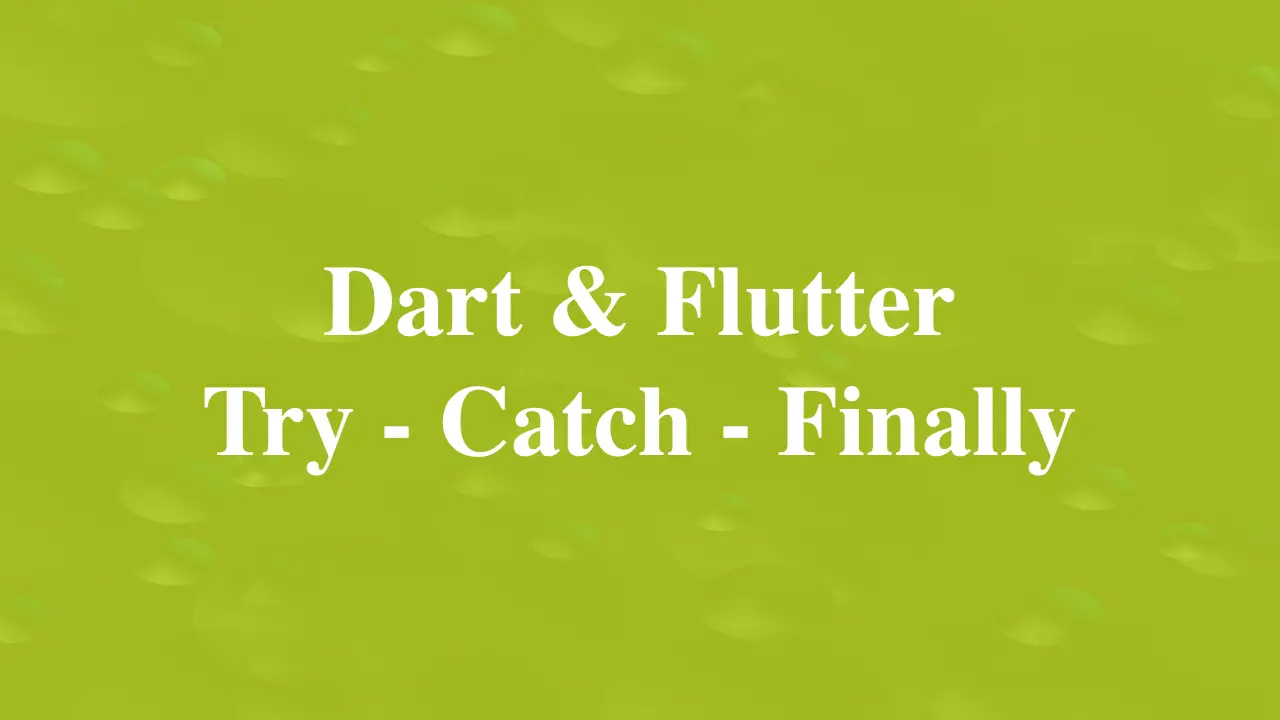
Overview
In Dart and many other programming languages, the purpose of the try/catch/finally
statement is to handle errors and exceptions that may occur during the execution of a program. Errors are problems that prevent the program from running correctly, such as syntax errors or type errors. Exceptions are problems that occur while the program is running, such as a file not found or division by zero. The try/catch/finally
syntax allows you to specify what actions to take when an error or an exception occurs, and what actions to take regardless of whether an error or an exception occurs.
In most cases, you’ll use the try/catch/finally
statement as follows:
try {
// Code that might throw an error or an exception
} on SpecificException catch (e) {
// Code that handles the specific exception
} catch (e) {
// Code that handles any other exception
} finally {
// Code that runs whether or not an exception is thrown
}
The parameters of the catch clause are optional, but if they are used, they must be in the order of (e)
and (e, s)
, where e
is the exception object and s
is the stack trace object. The value you receive after the try/catch/finally
block is the value calculated by the last executed statement in the block, or null
if no statement is executed.
Words can be ambiguous and hard to understand. Let’s write some actual code to see how things work in reality.
Examples
Basic error handling
This example shows how to use a simple try/catch block to handle a possible error when parsing a string into an integer.
// main.dart
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
String input =
'kindacode.com'; // A string that cannot be parsed into an integer
int number;
try {
number = int.parse(input); // This might throw a FormatException
if (kDebugMode) {
print('The number is $number.');
}
} catch (e) {
if (kDebugMode) {
print('The input is not a valid number: $e');
}
}
}
Output:
The input is not a valid number: FormatException: Invalid radix-10 number (at character 1)
kindacode.com
Handling specific exceptions
In this example, we’ll use the on
keyword to handle specific types of exceptions, such as FormatException
and ArgumentError
.
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
void main() {
String input = '-10'; // A string that can be parsed into an integer
int number;
try {
number = int.parse(input); // This might throw a FormatException
debugPrint('The number is $number.');
if (number < 0) {
throw ArgumentError(
'The number cannot be negative.'); // This might throw an ArgumentError
}
} on FormatException catch (e) {
debugPrint('The input is not a valid number: $e');
} on ArgumentError catch (e) {
debugPrint('The input is not acceptable: $e');
}
}
Output:
The number is -10.
The input is not acceptable: Invalid argument(s): The number cannot be negative.
Using the finally clause
This example demonstrates the way to use the finally
clause to perform some cleanup actions after a loop that might throw an exception. In this example, we use a List
object to store some numbers, and we use the finally
clause to print the product of the numbers.
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
void main() {
List<int> numbers = [1, 2, 3, 4, 5, 0, 6]; // A list of numbers
int product = 1; // A variable to store the product
try {
// Loop through the list of numbers
for (int i = 0; i < numbers.length; i++) {
// If the number is 0, throw an exception
if (numbers[i] == 0) {
throw Exception('The number cannot be zero.');
}
// Multiply the number with the current product
product *= numbers[i];
}
} catch (e) {
// Handle any errors or exceptions
debugPrint('An error occurred while looping through the list: $e');
} finally {
// Print the product of the numbers in the finally block
debugPrint(
'The product of the numbers before the exception occurred (if any) is: $product');
}
}
Output:
An error occurred while looping through the list: Exception: The number cannot be zero.
The product of the numbers before the exception occurred (if any) is: 120
Conclusion
In order to become a true Flutter developer, there’s no way to avoid learning try/catch/finally
. I hope this article is helpful for you. If this isn’t the case, please let me know by leaving a comment so that I can improve it.
If you’d like to explore more new and interesting stuff about Flutter and Dart, take a look at the following articles:
- Ways to iterate through a list in Dart and Flutter
- Flutter Stream.periodic: Tutorial & Example
- Flutter Web: How to Pick and Display an Image
- How to Get Device ID in Flutter (2 approaches)
- Flutter AnimatedList – Tutorial and Examples
- Dart & Flutter: Convert String/Number to Byte Array (Byte List)
- Flutter: How to put multiple ListViews inside a Column
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.