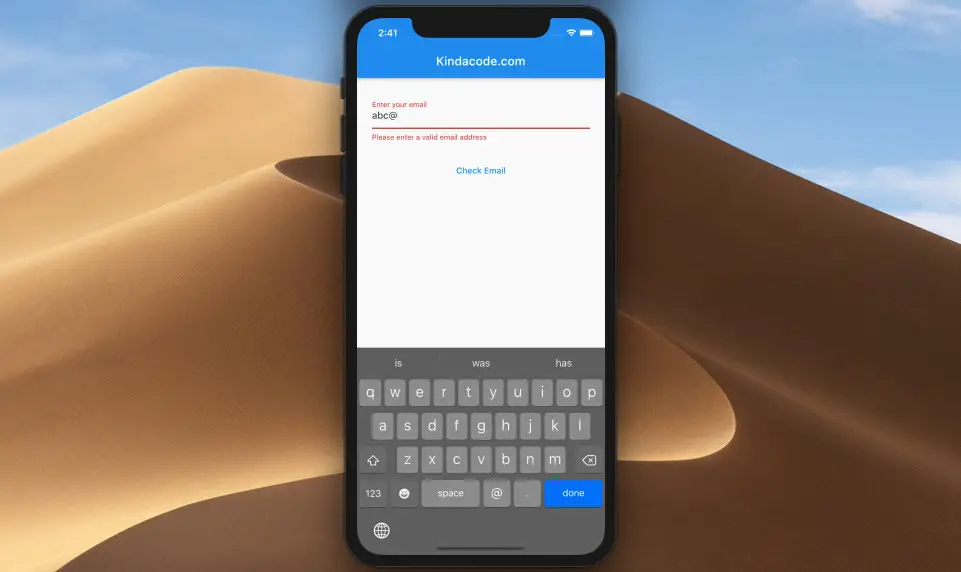
This article shows you an elegant method to validate email in Flutter. We’ll use the RegExp class of dart:core for working with regular expressions.
The email pattern:
r'\S+@\S+\.\S+'
The Code
Create a new Flutter project and replace the default code in main.dart with the following:
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData.light(),
home: const HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
final _form = GlobalKey<FormState>();
bool _isValid = false;
void _saveForm() {
setState(() {
_isValid = _form.currentState!.validate();
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Padding(
padding: const EdgeInsets.all(25),
// the Form here
child: Form(
key: _form,
child: Column(
children: [
TextFormField(
decoration:
const InputDecoration(labelText: 'Enter your email'),
keyboardType: TextInputType.emailAddress,
validator: (value) {
// Check if this field is empty
if (value == null || value.isEmpty) {
return 'This field is required';
}
// using regular expression
if (!RegExp(r'\S+@\S+\.\S+').hasMatch(value)) {
return "Please enter a valid email address";
}
// the email is valid
return null;
},
),
const SizedBox(height: 25),
TextButton(
onPressed: _saveForm, child: const Text('Check Email')),
const SizedBox(height: 25),
_isValid ? const Text('Your email seems nice!') : Container()
],
),
),
),
);
}
}
Try it:
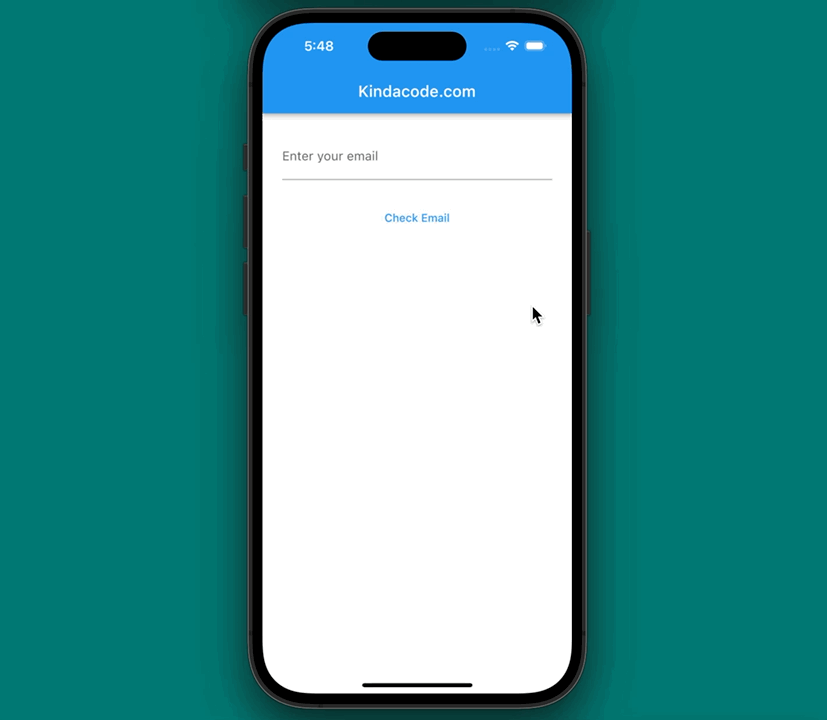
Hope this helps. Further reading:
- Best Libraries for Making HTTP Requests in Flutter
- Creating Masonry Layout in Flutter with Staggered Grid View
- Using BlockSemantics in Flutter: Tutorial & Example
- Flutter: Adding a Gradient Border to a Container (2 Examples)
- Flutter: TextField and Negative Numbers
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.