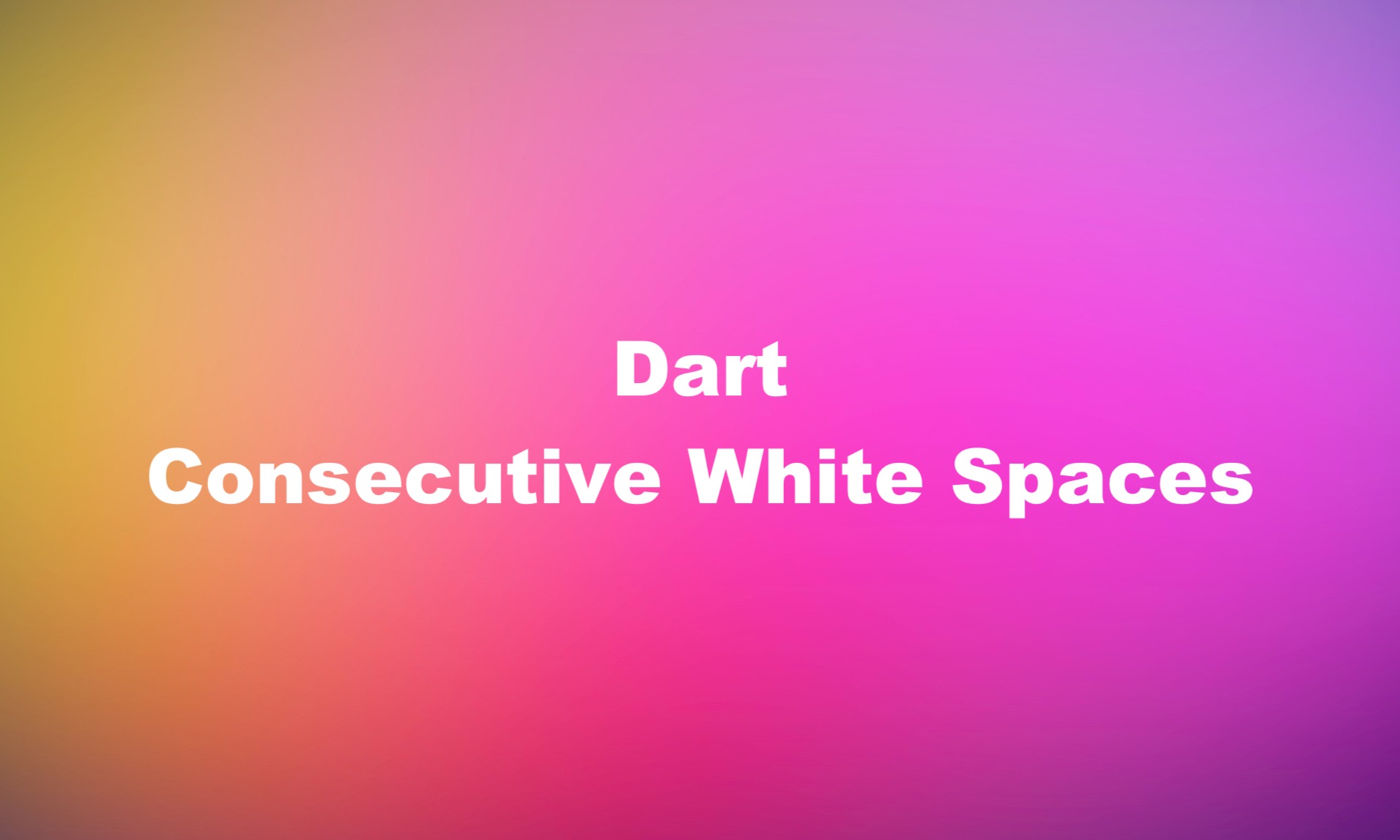
Consecutive white spaces in a string are multiple continuous spaces, tabs, or other whitespace characters appearing one after the other without any non-whitespace characters in between. In the vast majority of cases, you’ll only need a single white space between two words. This concise, example-based article will walk you through several different ways to replace multiple consecutive white spaces with a single white space. Let’s begin!
Using regular expressions
This approach uses a regular expression (RegExp
) object to match all the white space characters in the string and then replaces them with a single space using the replaceAll()
method of the String
class. The steps are:
- Create a RegExp object with the pattern
r"\s+"
, which matches one or more white space characters. - Call the replaceAll method on the string and pass the RegExp object and a single space as arguments.
- Return or print the resulting string.
Example:
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
// Define a function that removes multiple spaces from a string
String removeMultipleSpaces(String input) {
// Create a RegExp object with the pattern r"\s+"
RegExp whitespaceRE = RegExp(r"\s+");
// Replace all the white space characters with a single space
String output = input.replaceAll(whitespaceRE, " ");
// Return the resulting string
return output;
}
void main() {
String input = "Hi buddy. Welcome to KindaCode.com.";
String output = removeMultipleSpaces(input);
debugPrint(output);
}
Output:
Hi buddy. Welcome to KindaCode.com.
This solution is simple, concise, and easy to understand. It works for any kind of white space character, such as spaces, tabs, or newlines.
Using split() and join()
In this approach, we’ll use the split()
method of the String
class to split the input string into a list of substrings based on the white space characters, and then use the join()
method to join them back into a single string with a single space as the separator.
Example:
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
// Define a function that removes multiple spaces from a string
String removeMultipleSpaces(String input) {
// Split the input string into a list of substrings
List<String> substrings = input.split(" ");
// Remove empty substrings from the list
substrings = substrings.where((substring) => substring.isNotEmpty).toList();
// Join the substrings back into a single string with a single space as the separator
String output = substrings.join(" ");
// Return the resulting string
return output;
}
void main() {
String input =
"This is a test. KindaCode.com is a nice place to learn Flutter.";
String output = removeMultipleSpaces(input);
debugPrint(output);
}
Output:
This is a test. KindaCode.com is a nice place to learn Flutter.
This technique is a little bit more verbose than the previous one, but it doesn’t require you to define a regular expression pattern (which can be hard for newcomers).
Using StringBuffer and for loop
The main idea here is to use a StringBuffer
object to create a mutable string buffer, and then use a for
loop to iterate over each character in the input string and append it to the buffer if it is not a white space character or if it is not preceded by another white space character. Finally, convert the buffer into a string using the toString()
method.
Code example:
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
// Define a function that removes multiple spaces from a string
String removeMultipleSpaces(String input) {
// Create a StringBuffer object with an empty initial content
StringBuffer buffer = StringBuffer();
// Create a boolean variable to keep track of whether the previous character was a white space character or not
bool prevIsSpace = false;
// Use a for loop to iterate over each character in the input string
for (int i = 0; i < input.length; i++) {
// Get the current character
String char = input[i];
// If the current character is not a white space character
if (char != " ") {
// Append it to the buffer and set the boolean variable to false
buffer.write(char);
prevIsSpace = false;
}
// If the current character is a white space character and the boolean variable is false
else if (!prevIsSpace) {
// Append it to the buffer and set the boolean variable to true
buffer.write(char);
prevIsSpace = true;
}
// If the current character is a white space character and the boolean variable is true, do nothing
}
// Convert the buffer into a string
String output = buffer.toString();
// Return the resulting string
return output;
}
void main() {
String input =
"This is a test. KindaCode.com is a nice place to learn Flutter.";
String output = removeMultipleSpaces(input);
debugPrint(output);
}
Output:
This is a test. KindaCode.com is a nice place to learn Flutter.
This solution is much longer and more complicated the the two previous ones. However, with very big strings and a very large amount of text data, it can be more efficient since it does not create any new list or string objects, and iterates over the input string only once.
Conclusion
We’ve covered some techniques to replace multiple consecutive white spaces with single white spaces. If you value simplicity and conciseness, you may prefer the first or the second solution. If you value efficiency and memory usage, you may prefer the third solution.
If you’d like to explore more new and interesting stuff in the world of Flutter and Dart, take a look at the following articles:
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
- Flutter: ValueListenableBuilder Example
- How to find the iOS Bundle ID of a Flutter project
- Using Provider for State Management in Flutter
- Flutter: Creating a Fullscreen Modal with Search Form
- Using List.generate() in Flutter and Dart (4 examples)
- Flutter & Dart: How to Trim Leading and Trailing Whitespace
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.
I enjoy your website, obviously, but you should check the spelling on a number of your posts. A number of them have numerous spelling errors, which makes it difficult for me to tell the truth, but I will definitely return.