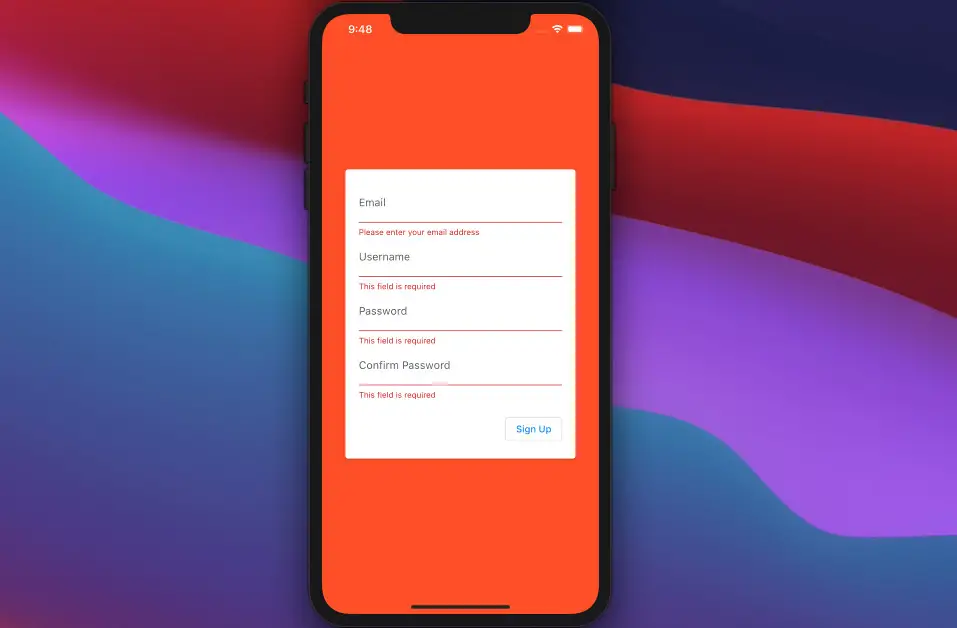
Many web and mobile applications have forms to allow users to enter some information such as names, email addresses, phone numbers, zip codes, passwords, etc.
Form validation in Flutter allows error messages to be displayed if the user has not correctly filled out text fields with the expected type of inputs, otherwise processes the provided information.
In this article, you will see an example of how to validate a form and user inputs in a Flutter application.
The Example
We’ll make a simple SIGN UP screen that requires the user to enter his/her email address, username, password, and confirmation password. The user information is valid if:
- The email address matches this regular expression pattern: r’\S+@\S+\.\S+’ (you can improve this pattern or use a third-party package if you want more accuracy).
- The username is at least four characters in length.
- The password is at least eight characters in length.
- The confirmation password matches the password.
App Preview
Note: If you’re using Safari, this demo video might not work nicely or not start at all. Please use Chrome, Edge, Firefox, or other web browsers instead.
The Code
The complete code in main.dart with explanations:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// Define a key to access the form
final _formKey = GlobalKey<FormState>();
String _userEmail = '';
String _userName = '';
String _password = '';
String _confirmPassword = '';
// This function is triggered when the user press the "Sign Up" button
void _trySubmitForm() {
final bool? isValid = _formKey.currentState?.validate();
if (isValid == true) {
debugPrint('Everything looks good!');
debugPrint(_userEmail);
debugPrint(_userName);
debugPrint(_password);
debugPrint(_confirmPassword);
/*
Continute proccessing the provided information with your own logic
such us sending HTTP requests, savaing to SQLite database, etc.
*/
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
color: Colors.deepOrange,
alignment: Alignment.center,
child: Center(
child: Card(
margin: const EdgeInsets.symmetric(horizontal: 35),
child: Padding(
padding: const EdgeInsets.all(20),
child: Form(
key: _formKey,
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
/// Eamil
TextFormField(
decoration: const InputDecoration(labelText: 'Email'),
validator: (value) {
if (value == null || value.trim().isEmpty) {
return 'Please enter your email address';
}
// Check if the entered email has the right format
if (!RegExp(r'\S+@\S+\.\S+').hasMatch(value)) {
return 'Please enter a valid email address';
}
// Return null if the entered email is valid
return null;
},
onChanged: (value) => _userEmail = value,
),
/// username
TextFormField(
decoration:
const InputDecoration(labelText: 'Username'),
validator: (value) {
if (value == null || value.trim().isEmpty) {
return 'This field is required';
}
if (value.trim().length < 4) {
return 'Username must be at least 4 characters in length';
}
// Return null if the entered username is valid
return null;
},
onChanged: (value) => _userName = value,
),
/// Password
TextFormField(
decoration:
const InputDecoration(labelText: 'Password'),
obscureText: true,
validator: (value) {
if (value == null || value.trim().isEmpty) {
return 'This field is required';
}
if (value.trim().length < 8) {
return 'Password must be at least 8 characters in length';
}
// Return null if the entered password is valid
return null;
},
onChanged: (value) => _password = value,
),
/// Confirm Password
TextFormField(
decoration: const InputDecoration(
labelText: 'Confirm Password'),
obscureText: true,
validator: (value) {
if (value == null || value.isEmpty) {
return 'This field is required';
}
if (value != _password) {
return 'Confimation password does not match the entered password';
}
return null;
},
onChanged: (value) => _confirmPassword = value,
),
const SizedBox(height: 20),
Container(
alignment: Alignment.centerRight,
child: OutlinedButton(
onPressed: _trySubmitForm,
child: const Text('Sign Up')))
],
)),
),
),
),
),
);
}
}
Conclusion
At this point, you should have a better understanding of validating user inputs in Flutter. If you want to validate the text field while the user is typing, read live email validation in Flutter. To explore more new and interesting things about Flutter, take a look at the following articles:
- Flutter & Hive Database: CRUD Example
- Flutter TextField: Styling labelText, hintText, and errorText
- Flutter: Making a Dropdown Multiselect with Checkboxes
- How to Create a Sortable ListView in Flutter
- Flutter: Caching Network Images for Big Performance gains
- Flutter: Making a Tic Tac Toe Game from Scratch
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.
very interesting
Eu posso criar um outro arquivo pra chamar esse parâmetro de cadastro de senhas, ou é necessário ficar em main.dart?