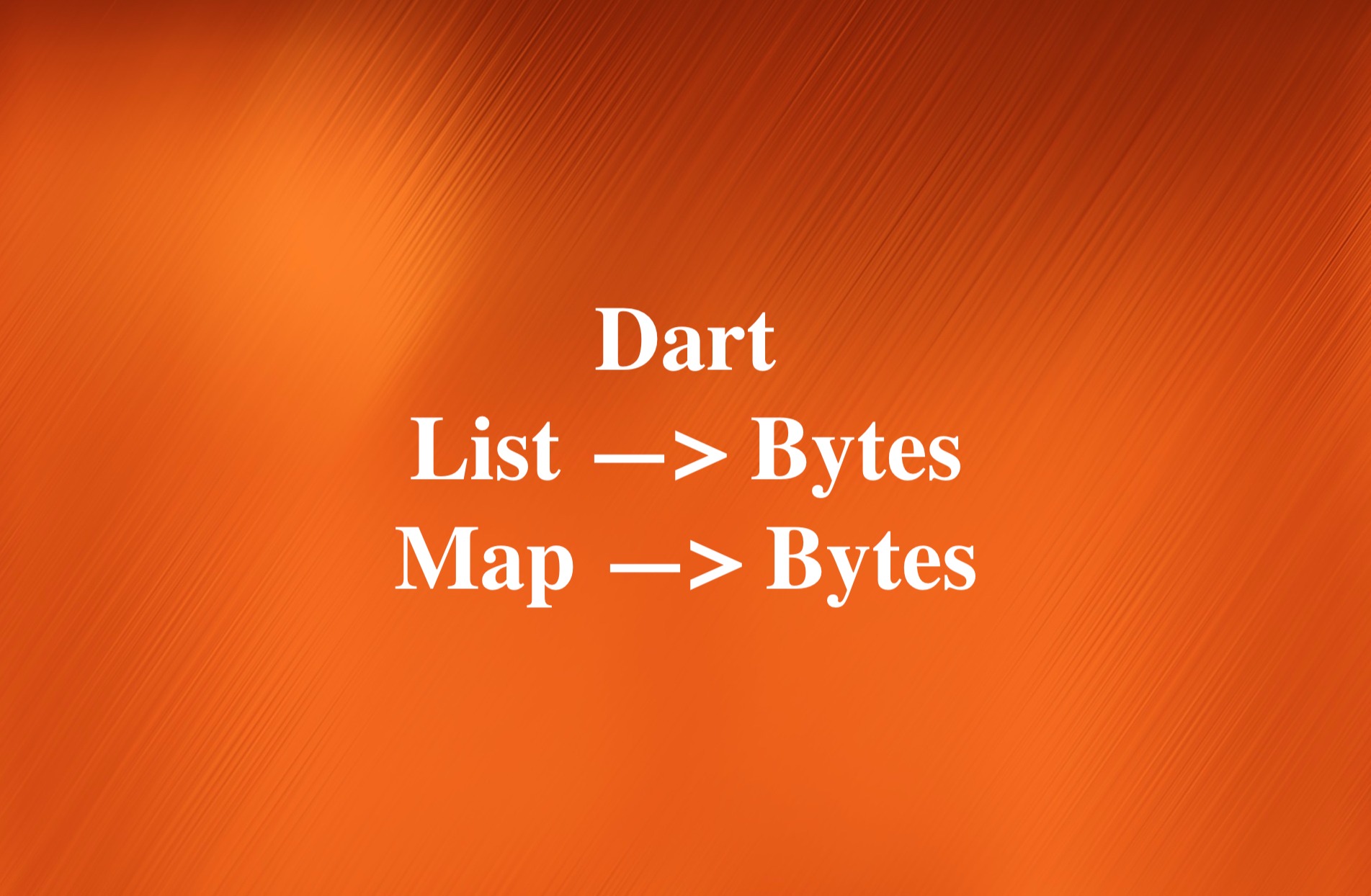
Bytes are units of digital information that represent binary data. A byte consists of eight bits, which are either 0 or 1. This concise, example-based article will show you how to convert a list or a map into bytes in Dart (and Flutter as well). Let’s begin!
Convert a List to Bytes
To turn a list into bytes, you can use the Utf8Codec
class, which encodes and decodes strings using the UTF-8 format. UTF-8 is a standard way of representing text using bytes. You can create an instance of Utf8Codec
and call its encode
method with the list as the argument. The encode
method will return a list of bytes that represents the original list as a string.
Example:
import 'dart:convert';
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
// a list of numbers
final list1 = [1, 2, 3];
// a list of strings
final list2 = ['a', 'b', 'kindacode.com'];
// Convert the list into bytes using Utf8Codec
const codec = Utf8Codec();
final bytesList1 = codec.encode(list1.toString());
final bytesList2 = codec.encode(list2.toString());
if (kDebugMode) {
print(bytesList1);
print(bytesList2);
}
}
Output:
[91, 49, 44, 32, 50, 44, 32, 51, 93]
[91, 97, 44, 32, 98, 44, 32, 107, 105, 110, 100, 97, 99, 111, 100, 101, 46, 99, 111, 109, 93]
Convert a Map to Bytes
In order to turn a map into bytes, you can use the JsonCodec
class, which encodes and decodes objects using the JSON format. JSON is a standard way of representing data using strings. You can create an instance of JsonCodec
and call its encode
method with the map as the argument. The encode
method will return a string that represents the original map as a JSON object. Then, you can use the Utf8Codec
class to convert the string into bytes, as explained above.
Example:
import 'dart:convert';
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
final map = {'key1': 'foo bar', 'key2': 123, 'key3': 'kindacode.com'};
const jsonCodec = JsonCodec();
final jsonMap = jsonCodec.encode(map);
final bytesMap = const Utf8Codec().encode(jsonMap);
if (kDebugMode) {
print(bytesMap);
}
}
Output:
[123, 34, 107, 101, 121, 49, 34, 58, 34, 102, 111, 111, 32, 98, 97, 114, 34, 44, 34, 107, 101, 121, 50, 34, 58, 49, 50, 51, 44, 34, 107, 101, 121, 51, 34, 58, 34, 107, 105, 110, 100, 97, 99, 111, 100, 101, 46, 99, 111, 109, 34, 125]
That’s it. Further reading:
- Using Try-Catch-Finally in Dart (3 examples)
- Using List.generate() in Flutter and Dart (4 examples)
- Flutter: Global, Unique, Value, Object, and PageStorage Keys
- Flutter AnimatedList – Tutorial and Examples
- Using Dio to fetch data in Flutter (with example)
- How to Create a Stopwatch in Flutter
- Flutter StreamBuilder: Tutorial & Examples (Updated)
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.