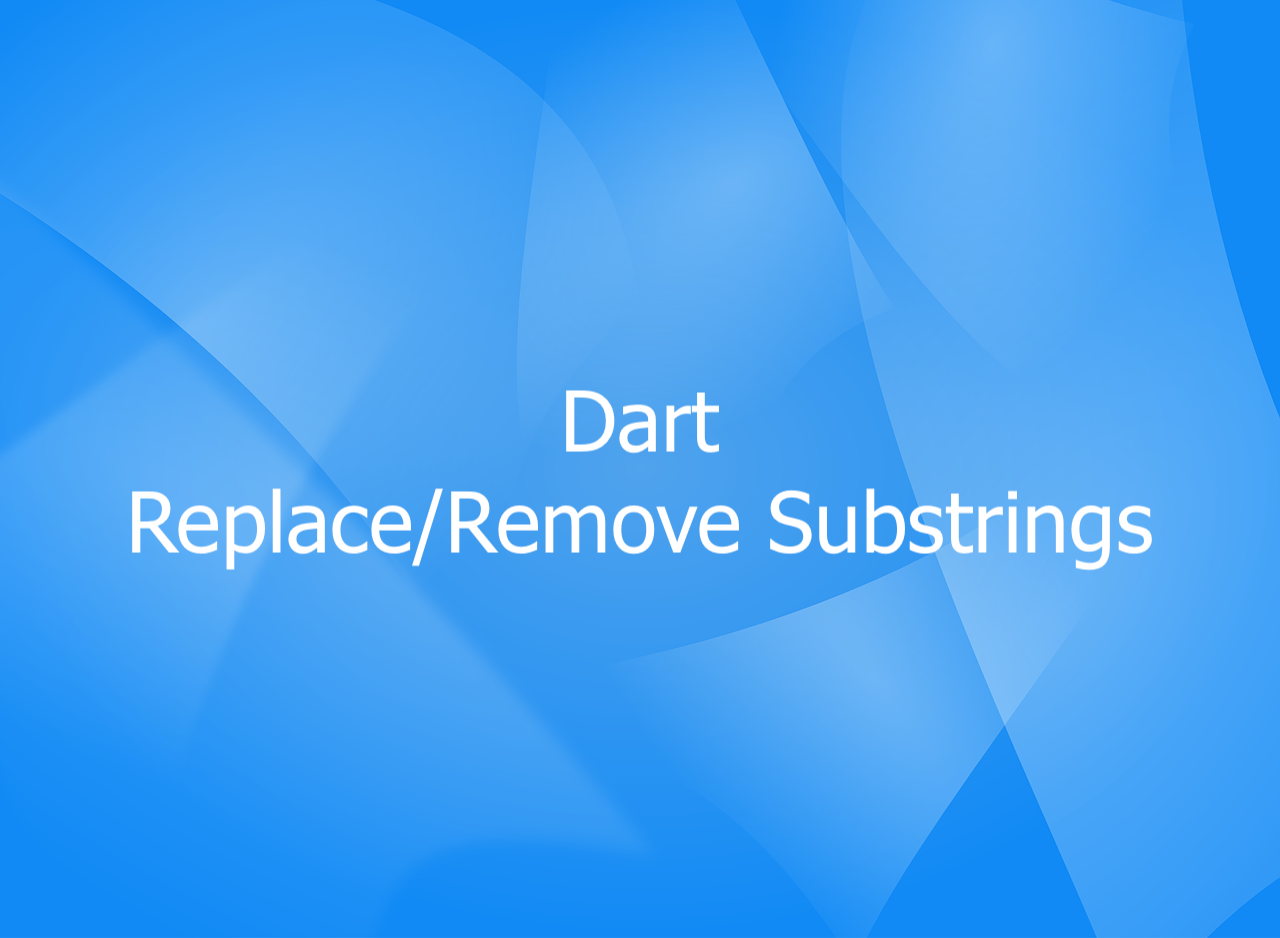
In Dart (and Flutter as well), you can replace one or multiple substrings in a parent string by using the following built-in methods:
- replaceAll()
- replaceAllMapped()
- replaceFirst()
- replaceFirstMapped()
- replaceRange()
You can remove substrings from a string by replacing them with empty strings (”).
Now it’s time for practical examples.
Using replaceAll()
In this example, we use the replaceAll() method to replace all occurrences of the substring “day” in a given message with “night”.
The code:
void main() {
String str = "Welcome to Kindacode.com. Have a nice day and happy coding!";
String substr = "day";
String replacement = "night";
String newStr = str.replaceAll(substr, replacement);
print(newStr);
}
Output:
Welcome to Kindacode.com. Have a nice night and happy coding!
Using replaceAllMapped()
In this example, we use the replaceAllMapped() method to replace all five-letter words in the string “I like playing video games as well as reading articles on Kindacode.com” with “*****”. We pass a regular expression pattern to the RegExp constructor to match any five-letter word and use an anonymous function to specify the replacement value.
The code:
void main() {
String str =
"I like playing video games as well as reading articles on Kindacode.com";
// matches any five-letter word
var pattern = RegExp(r'\b\w{5}\b');
String replacement = "*****";
String newStr = str.replaceAllMapped(pattern, (match) => replacement);
print(newStr);
}
Output:
I like playing ***** ***** as well as reading articles on Kindacode.com
See also: Regular Expression Examples in Flutter
Using replaceRange()
In the code snippet below, we use the replaceRange() method to replace a range of characters in the string “Goodbye cruel world!” with the string “Hello”. We specify the starting and ending indices of the range to be replaced and the replacement value.
The code:
void main() {
String str = "Goodbye cruel world!";
int start = 0;
int end = 7;
String replacement = "Hello";
String newStr = str.replaceRange(start, end, replacement);
print(newStr);
}
Output:
Hello cruel world!
Using replaceFirst()
This example uses the replaceFirst() method to replace the first occurrence of the word “bad” in the string “I see bad days coming. I head bad songs playing. I will be sad.” with “good”.
The code:
void main() {
String str =
"I see bad days coming. I head bad songs playing. I will be sad.";
String substr = "bad";
String replacement = "good";
String newStr = str.replaceFirst(substr, replacement);
print(newStr);
}
Output:
I see good days coming. I head bad songs playing. I will be sad.
Using replaceFirstMapped()
This example uses the replaceFirstMapped() method to remove the first numeric substring in the string “kindacode.com123449949493 is a fast website.”. We use an anonymous function to specify the replacement value.
The code:
void main() {
String str = "kindacode.com123449949493 is a fast website.";
// match all numeric characters
RegExp pattern = RegExp(r"\d+");
// use an empty string as replacement
// so that the matched characters will be removed
String replacement = "";
String newStr = str.replaceFirstMapped(pattern, (match) => replacement);
print(newStr);
}
Output:
kindacode.com is a fast website.
Conclusion
We’ve walked through 5 examples where each example demonstrates a different way to replace substrings in a parent string. Continue learning more new and awesome stuff in Dart and Flutter by taking a look at the following articles:
- Dart regular expressions to check people’s names
- Flutter & Dart: Convert a String into a List and vice versa
- How to Create a Stopwatch in Flutter
- How to Create a Sortable ListView in Flutter
- Flutter: 2 Ways to Create an Onboarding Flow (Intro Slider)
- 4 Ways to Create Full-Width Buttons in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.