
This article shows you a couple of different ways to automatically change text color based on the brightness of background color.
What Is The Point?
Flutter provides a few methods that can help us get the job done.
1. The computeLuminance() method from the Color class.
You can use this method to programmatically compute the background luminance and then determine whether the text color should be white or black, like this:
Text(
'Hello',
style: TextStyle(
fontSize: 80,
color: _backgroundColor.computeLuminance() > 0.5
? Colors.black
: Colors.white),
),
2. The estimateBrightnessForColor() method from the ThemeData class.
Text(
'Hello',
style: TextStyle(
fontSize: 80,
color: ThemeData.estimateBrightnessForColor(_bgColor) ==
Brightness.light
? Colors.black
: Colors.white),
),
For more clarity, see the demo app below.
The Complete Example
App Preview
The app we are going to make has a box with text inside and 4 buttons. When the user presses a button, the background color of the box will change according to the color of that button. The color of the text will also change automatically to white or black depending on the background color.
Here’s how it works:
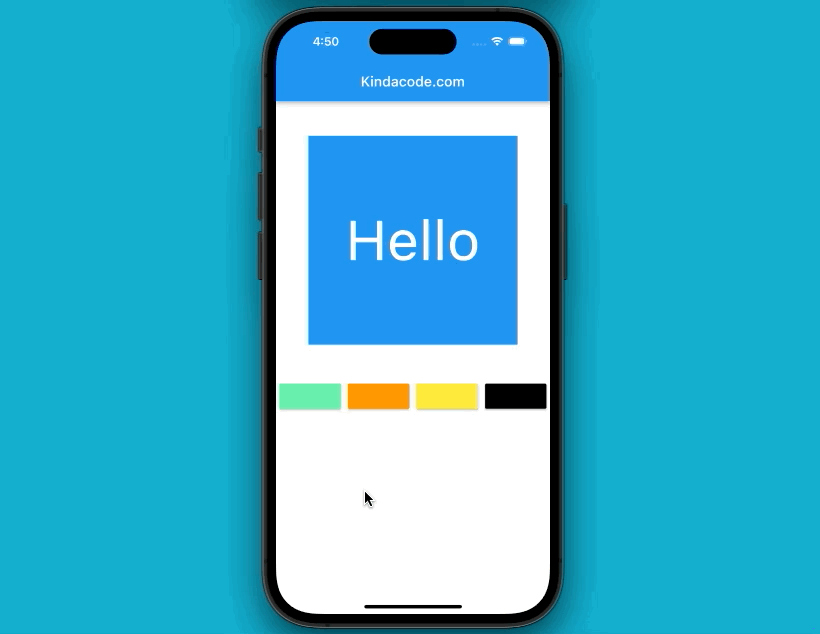
The Complete Code
Here’s the code with explanations:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// Background color of the box
// In the beginning, it is blue
Color _bgColor = Colors.blue;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Column(
children: [
const SizedBox(
height: 50,
),
Container(
width: 300,
height: 300,
color: _bgColor,
child: Center(
child: Text(
'Hello',
style: TextStyle(
fontSize: 80,
color: _bgColor.computeLuminance() > 0.5
? Colors.black
: Colors.white),
),
),
),
const SizedBox(
height: 50,
),
// The buttons below used to change the box color
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
// Green Acent
MaterialButton(
onPressed: () {
setState(() {
_bgColor = Colors.greenAccent;
});
},
color: Colors.greenAccent,
),
// Orange
MaterialButton(
onPressed: () {
setState(() {
_bgColor = Colors.orange;
});
},
color: Colors.orange,
),
// Yellow
MaterialButton(
onPressed: () {
setState(() {
_bgColor = Colors.yellow;
});
},
color: Colors.yellow,
),
// Black
MaterialButton(
onPressed: () {
setState(() {
_bgColor = Colors.black;
});
},
color: Colors.black,
)
],
)
],
),
);
}
}
Conclusion
You’ve learned how to programmatically change text color based on the brightness of its background color. Continue exploring more about color stuff and other interesting things in Flutter by taking a look at the following articles:
- Flutter: Save and Retrieve Colors from Database or File
- Flutter: Make a simple Color Picker from scratch
- Using GetX (Get) for State Management in Flutter
- Flutter + Firebase Storage: Upload, Retrieve, and Delete files
- Flutter and Firestore Database: CRUD example
You can also check out our Flutter category page, or Dart category page for the latest tutorials and examples.