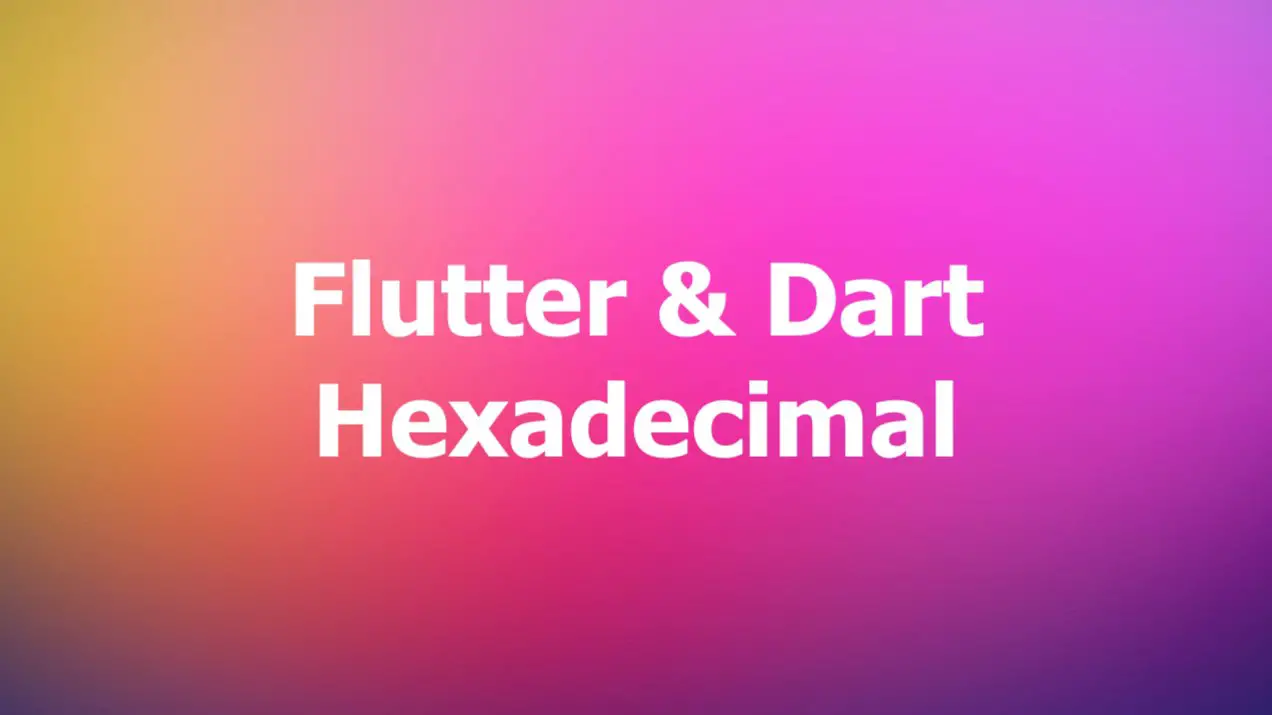
This practical shows you how to convert a number (integer) or a string to a hexadecimal value in Dart (and Flutter as well).
Turn a Number into Hex
Calling the toRadixString() method with a radix of 16 will produce a hex string from an input integer.
Code example:
// KindaCode.com
import 'package:flutter/foundation.dart';
void main() {
int number = 20232024;
String hexString = number.toRadixString(16);
debugPrint(hexString);
}
Output:
134b758
Convert a String to Hex
The main idea here is to use the runes property to get a list of Unicode code points for each character in the input string. Then, call the map() function to convert each code point to a hexadecimal string using toRadixString(16).
Example:
// KindaCode.com
import 'package:flutter/foundation.dart';
void main() {
String input = "Welcome to KindaCode.com!";
List<int> charCodes = input.runes.toList();
String hexString = charCodes.map((code) => code.toRadixString(16)).join('');
debugPrint(hexString);
}
Output:
57656c636f6d6520746f204b696e6461436f64652e636f6d21
That’s it. Further reading:
- Flutter & Dart: Convert Strings to Binary
- Flutter & Hive Database: CRUD Example
- Flutter & SQLite: CRUD Example
- Flutter Stream.periodic: Tutorial & Example
- How to Create a Countdown Timer in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.