In this article, we’ll build a small Flutter app to demonstrate how to validate email while the user is typing. This will help him or her quickly identifies if there is a typo or mistake going on. Here’s what you’ll see when running the code at the end of this tutorial:
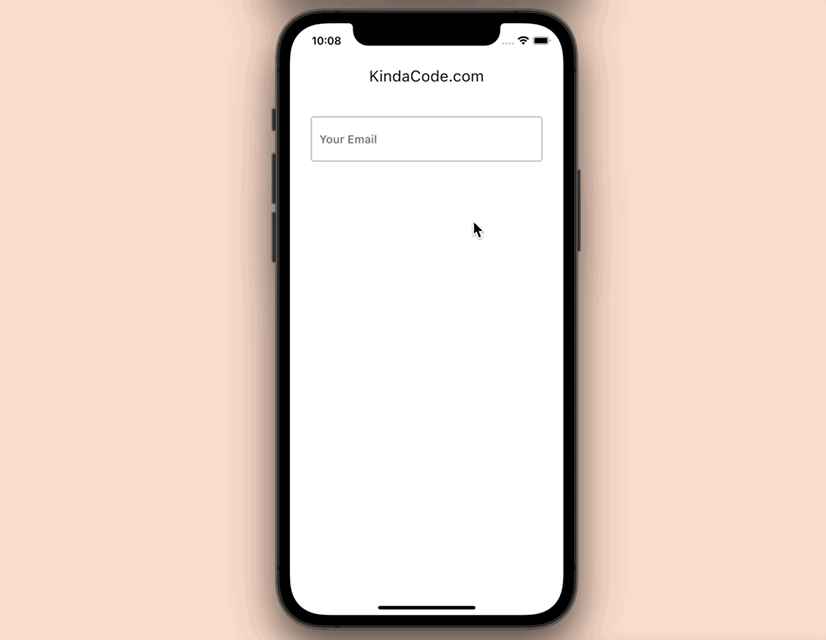
Let’s Write Code
1. Create a brand new Flutter project
flutter create email_validation_app
2. Install the email_validator package
Run:
flutter pub add email_validator
Then:
flutter pub get
Note: If you want to use a regular expression instead of a third-party plugin to validate email in Flutter, see this article.
3. Open your /lib/main.dart file, remove all of the default code and add the following:
import 'package:flutter/material.dart';
// import the email validator package
import 'package:email_validator/email_validator.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
theme: ThemeData(
// use Material 3
useMaterial3: true),
title: 'Flutter Tutorial',
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final _emailController = TextEditingController();
// This message will be displayed under the email input
String message = '';
void validateEmail(String enteredEmail) {
if (EmailValidator.validate(enteredEmail)) {
setState(() {
message = 'Your email seems nice!';
});
} else {
setState(() {
message = 'Please enter a valid email address!';
});
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Padding(
padding: const EdgeInsets.all(30),
child: Column(children: [
// Input email
TextField(
decoration: const InputDecoration(
labelText: 'Your Email', border: OutlineInputBorder()),
keyboardType: TextInputType.emailAddress,
controller: _emailController,
onChanged: (enteredEmail) => validateEmail(enteredEmail),
),
// Just add some space
const SizedBox(height: 20),
// Display the message
Text(message)
]),
));
}
}
4. Try it:
flutter run
Conclusion
You’ve learned a simple and quick solution to validate emails in Flutter. If you’d like to explore more new and fascinating things about the awesome SDK, take a look at the following articles:
- Flutter form validation example
- Flutter: Firebase Remote Config example
- Flutter: Making Beautiful Chat Bubbles (2 Approaches)
- Flutter: Creating OTP/PIN Input Fields (2 approaches)
- Using Provider for State Management in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.