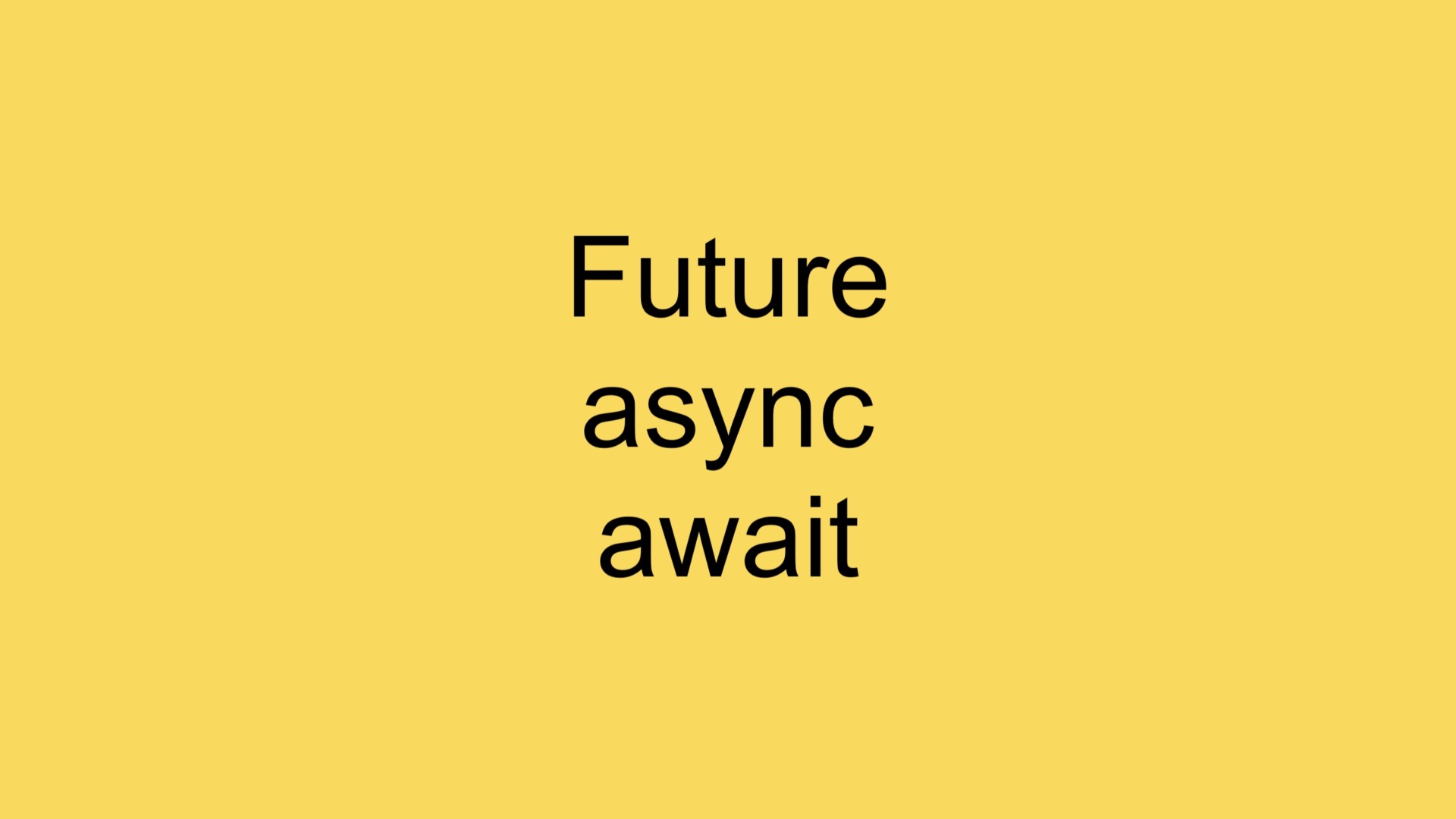
A few examples of using Future
, async
, and await
in Flutter applications.
Simple Example
This example simply prints “Doing…” and “Task completed!” to the console. However, the first will appear before the later 2 seconds.
The code:
import 'package:flutter/foundation.dart' show debugPrint;
Future<void> doSomething() {
return Future.delayed(const Duration(seconds: 2), () {
debugPrint('Task completed!');
});
}
void main() {
doSomething();
debugPrint('Doing...');
}
When looking at your terminal window, you’ll see “Doing…“ before “Task completed!” like this:
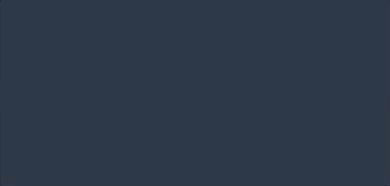
Fetching Data From APIs
Fetching data from APIs on remote servers is one of the most common use cases of Future
, async
, and await
in Flutter. For convenience, you should install the http
package, a Future-based library for making HTTP requests.
To install the http package, add http
and its version to the dependencies section in your pubspec.yaml
file by executing this:
flutter pub add http
Then run this command:
flutter pub get
The code:
import 'dart:convert';
import 'package:flutter/foundation.dart';
import 'package:http/http.dart' as http;
Future<void> loadData() async {
// This is an open api for testing
// Thanks to the Typicode team
final url = Uri.parse('https://api.slingacademy.com/v1/sample-data/users');
try {
final http.Response response = await http.get(url);
final loadedTodos = json.decode(response.body);
if (kDebugMode) {
print(loadedTodos);
}
} catch (err) {
rethrow;
}
}
// Call the _loadData function somewhere
void main() {
loadData();
}
Output:
{success: true, message: Sample data for testing and learning purposes, total_users: 1000, offset: 0, limit: 10, users: [{id: 1, gender: female, date_of_birth: 2002-04-26T00:00:00, job: Herbalist, city: Humphreyfurt, zipcode: 79574, latitude: 13.032103, profile_picture: https://api.slingacademy.com/public/sample-users/1.png, email: [email protected], first_name: Kayla, last_name: Lopez, phone: +1-697-415-3345x5215, street: 3388 Roger Wells Apt. 010, state: Vermont, country: Jordan, longitude: 112.16014}, {id: 2, gender: female, date_of_birth: 1924-05-14T00:00:00, job: Technical author, city: West Angelaside, zipcode: 44459, latitude: 51.5214995, profile_picture: https://api.slingacademy.com/public/sample-users/2.png, email: [email protected], first_name: Tina, last_name: Patrick, phone: 800-865-4932, street: 4564 Gamble Light Suite 885, state: Kansas, country: Greenland, longitude: -21.22766}, {id: 3, gender: female, date_of_birth: 1998-04-23T00:00:00, job: P<…>
If you want to fully print a very long output, see this article: How to Fully Print a Long String/List/Map in Flutter.
Further reading:
- Flutter: ListView Pagination (Load More) example
- Best Libraries for Making HTTP Requests in Flutter
- Flutter & Hive Database: CRUD Example
- Flutter: Firebase Remote Config example
- How to encode/decode JSON in Flutter
- Using Try-Catch-Finally in Dart (3 examples)
- Using List.generate() in Flutter and Dart (4 examples)
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.