Using an image as a full-screen background will help your app convey more messages to the user, such as showing that your app is about education, entertainment, finances, etc.
In this article, we’ll go over 2 examples of using an image as a background for the entire screen of a Flutter application. The first example will use a single big network image and the second one will use a small pattern local image that is repeated to fill.
What is the point?
In general, the Scaffold widget is frequently used as the root widget but this one doesn’t support an option for setting image background so we need to wrap it within a Container widget. Then, we can set an image background for the Container like this:
Container(
decoration: BoxDecoration(
image: DecorationImage(
image: // a network image or a local image here,
)),
child: Scaffold(),
);
If you use a large image as the background image for the full screen, in many cases, it will not fit. To fix it, add this option to DecorationImage:
fit: BoxFit.cover,
If you use a small pattern, let it repeat over and over:
repeat: ImageRepeat.repeat,
To really make these transparent, we will jump into the 2 actual examples below.
Using a single Network Image as Background
Here’s the image used in this example:

The full code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
decoration: const BoxDecoration(
image: DecorationImage(
fit: BoxFit.cover,
image: NetworkImage(
'https://www.kindacode.com/wp-content/uploads/2021/01/blue.jpg',
),
)),
child: Scaffold(
backgroundColor: Colors.transparent,
body: Center(
child: Card(
elevation: 10,
color: Colors.white,
child: Container(
width: 300,
height: 300,
alignment: Alignment.center,
child: const Text('www.kindacode.com',
style: TextStyle(fontSize: 24)),
),
),
)),
);
}
}
// Kindacode.com
Output:
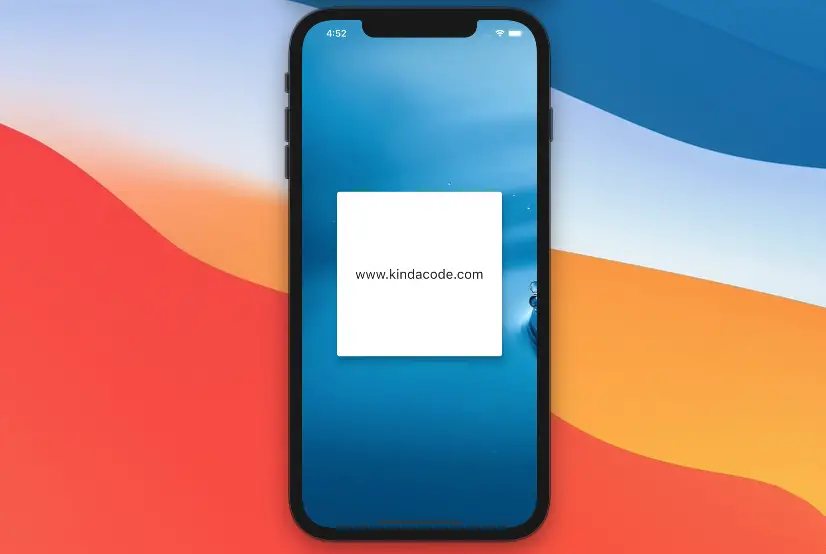
Repeating a small Pattern Image
Here’s the image used in this example:
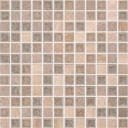
To use a local image, you have to add it to your project. We’ll download the above square image to a folder named images (create this folder if you don’t have it yet):

Then declare it in the flutter section of the pubspec.yaml file:
flutter:
assets:
- images/square.jpeg
Now everything is ready, let’s jump into the code.
The full code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Container(
decoration: const BoxDecoration(
image: DecorationImage(
repeat: ImageRepeat.repeat,
image: AssetImage('images/square.jpeg'),
)),
child: Scaffold(
backgroundColor: Colors.transparent,
body: Center(
child: Card(
elevation: 10,
color: Colors.white,
child: Container(
width: 300,
height: 300,
alignment: Alignment.center,
child: const Text('www.kindacode.com',
style: TextStyle(fontSize: 24)),
),
),
)),
);
}
}
Screenshot:

Conclusion
In this article, we’ve explored how to set an image background for the whole screen in Flutter. If you would like to learn more about Flutter, see the following articles:
- 3 Ways to Create Random Colors in Flutter
- How to make an image carousel in Flutter
- How to create a gradient background AppBar in Flutter
- Make a “Scroll Back To Top” button in Flutter
- How to Create a Countdown Timer in Flutter
- Flutter Stream.periodic: Tutorial & Example
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.