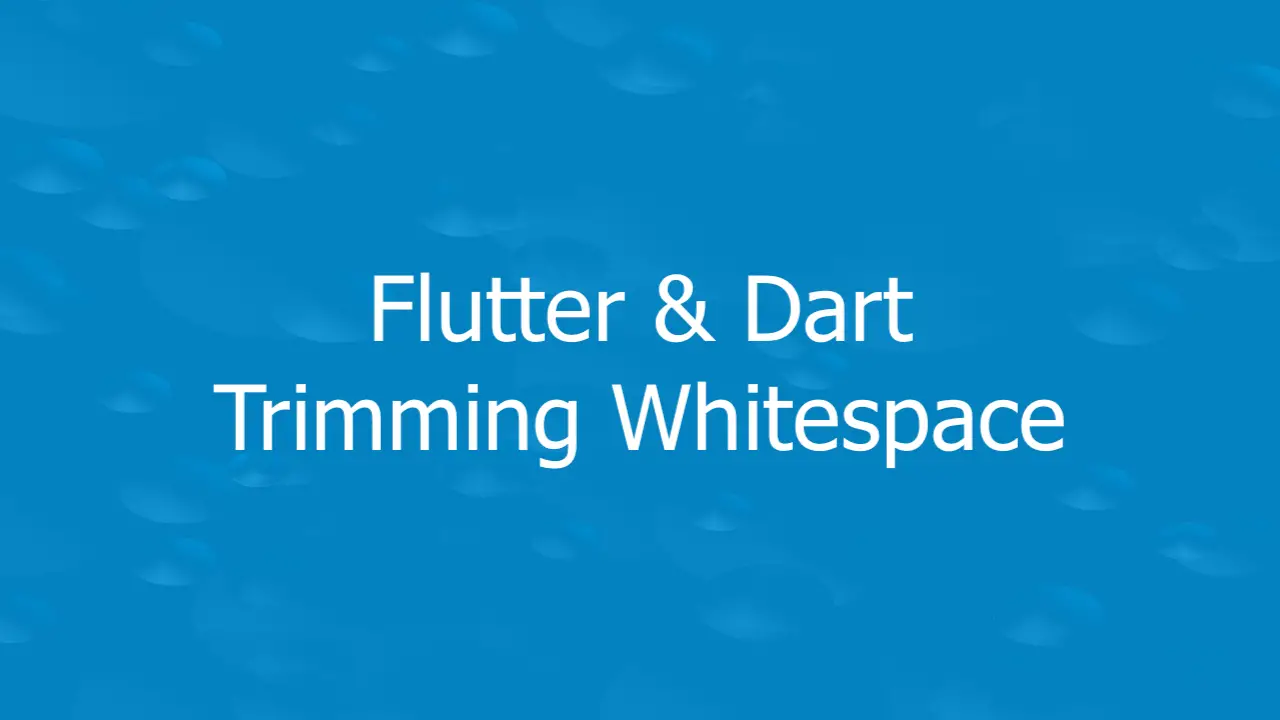
When using your app, users may unintentionally include leading or trailing whitespace when inputting data, which can result in inconsistent data. Trimming the whitespace ensures that the data is consistent and easier to work with. In addition, it’s important to ensure that the input is in the expected format. If leading or trailing whitespace is present, it can cause issues with validation and result in unexpected errors.
This concise article shows you how to trim the leading and trailing whitespace of a string in Dart and Flutter.
Using the trim() method
All spaces before and after your string will be removed.
Example:
void main() {
String input = " Welcome to Kindacode.com ";
String trimmedInput = input.trim();
print(trimmedInput);
print(
"The length has been reduced from ${input.length} to ${trimmedInput.length}");
}
Output:
Welcome to Kindacode.com
The length has been reduced from 29 to 24
Using the trimLeft() method
Sometimes, you may only want to remove the leading whitespace from a string while keeping any trailing whitespace intact. If this is the case, you can use the trimLeft() method.
Example:
void main() {
String input = " Welcome to Kindacode.com ";
String trimmedInput = input.trimLeft();
print(trimmedInput);
print(
"The length has been reduced from ${input.length} to ${trimmedInput.length}");
}
Output:
Welcome to Kindacode.com
The length has been reduced from 29 to 27
Using the trimRight() method
Similar to the example in the previous section, but in the opposite direction, if you only want to remove trailing whitespace from a string but leave any leading whitespace intact, just use the trimRight() method.
Example:
void main() {
String input = " Welcome to Kindacode.com ";
String trimmedInput = input.trimRight();
print(trimmedInput);
print(
"The length has been reduced from ${input.length} to ${trimmedInput.length}");
}
Output:
Welcome to Kindacode.com
flutter: The length has been reduced from 29 to 26
Afterword
If you have something you don’t understand or find mistakes in the examples above, please leave a comment. We will appreciate and welcome them.
If you want to strengthen and improve your skills in Flutter and Dart, take a look at the following articles:
- Flutter: Creating a Custom Number Stepper Input
- Dart: How to Remove or Replace Substrings in a String
- How to Create a Countdown Timer in Flutter
- How to Create a Sortable ListView in Flutter
- 2 Ways to Create Typewriter Effects in Flutter
- How to Check if a Variable is Null in Flutter and Dart
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.