight-to-the-point article will walk you through a couple of different ways to turn an object (class instance) to JSON in Dart (and Flutter as well). Let’s begin!
Using self-written code
This approach involves manually writing functions to convert your Dart object to and from JSON. This is a straightforward solution where you have full control over the serialization process.
The steps are:
- Define your class.
- Create a method inside the class to convert the object into a
Map
. - Convert the
Map
object to a JSON string using thejsonEncode()
function from thedart:convert
library.
Code example:
// KindaCode.com
// main.dart
import 'dart:convert';
import 'package:flutter/foundation.dart' show debugPrint;
// Define a class
class User {
final String name;
final int age;
final bool isMarried;
User(this.name, this.age, this.isMarried);
// Define a toJson method to convert User object to JSON
Map<String, dynamic> toJson() {
return {
'name': name,
'age': age,
"isMarried": isMarried,
};
}
}
void main() {
User user = User('Lone Wolf', 225, true);
// Convert User object to JSON
String jsonString = jsonEncode(user.toJson());
debugPrint(jsonString);
}
Output:
{"name":"Lone Wolf","age":225,"isMarried":true}
This technique is flexible and does not require any external dependencies or packages. The tradeoff is that you have to write some extra code and the logic may vary on different use cases. As the complexity of your objects increases, the manual implementation can become error-prone and inefficient.
Using json_serializable package
This solution uses the json_serializable
package that provides code generation for JSON serialization and deserialization. In the following example, we’ll define a User
class (which is more complicated than the one you’ve seen in the preceding example). This time, we’ll put the class in a separate file named user.dart
.
The steps to follow are:
1. Install the json_annotation
, json_serializable
, and build_runner
packages by executing this command:
flutter pub add json_annotation json_serializable build_runner
Then run this one:
flutter pub get
2. In the lib
directory of your Flutter project, add a new file called user.dart
. Define the User
class with the @JsonSerializable()
annotation like so:
// lib/user.dart
import 'package:json_annotation/json_annotation.dart';
part 'user.g.dart';
@JsonSerializable()
class User {
String name;
int age;
// Use JsonKey to change the name of the field in JSON
@JsonKey(name: 'is_admin')
bool isAdmin;
User(this.name, this.age, this.isAdmin);
// The generated toJson method
Map<String, dynamic> toJson() => _$UserToJson(this);
// The generated fromJson method
factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json);
}
3. Build the JSON serialization code with build_runner
by performing the following command:
dart run build_runner build
A new file named user.g.dart
will be automatically generated in your lib
folder:
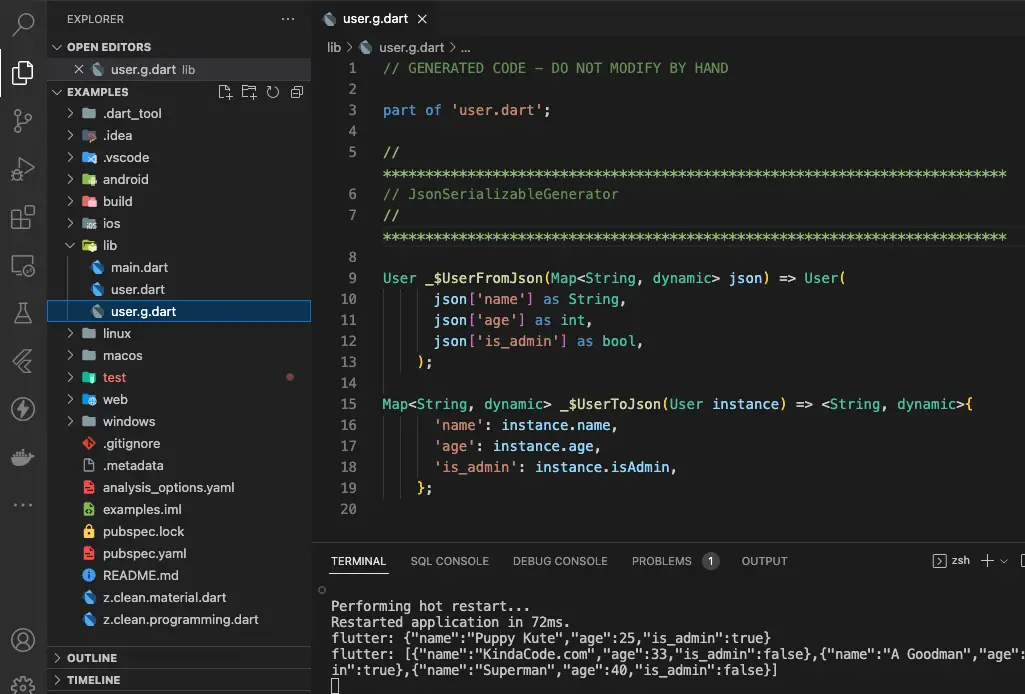
Here’s its content (you don’t have to understand the code in this file at all):
// GENERATED CODE - DO NOT MODIFY BY HAND
part of 'user.dart';
// **************************************************************************
// JsonSerializableGenerator
// **************************************************************************
User _$UserFromJson(Map<String, dynamic> json) => User(
json['name'] as String,
json['age'] as int,
json['is_admin'] as bool,
);
Map<String, dynamic> _$UserToJson(User instance) => <String, dynamic>{
'name': instance.name,
'age': instance.age,
'is_admin': instance.isAdmin,
};
4. Use the generated toJson()
method to convert the object or a list of objects to a JSON string. You can also use the generated fromJson()
method to convert a JSON string back to an object or a list of objects.
// lib/main.dart
import 'dart:convert' show jsonEncode;
import 'package:flutter/foundation.dart' show kDebugMode;
import 'user.dart';
void main() {
// Create a user object
User user = User('Puppy Kute', 25, true);
// Convert the user object to JSON
Map<String, dynamic> jsonUser = user.toJson();
// Print the JSON string
if (kDebugMode) {
print(jsonEncode(jsonUser));
// Prints: {"name":"Puppy Kute","age":25,"is_admin":true}
}
// Create a list of user objects
List<User> users = [
User('KindaCode.com', 33, false),
User('A Goodman', 35, true),
User('Superman', 40, false),
];
// Convert the list of user objects to a JSON string
List<Map<String, dynamic>> jsonUsers =
users.map((user) => user.toJson()).toList();
// Print the JSON string
if (kDebugMode) {
print(jsonEncode(jsonUsers));
// Prints: [{"name":"KindaCode.com","age":33,"is_admin":false},{"name":"A Goodman","age":35,"is_admin":true},{"name":"Superman","age":40,"is_admin":false}]
}
}
This approach supports converting nested objects and complex types such as dates or enums to JSON strings with annotations. However, it requires adding a bunch of external dependencies and packages to your project. You’ll also have to run a command to generate the code for the toJson()
and fromJson()
methods every time you make changes to your classes.
Conclusion
We’ve examined two different ways to turn a class object into JSON. The first one is quick and works well for simple use cases. The second one is powerful and really shines with complex data structures.
If you’d like to learn more new and interesting things about Dart and Flutter, take a look at the following articles:
- Flutter & Dart: Sorting a List of Objects/Maps by Date
- Dart: Convert Class Instances (Objects) to Maps and Vice Versa
- Dart Map.putIfAbsent() method (explained with examples)
- Using Dio to fetch data in Flutter (with example)
- Flutter: Get the Width & Height of a Network Image
- Flutter TextField: Styling labelText, hintText, and errorText
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.