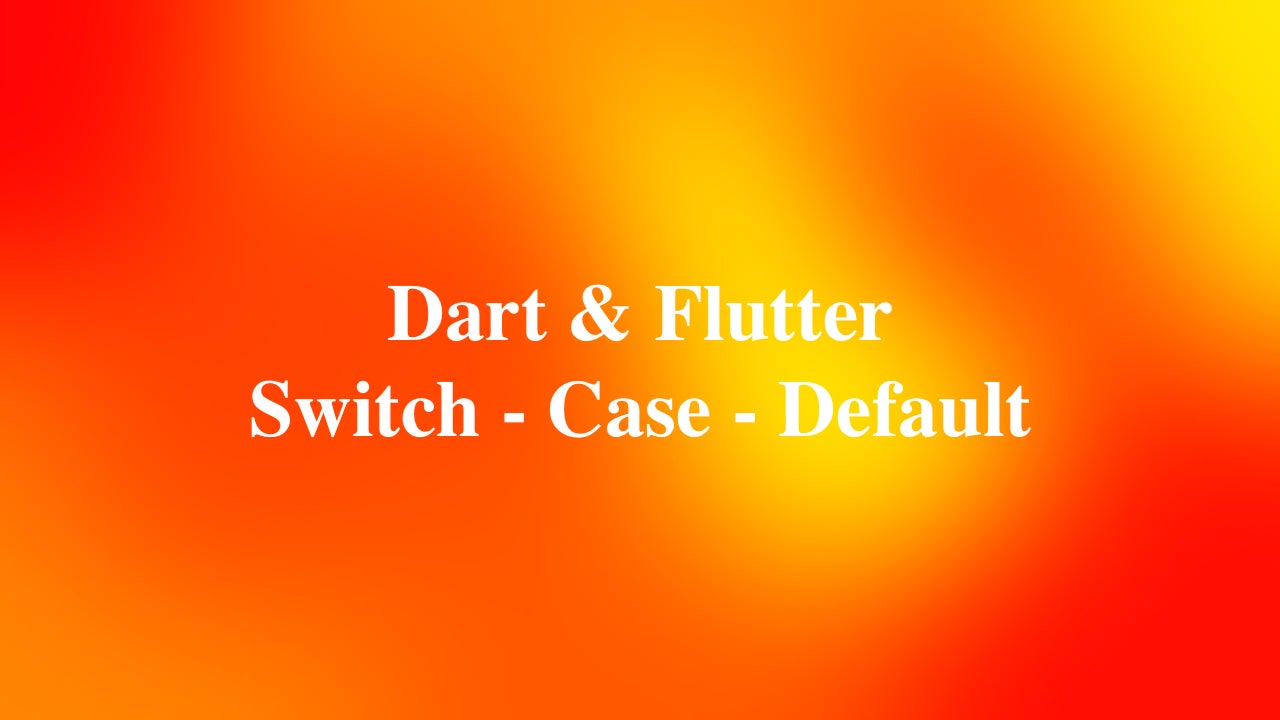
Overview
In Dart and Flutter, the switch…case…default
statement is used to avoid the long chain of the if-else
statement. It is a simplified form of a nested if-else
statement. The value of a variable is compared with multiple cases, and if a match is found, then it executes a block of code associated with that particular case.
Syntax
In general, you’ll use the switch
statement like so:
switch (expression) {
case value1:
// code block for value1
break;
case value2:
// code block for value2
break;
...
default:
// code block for no match
}
Where:
expression
: A variable or an expression that evaluates to a value of typeint
,String
, or any compile-time constant.value1
,value2
, …: Constant values that are comparable with the expression using the==
operator. Each case should be distinct and end with abreak
,continue
,return
, orthrow
statement to exit theswitch
.default
: An optional case that is executed when no other case matches theexpression
. It must be the last case in theswitch
.
Enough of words. Let’s see some actual code to see how the switch
statement works in practice.
Examples
Basic switch…case…default statement
This example shows how to use a basic switch…case…default
statement to print different messages based on an integer variable.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
void main() {
int number = 2;
switch (number) {
case 0:
debugPrint('zero!');
break;
case 1:
debugPrint('one!');
break;
case 2:
debugPrint('two!');
break;
default:
debugPrint('choose a different number!');
}
}
Output:
two!
Switch…case…default statement with String values
This example uses a switch…case…default
statement with String
values to print different messages based on an animal’s name.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
void main() {
String animal = 'tiger';
switch (animal) {
case 'cat':
debugPrint("it's a cat");
break;
case 'dog':
debugPrint("it's a dog");
break;
case 'tiger':
debugPrint("it's a tiger");
break;
default:
debugPrint("it's not an animal");
}
}
Output:
it's a tiger
Switch…case…default statement with fall-through cases
This example demonstrates how to use a switch…case…default
statement with fall-through cases, which means that multiple cases can be executed without breaking out of the switch
.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
void main() {
int number = 3;
switch (number) {
case -5:
case -4:
case -3:
case -2:
case -1:
debugPrint('negative!');
break;
case 0:
debugPrint('zero!');
break;
case 1:
case 2:
case 3:
case 4:
case 5:
debugPrint('positive!');
break;
default:
debugPrint('choose a number between -5 and 5!');
}
}
Output:
positive!
Using switch…case with labels
Labels are identifiers that can be used to mark a case
clause in a switch
statement, and then be referenced by a continue
statement to jump to that case. This can be useful when you want to execute more than one case clause for a given value of the expression
. Here is an example that uses a label (rainy
) to print an additional message if the season of the year is spring.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show debugPrint;
void main() {
String season = 'spring';
switch (season) {
case 'spring':
debugPrint('It is spring time!');
continue rainy; // continue to the rainy label
case 'summer':
debugPrint('It is summer time!');
break;
case 'autumn':
debugPrint('It is autumn time!');
break;
case 'winter':
debugPrint('It is winter time!');
break;
rainy: // this is the rainy label
case 'rainy':
debugPrint('It is rainy time!');
break;
default:
debugPrint('Invalid season!');
}
}
Output:
It is spring time!
It is rainy time!
Conclusion
You’ve learned the fundamentals of the switch
statement and examined several examples of using it in practice. If you’d like to explore more new and interesting stuff in Flutter and Dart, take a look at the following articles:
- Flutter AnimatedList – Tutorial and Examples
- Using Dio to fetch data in Flutter (with example)
- Flutter: Caching Network Images for Big Performance gains
- Using Try-Catch-Finally in Dart (3 examples)
- Using List.generate() in Flutter and Dart (4 examples)
- Flutter & Dart: Sorting a List of Objects/Maps by Date
- 2 Ways to Get a Random Item from a List in Dart (and Flutter)
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.