There may be times you want to create random colors to make your Flutter application more vivid, and colorful and give users a new experience, such as you want to create a ListView or GridView with different colored items, you want the background color of an entire screen or a widget not to be tied to a fixed color.
This article will walk through 3 different approaches to generating random colors in Flutter. The first 2 ones only use self-written code and the third one uses a third-party package.
Using Colors.primaries and Random()
The Colors class provides a Material design’s color palette. Currently, it has 18 color swatches.
The Random class comes with a method name nextInt that can generate a random integer in the range from 0, inclusive, to a given max value, exclusive.
You can make a random color like this:
Color _randomColor = Colors.primaries[Random().nextInt(Colors.primaries.length)];
If you need more randomness, just do like this:
Color _randomColor = Colors.primaries[Random().nextInt(Colors.primaries.length)][Random.nextInt(9) * 100];
Don’t forget to import package:flutter/material.dart and dart:math.
Example
This example shows a ListView with random color items.
Preview:

Note that the background colors of the items in the list will change each time you reload the app (thanks to the randomness).
The full code:
// main.dart
import 'package:flutter/material.dart';
import 'dart:math';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
HomePage({Key? key}) : super(key: key);
// This holds the items of the ListView
final _listItems = List.generate(200, (i) => "Item $i");
// Used to generate random integers
final _random = Random();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: ListView.builder(
itemCount: _listItems.length,
itemBuilder: (_, index) {
return Card(
margin: const EdgeInsets.symmetric(vertical: 15, horizontal: 20),
color: Colors.primaries[_random.nextInt(Colors.primaries.length)]
[_random.nextInt(9) * 100],
child: Container(
padding: const EdgeInsets.all(30),
child: Text(
_listItems[index],
style: const TextStyle(fontSize: 24),
),
),
);
},
));
}
}
// By Kindacode.com
Using the Color class and the Random class
The built-in Colors class of Flutter gives us beautiful, standard colors but its limitation is that the number of colors is not too many. By using the Color (without an “s”) class, we are able to generate millions of random colors like this:
final _random = Random();
/* ....... */
// Using Color.fromARGB
final _randomColor = Color.fromARGB(
_random.nextInt(256),
_random.nextInt(256),
_random.nextInt(256),
_random.nextInt(256)
);
// Using Color.fromRGBO
final _anotherRandomColor = Color.fromRGBO(
_random.nextInt(256),
_random.nextInt(256),
_random.nextInt(256),
_random.nextDouble()
);
Example
This example displays a GridView with one thousand of random color items.
Screenshot:
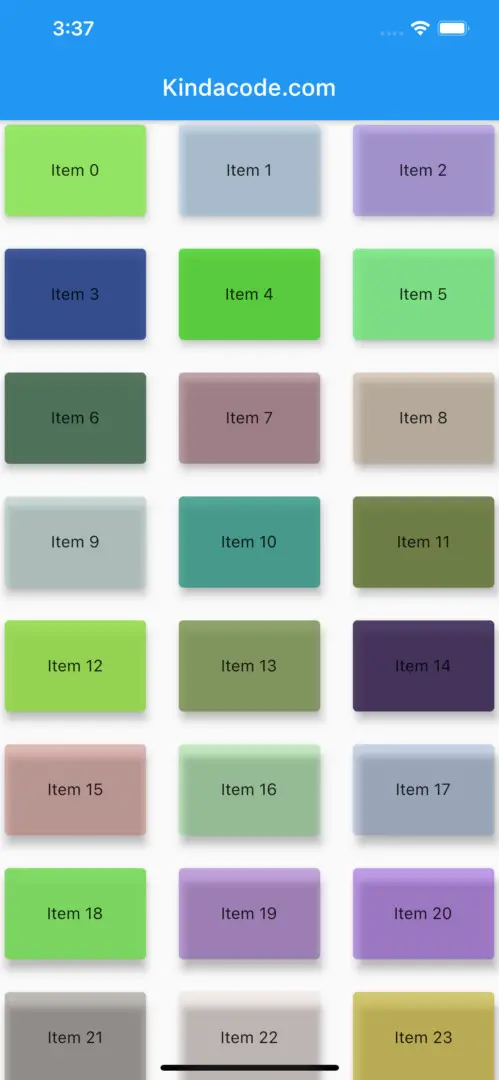
The full code:
// main.dart
import 'package:flutter/material.dart';
import 'dart:math';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
HomePage({Key? key}) : super(key: key);
final _random = Random();
final List _gridItems = List.generate(1000, (i) => "Item $i");
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: GridView.builder(
itemCount: _gridItems.length,
gridDelegate: const SliverGridDelegateWithMaxCrossAxisExtent(
maxCrossAxisExtent: 150,
childAspectRatio: 3 / 2,
crossAxisSpacing: 20,
mainAxisSpacing: 20),
itemBuilder: (_, index) {
return Card(
elevation: 6,
color: Color.fromARGB(_random.nextInt(256), _random.nextInt(256),
_random.nextInt(256), _random.nextInt(256)),
child: Container(
alignment: Alignment.center, child: Text(_gridItems[index])),
);
},
));
}
}
// By Kindacode.com
Using a third-party package
In general, we can make random colors ourselves with only a few lines of code so there’s no need to use a third-party plugin. However, using plugins also has a few advantages. For example, a package name random_color generates random colors that are visually pleasing and can be customized by passing custom hue, saturation, and brightness range. This allows it to generate just specific colors with slight differences.
Installation:
dependencies:
random_color: ^1.0.5
Simple usage
import 'package:random_color/random_color.dart';
RandomColor _randomColor = RandomColor();
Color _color = _randomColor.randomColor(colorHue: ColorHue.red);
This code only returns random red colors:

Conclusion
We have learned some methods to generate random colors in Flutter with and without third-party packages. In many situations, random colors bring users an enjoyable experience and pleasant. However, you also need to be careful when using them because in some cases they can cause bad effects such as making the user dizzy and uncomfortable.
To explore more about Flutter, you can read also the following articles:
- Flutter AnimatedList – Tutorial and Examples
- Scrolling to the desired Item in a ListView
- Flutter: Global, Unique, Value, Object, and PageStorage Keys
- Flutter: Get the Width & Height of a Network Image
- How to Create a Countdown Timer in Flutter
- How to Create a Stopwatch in Flutter
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.