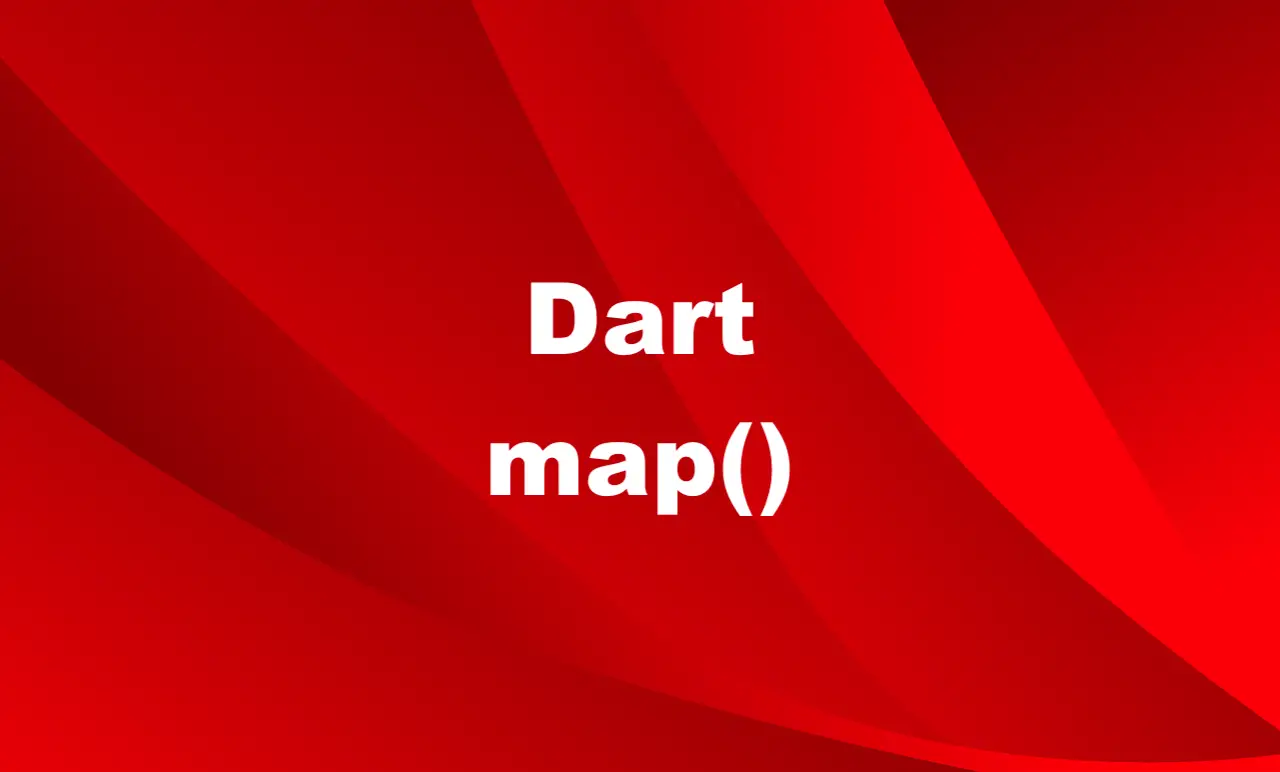
A Brief Overview
In Dart (and Flutter), the map()
method is used to transform each element of an iterable (such as a list, a set, or a map) into a new element according to a given function, and return a new iterable containing the transformed elements. The original iterable is not modified by the map()
method.
Syntax:
Iterable<T> map<T>(T f(E element))
Where T
is the type of the new elements, E
is the type of the original elements, and f
is the function that takes an element of type E
and returns an element of type T
. The map()
method returns an iterable of type Iterable<T>
.
Examples
Some practical examples that can help you understand the map()
method better.
Convert a list of numbers to a list of strings
Suppose we have a list of integers called numbers
, and we want to create a new list of strings called strings
, where each string is the number followed by a dot. For example, if numbers
is [1, 2, 3]
, then strings
should be ["1.", "2.", "3."]
. We can use the map()
method to achieve this by passing a function that appends a dot to each number as an argument.
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
// Define a list of numbers
List<int> numbers = [1, 2, 3];
// Define a function that appends a dot to each number
String addDot(int n) {
return "$n.";
}
// Use the map() method to create a new list of strings
List<String> strings = numbers.map(addDot).toList();
// Print the result
if (kDebugMode) {
print(strings);
}
}
Output:
[1., 2., 3.]
Using anonymous functions with the map() method
Sometimes, we may want to use a simple function that does not need a name or a separate definition. For example, if we want to double each element of a list of numbers, we can use an anonymous function that takes a number and returns its double. We can write anonymous functions using the arrow syntax (=>
) or the curly braces syntax ({}
).
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
// Define a list of numbers
List<int> numbers = [1, 2, 3];
// Use an anonymous function with arrow syntax to double each element
List<int> doubles = numbers.map((n) => n * 2).toList();
// Print the result
if (kDebugMode) {
print("Doubles: $doubles");
}
// Use an anonymous function with curly braces syntax to triple each element
List<int> triples = numbers.map((n) {
return n * 3;
}).toList();
// Print the result
if (kDebugMode) {
print("Triples: $triples");
}
}
Output:
Doubles: [2, 4, 6]
Triples: [3, 6, 9]
Using map() on maps
Maps are another type of iterable in Dart that stores data in the form of key-value pairs. Each pair is represented by an object called MapEntry
, which has two properties: key
and value
. We can use the map()
method on maps to transform both keys and values into new keys and values according to a given function. The function should take a pair of key and value as arguments and return another MapEntry
.
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
// Define a map of names and ages
Map<String, int> namesAndAges = {
"Foo": 50,
"Bar": 30,
"KindaCode.com": 90,
};
// Define a function that reverses the name and adds one to the age
MapEntry<String, int> reverseAndIncrement(String key, int value) {
// Reverse the name using split(), reversed, and join()
String reversedName = key.split("").reversed.join("");
// Add one to the age
int incrementedAge = value + 1;
// Return a new MapEntry with the reversed name and incremented age
return MapEntry(reversedName, incrementedAge);
}
// Use the map() method to create a new map with the transformed entries
Map<String, int> reversedAndIncremented =
namesAndAges.map(reverseAndIncrement);
// Print the result
if (kDebugMode) {
print(reversedAndIncremented);
}
}
Output:
{ooF: 51, raB: 31, moc.edoCadniK: 91}
Conclusion
The map()
method is a very useful and powerful feature of the Dart programming language. It allows us to apply a function to each element of an iterable and create a new iterable with the transformed elements. This can help us simplify our code and avoid writing loops or creating temporary variables.
If you’d like to explore more new and interesting things in Flutter and Dart, take a look at the following articles:
- Dart: How to Convert a List/Map into Bytes
- Flutter AnimatedList – Tutorial and Examples
- Flutter Slider: Tutorial & Example
- Flutter: SliverGrid example
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
- Using Dio to fetch data in Flutter (with example)
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.