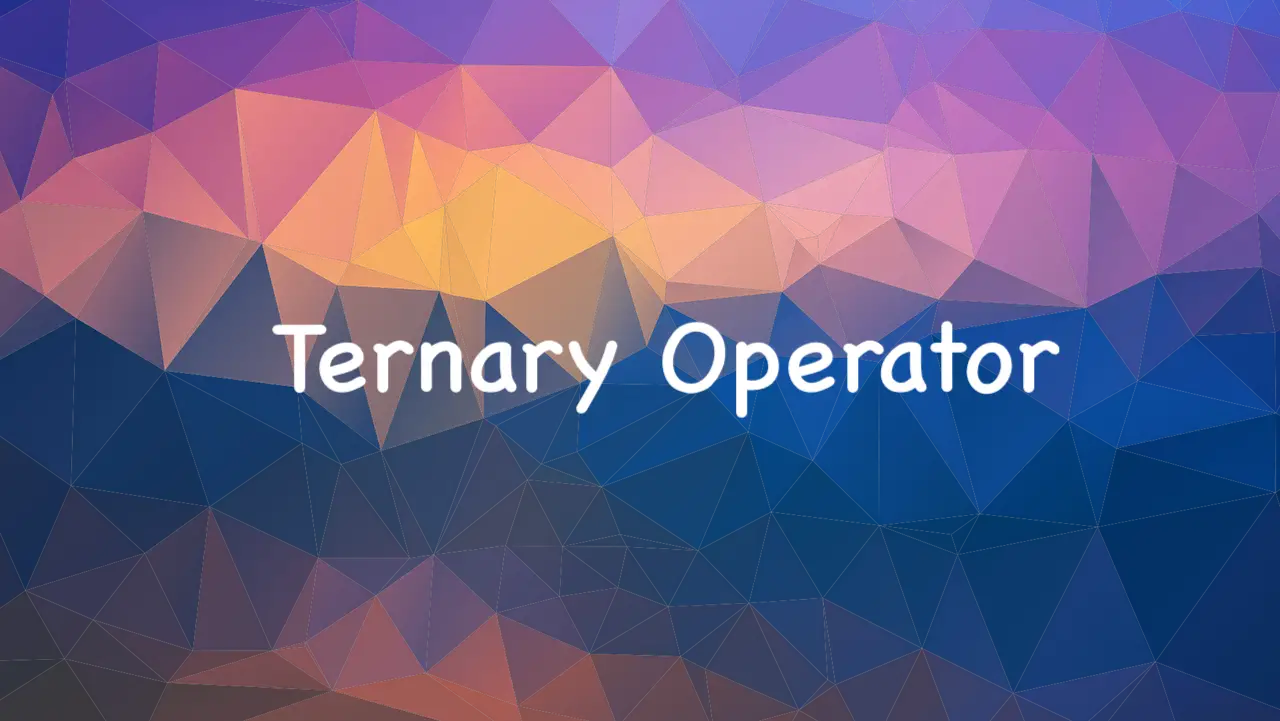
This article is a deep dive into conditional (ternary) operators in Dart and Flutter.
The Basic
The conditional (ternary) operator is just a Dart operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy.
The syntax:
condition ? exprIfTrue : exprIfFalse
Where:
- condition: An expression whose value is used as a condition.
- exprIfTrue: An expression that is evaluated if the condition evaluates to a truthy value (one which equals or can be converted to true).
- exprIfFalse: An expression that is executed if the condition is falsy (that is, has a value that can be converted to false).
Examples:
String message(bool isValid) {
return isValid ? 'This is valid' : 'This is not valid';
}
void main() {
print(message(true));
}
Center(
child: isLogin ? Text('You are a member') : Text('Hello Guest'),
),
Conditional chains
The ternary operator is right-associative, which means it can be “chained” to check multiple conditions in turn.
The syntax:
condition1 ? value1
: condition2 ? value2
: condition3 ? value3
: condition4 ? value4
: condition5 ? value5 // and so on
: valueN;
Example:
void main() {
int age = 30;
String output = age < 1
? 'Infrant'
: age < 4
? 'Baby'
: age < 12
? 'Middle Childhood'
: age < 18
? 'Adolescence'
: 'Grown Up';
print(output);
}
Null Checking Expression
This expression uses a double-question mark and can be used to test for null.
The syntax:
expr1 ?? expr2
If expr1 is non-null, returns its value; otherwise, evaluates and returns the value of expr2.
Example:
// Flutter
Center(
child: Text(name ?? 'No Name Found'),
),
Ternary operator vs If-else statement
The conditional expression condition ? expr1: expr2 has a value of expr1 or expr2 while an if-else statement has no value. A statement often contains one or more expressions, but an expression can’t directly contain a statement.
There are some things that you can do with the ternary operator but can’t do with if-else. For example, if you need to initialize a constant or reference:
const int number = (some conditions) ? 100 : 1;
Writing Concise Code
In the vast majority of cases, you can do the same thing with a ternary operator and an if-else statement. However, using ? : helps us avoid redundantly repeating other parts of the same statements/function-calls, for example:
if (someCondition)
return x;
else
return y;
Compare with:
return condition ? x : y;
Conclusion
You’ve learned the fundamentals of using conditional expressions when programming in Dart. Continue exploring more new and interesting stuff by taking a look at the following articles:
- Sorting Lists in Dart and Flutter (5 Examples)
- Flutter and Firestore Database: CRUD example
- Flutter & Dart: Get File Name and Extension from Path/URL
- Flutter & Dart: Sorting a List of Objects/Maps by Date
- How to Create a Countdown Timer in Flutter
- Flutter: Making a Tic Tac Toe Game from Scratch
You can also take a tour around our Flutter topic page, or Dart topic page for the latest tutorials and examples.