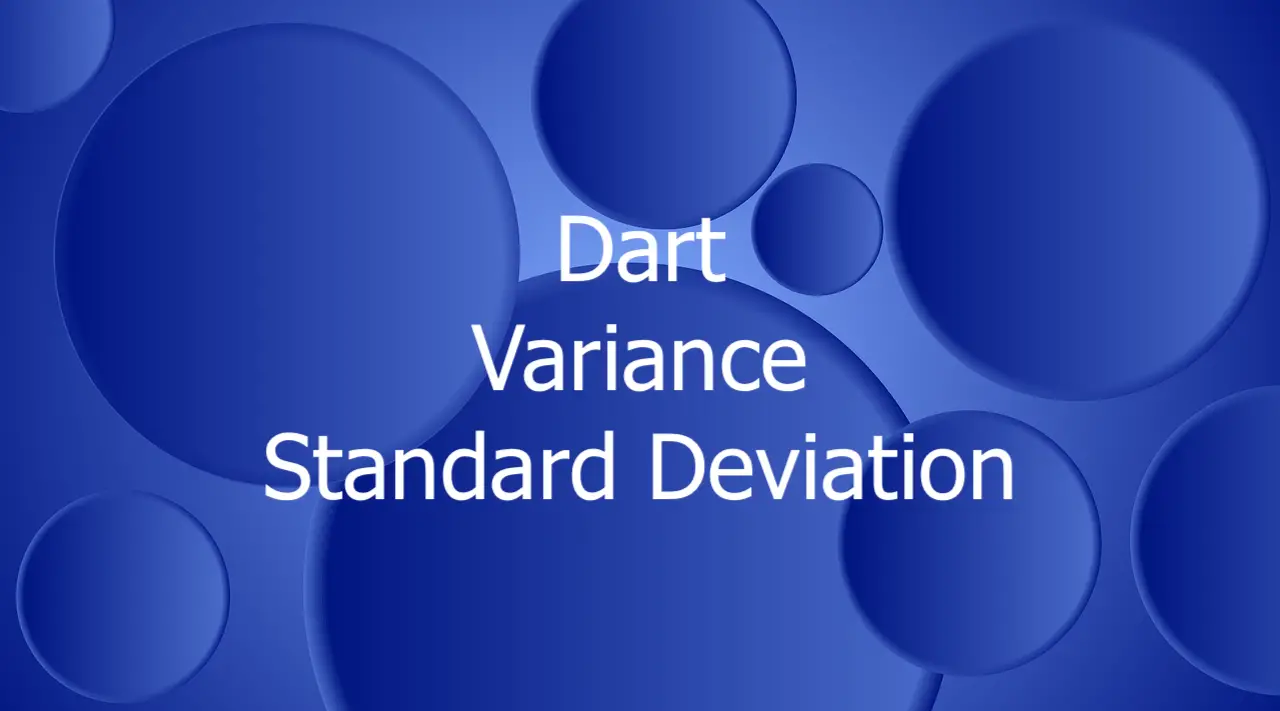
Variance is a statistical measure that quantifies the amount of variability or spread in a set of data. It is calculated as the average of the squared differences from the mean of the data.
Standard deviation is the square root of the variance and is another statistical measure of the amount of variability in a set of data. It tells you how much the data deviates from the mean, on average.
In Dart, you can calculate the variance and standard deviation of a list of numbers like this:
// Kindacode.com
// main.dart
import 'dart:math';
void main() {
List<double> data = [0.5, 1.1, 3, 4, 5, 10, 11, 9, 7.4, 8.2];
// Calculate the mean
double mean = data.reduce((a, b) => a + b) / data.length;
// Calculate the variance
double variance = data.map((x) => pow(x - mean, 2)).reduce((a, b) => a + b) /
(data.length - 1);
// Calculate the standard deviation
double standardDeviation = sqrt(variance);
print('Variance: $variance');
print('Standard Deviation: $standardDeviation');
}
Output:
Variance: 13.888444444444444
Standard Deviation: 3.7267203335432137
If you want to round the results to 2 decimals numbers, use the toStringFixed() method as follows:
var roundedVariance = variance.toStringAsFixed(2);
var roundedStandardDeviation = standardDeviation.toStringAsFixed(2);
Further reading:
- Dart: 2 Ways to Calculate the Power of a Number (Exponentiation)
- How to add/remove a duration to/from a date in Dart
- 2 Ways to Get a Random Item from a List in Dart (and Flutter)
- How to Create a Stopwatch in Flutter
- Flutter: 2 Ways to Create an Onboarding Flow (Intro Slider)
- 2 Ways to Create Typewriter Effects in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.