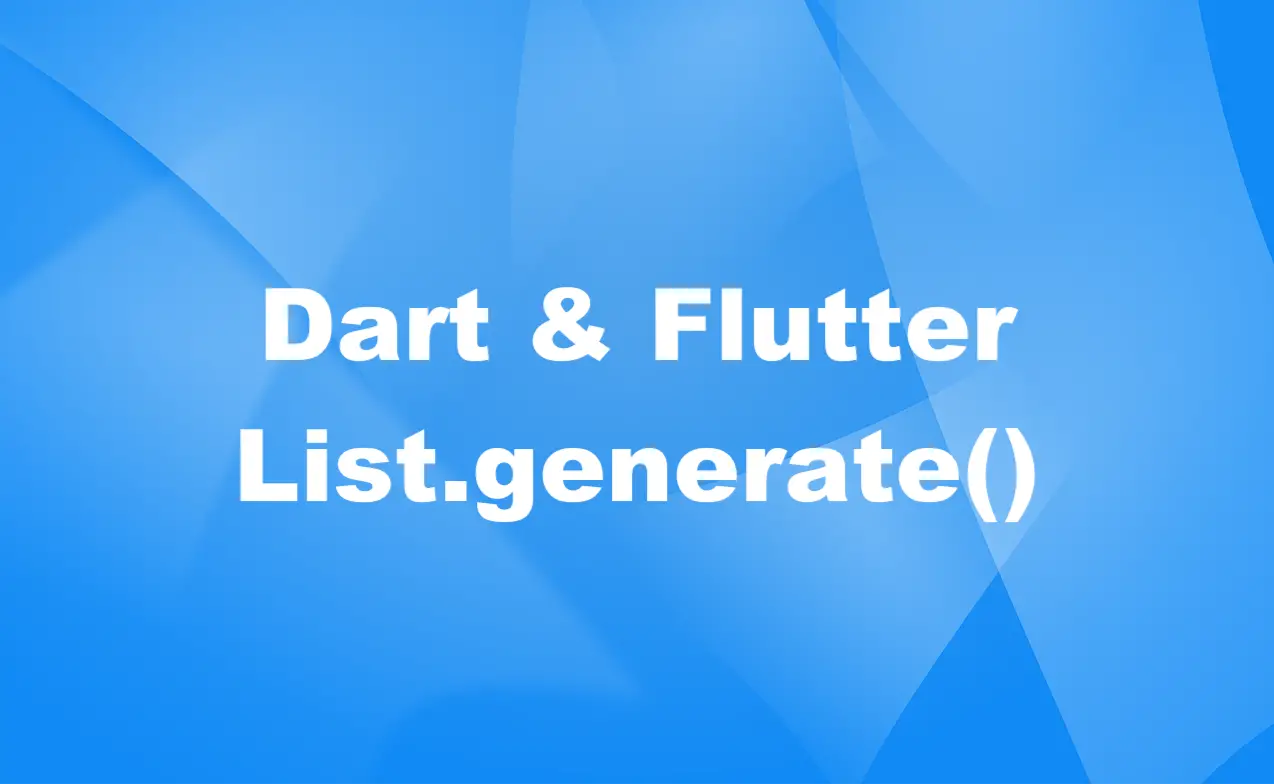
This concise, exampled-base article helps you deeply understand the List.generate()
constructor in Dart (and Flutter as well). Without any further ado (such as explaining what Flutter is or rambling about its awesome features), let’s get started.
Overview
The purpose of the List.generate()
constructor is to generate a list of values based on a given length and a generator function.
Syntax:
List<E>.generate(
int length,
E generator(int index),
{bool growable = true}
)
Where E
is the type of the elements in the list, length
is the number of elements in the list, generator
is the function that creates the values for each index, and growable
is a boolean flag that indicates whether the list can be modified after creation.
Let’s see some practical examples for more clarity.
Examples
Generating a List of Squares
This example demonstrates how to create a list of squares of integers from 0 to n
using the List.generate()
constructor.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart';
void main() {
// Declare the number of elements
int n = 10;
List<int> squares =
List<int>.generate(n + 1, (int index) => index * index, growable: false);
if (kDebugMode) {
print(squares);
}
}
Output:
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81, 100]
Creating a list of random numbers
In order to create a list of random numbers, we need to import the dart:math
library and create an instance of the Random
class. Then we can use the List.generate()
constructor with a generator function that calls the nextInt()
method on the random object with the upper bound as an argument. We can also add the lower bound to the result to get numbers within the desired range.
Here’s the code:
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart';
import 'dart:math';
void main() {
// Create a random object
Random random = Random();
// Create a list of random numbers between lower and upper bound
int lower = 10;
int upper = 50;
// Set the desired length of the list
int length = 10;
List<int> randomNumbers = List<int>.generate(
length, (int index) => lower + random.nextInt(upper - lower));
if (kDebugMode) {
print(randomNumbers);
}
}
Output:
[47, 44, 30, 25, 22, 36, 19, 22, 40, 48]
Due to the randomness, the resulting list will change each time you execute the code.
Creating a List of Maps
In this example, we’ll make use of List.generate()
to create a list of items where each item has an id, a name, and a price.
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart';
import 'dart:math';
void main() {
// Define how many items you want to generate
const length = 5;
// Initialize the random object
final random = Random();
List<Map<String, dynamic>> items = List<Map<String, dynamic>>.generate(
length,
(index) =>
{'id': index, 'name': 'Item $index', 'price': random.nextInt(100)});
if (kDebugMode) {
print(items);
}
}
Output (may vary):
[{id: 0, name: Item 0, price: 33}, {id: 1, name: Item 1, price: 99}, {id: 2, name: Item 2, price: 25}, {id: 3, name: Item 3, price: 51}, {id: 4, name: Item 4, price: 78}]
Generating a List of Widgets
This example creates a list of widgets by using the List.generate()
construction and then displays that list on the screen with the ListView.builder()
constructor.
// KindaCode.com
// main.dart
import 'package:flutter/material.dart';
import 'dart:core';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.blue),
useMaterial3: true,
),
home: const KindaCodeDemo(),
);
}
}
class KindaCodeDemo extends StatefulWidget {
const KindaCodeDemo({super.key});
@override
State<KindaCodeDemo> createState() => _KindaCodeDemoState();
}
class _KindaCodeDemoState extends State<KindaCodeDemo> {
// Create a list of 50 widgets
List<Widget> widgets = List<Widget>.generate(50, (int index) {
// Create different text and icons for each widget
String name = 'Item ${index + 1}';
IconData icon;
switch (index % 3) {
case 0:
icon = Icons.run_circle;
break;
case 1:
icon = Icons.cloud;
break;
default:
icon = Icons.sunny;
break;
}
// Return a Card widget whose child is a ListTile
return Card(
margin: const EdgeInsets.symmetric(horizontal: 20, vertical: 5),
color: Colors.amber[100],
child: ListTile(
title: Text(name),
subtitle: Text("This is the subtitle of $name"),
leading: Icon(icon),
),
);
});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: ListView.builder(
itemBuilder: (_, index) => widgets[index], itemCount: widgets.length),
);
}
}
The result:
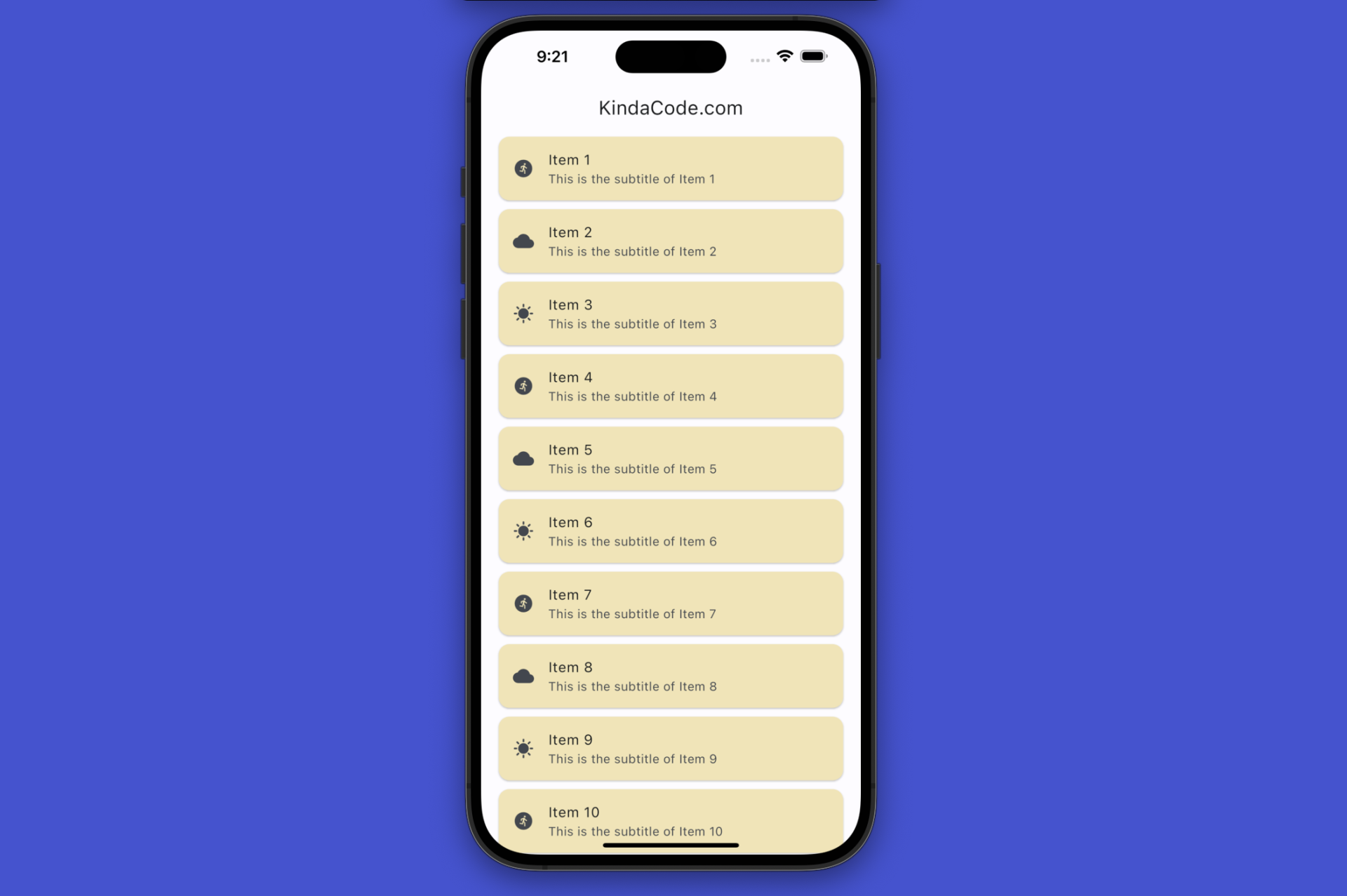
Conclusion
The List.generate()
constructor is a very useful and versatile feature of the Dart programming language. It allows you to create lists of any type and length with a simple and concise syntax. It also gives you the flexibility to choose whether the list is growable or not, and to customize the values for each index using a generator function. This brings to the table a great way to create dynamic and complex data structures for various purposes.
If you’d like to learn more new and interesting things in Flutter and Dart, take a look at the following articles:
- Dart & Flutter: Convert String/Number to Byte Array (Byte List)
- How to Create a Countdown Timer in Flutter
- Flutter AnimatedList – Tutorial and Examples
- Flutter StreamBuilder: Tutorial & Examples (Updated)
- Sorting Lists in Dart and Flutter (with Examples)
- 2 Ways to Get a Random Item from a List in Dart (and Flutter)
- Dart: Converting a List to a Set and vice versa
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.