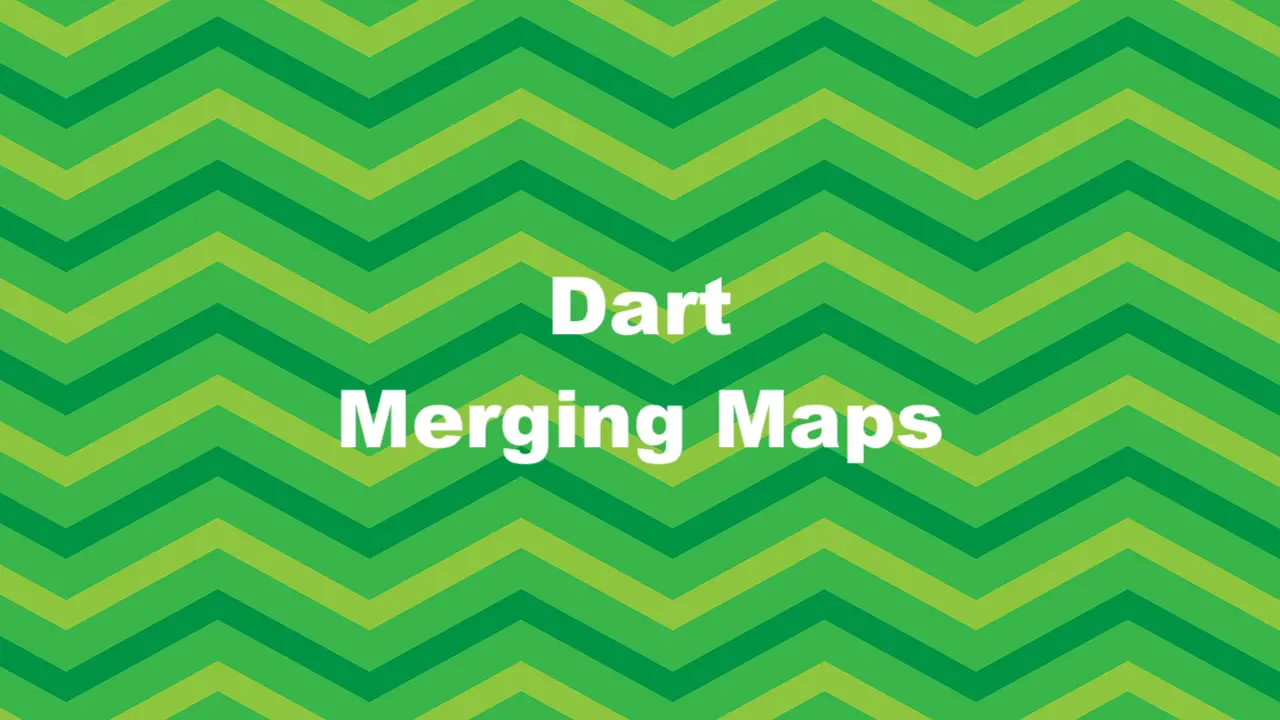
When working with Dart and Flutter, there might be times when you need to merge two maps, such as:
- You may want to combine two maps that contain different properties of the same object. For instance, you may have a map that stores the user’s name, email, and phone number and another map that stores the user’s address, preferences, and history. By joining these two ones, you can create a single map that contains all the information about the user.
- You may want to merge two maps that represent different sources of data, such as a local cache and a remote server.
- You may want to merge two maps that contain different configurations or settings for your app, such as a default configuration and a user-defined configuration.
This concise, exampled-base article will walk you through a couple of different approaches to merging two given maps in Dart. Let’s begin!
Using the spread operator
This approach uses the spread operator (...
) to merge two maps into a new map. The spread operator allows you to expand the elements of an iterable (in this case, the key-value pairs of the maps) into a new collection.
Example:
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
Map<String, int> map1 = {'a': 1, 'b': 2};
Map<String, int> map2 = {'c': 3, 'd': 4, 'kindacode.com': 5};
Map<String, int> mergedMap = {...map1, ...map2};
if (kDebugMode) {
print(mergedMap);
}
}
Output:
{a: 1, b: 2, c: 3, d: 4, kindacode.com: 5}
There is one important point you should be aware of: if there are duplicate keys in the maps being merged, the value in the second map will overwrite the value in the first map.
Using the addAll() method
This solution uses the addAll()
method to merge two maps. The addAll()
method adds all the key-value pairs from one map to another map.
Example:
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
Map<String, int> map1 = {'a': 1, 'b': 2};
Map<String, int> map2 = {'c': 3, 'd': 4, 'kindacode.com': 5};
// merge map2 into map1
map1.addAll(map2);
if (kDebugMode) {
print(map1);
}
}
Output:
{a: 1, b: 2, c: 3, d: 4, kindacode.com: 5}
This technique directly modifies the first map by adding all the key-value pairs from the second map. Similarly to the preceding solution, if there are duplicate keys, the value in the second map will overwrite the value in the first one.
Using the putIfAbsent() method
The putIfAbsent()
method adds the key-value pair from one map to another map only if the key is not already present in the second map.
Example:
// KindaCode.com
// main.dart
import 'package:flutter/foundation.dart' show kDebugMode;
void main() {
Map<String, int> map1 = {'a': 1, 'b': 2, 'c': 33};
Map<String, int> map2 = {'c': 3, 'd': 4, 'kindacode.com': 5};
map1.forEach((key, value) {
map2.putIfAbsent(key, () => value);
});
if (kDebugMode) {
print(map2);
}
}
Output:
{c: 3, d: 4, kindacode.com: 5, a: 1, b: 2}
This solution provides more control over handling duplicate keys. By using the putIfAbsent()
method, you can decide whether the value from the first map should overwrite the value in the second map or not. However, it requires iterating over the key-value pairs of the first map, which may impact performance for large maps.
Conclusion
We’ve gone through some techniques to merge multiple maps into a single one. Consider your specific use case, the size of the maps, and the possibility of duplicate keys when choosing the most suitable solution.
If you’d like to explore more new and interesting stuff in Flutter and Dart, take a look at the following articles:
- 6 Ways to iterate through a list in Dart and Flutter
- Dart: 2 Ways to Find Common Elements of 2 Lists
- Flutter CupertinoSliverNavigationBar: Tutorial & Example
- Flutter and Firestore Database: CRUD example
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Using Dio to fetch data in Flutter (with example)
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.