This article shows you how to use the loadingBuilder property when working with Image.network() in Flutter.
Overview
When an image takes too much time to load (caused by a bad internet connection, big file size, etc.), you can display something like a placeholder. This placeholder can be widgets, an indicator, a GIF, a spinning icon, or some text.
You implement a placeholder by using the loadingBuilder parameter of the Image widget like this:
Image.network(
/* Other code */
loadingBuilder: (context, child, loadingProgress) {
if (loadingProgress == null) return child;
return const Center(child: Text('Loading...'));
// You can use LinearProgressIndicator, CircularProgressIndicator, or a GIF instead
},
),
For more clarity, please see the example below.
Example
Preview
The slower your internet speed, the more obvious the loading process will be. If you don’t know how to config the internet speed of your Android emulator, see this article: How to slow down internet speed on Android Emulator.
Here’s how our sample app works on Android:
And here’s how it works on iOS (it happens so fast that the word “loading” only appears for a brief moment):
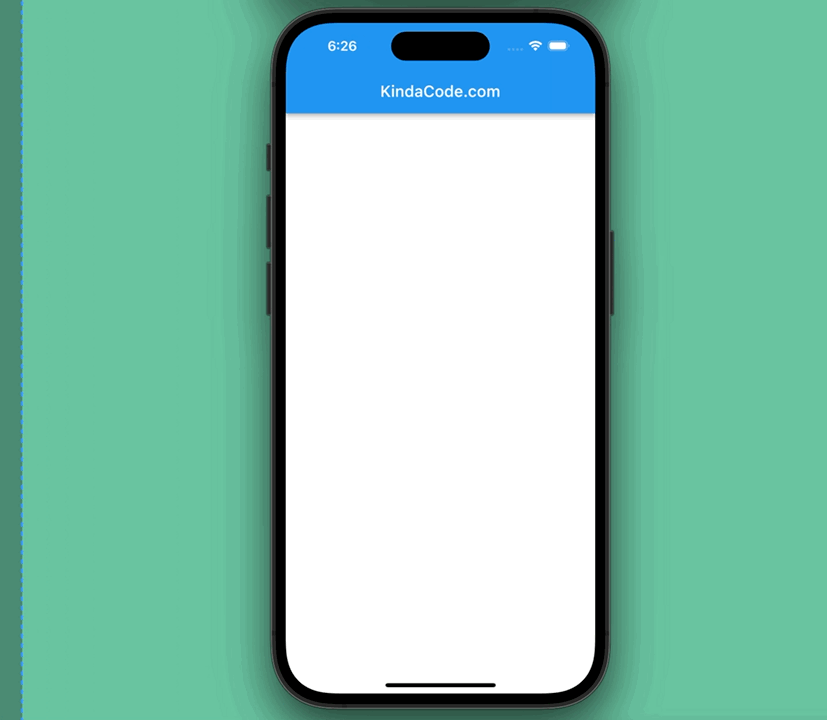
The Code
The minimal code (without boilerplate):
Container(
width: double.infinity,
height: 280,
alignment: Alignment.center,
child: Image.network(
'https://www.kindacode.com/wp-content/uploads/2020/10/sample.jpg',
fit: BoxFit.cover,
loadingBuilder: (context, child, loadingProgress) {
if (loadingProgress == null) return child;
return const Center(child: Text('Loading...'));
// You can use LinearProgressIndicator or CircularProgressIndicator instead
},
errorBuilder: (context, error, stackTrace) =>
const Text('Some errors occurred!'),
),
),
The complete source code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('KindaCode.com'),
),
body: Container(
width: double.infinity,
height: 280,
alignment: Alignment.center,
child: Image.network(
'https://www.kindacode.com/wp-content/uploads/2020/10/sample.jpg',
fit: BoxFit.cover,
loadingBuilder: (context, child, loadingProgress) {
if (loadingProgress == null) return child;
return const Center(child: Text('Loading...'));
// You can use LinearProgressIndicator or CircularProgressIndicator instead
},
errorBuilder: (context, error, stackTrace) =>
const Text('Some errors occurred!'),
),
),
);
}
}
Alternative
To save a few lines of code, you can use the FadeInImage widget:
FadeInImage.assetNetwork(
placeholder: 'assets/images/loading.gif',
image: 'https://www.kindacode.com/wp-content/uploads/2020/10/sample.jpg',
)
Note: Although using FadeInImage.assetNetwork() is easy and quick, it is less customizable than using Image.network().
Don’t forget to add assets/images/loading.gif (the placeholder image) to your project before running it.
Conclusion
You’ve learned how to display a placeholder (text or a GIF image) when an image is loading. If you’d like to explore more about image stuff and other interesting things in Flutter, take a look at the following articles:
- Flutter: Caching Network Images for Big Performance gains
- Flutter: Reading Bytes from a Network Image
- Flutter: Set an image Background for the entire screen
- Flutter: Display Text over Image without using Stack widget
- Best Libraries for Making HTTP Requests in Flutter
- Using BlockSemantics in Flutter: Tutorial & Example
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.