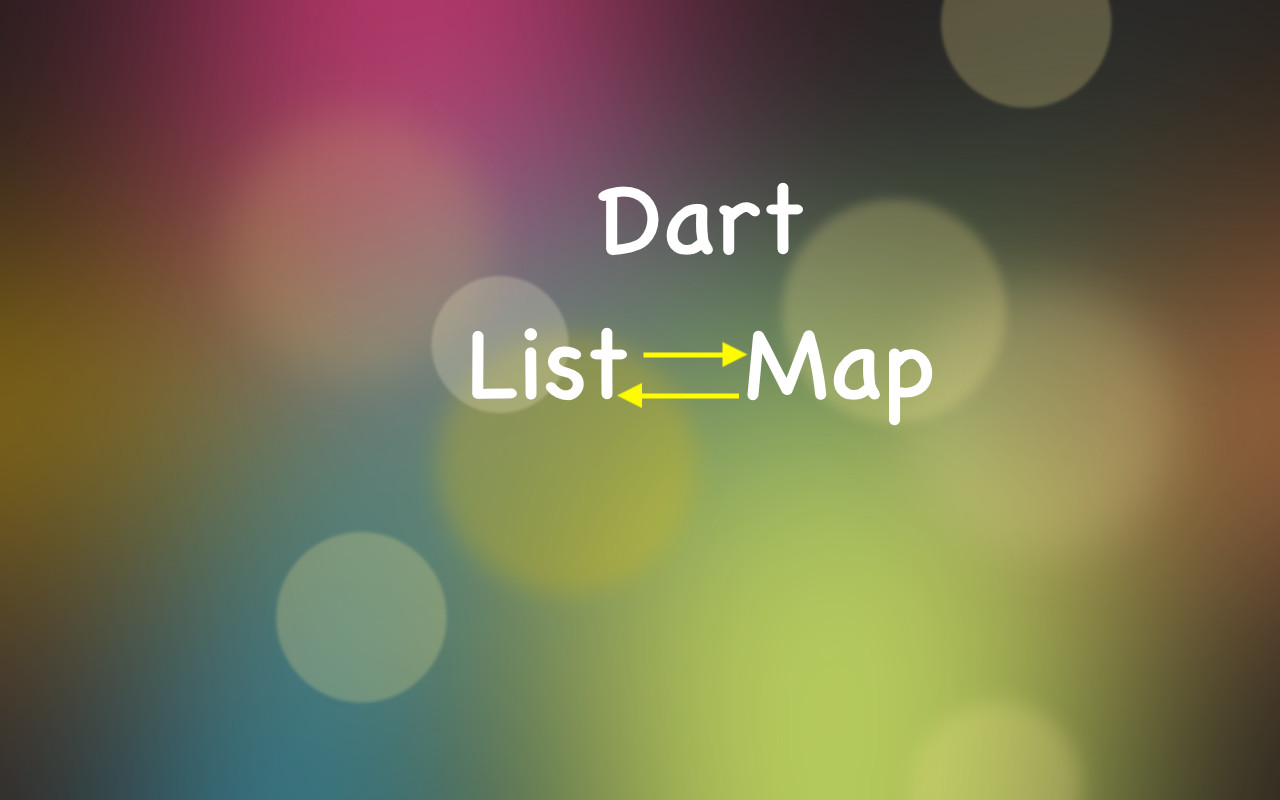
In this article, we will go over a couple of different ways to convert a list to a map as well as turn a map into a list in Dart. Without any further ado, let’s dive right in.
Turning Lists into Maps
Using List.asMap() method
This method will return an immutable map from a given list. The returned map uses the indexes of the list as keys and the corresponding objects as values.
Example:
// kindacode.com
import 'package:flutter/foundation.dart';
void main() {
List list = ['orange', 'banana', 'apple', 'papaya'];
Map<int, dynamic> map = list.asMap();
if (kDebugMode) {
print(map);
print(map[0]);
print(map[1]);
}
}
Output:
{0: orange, 1: banana, 2: apple, 3: papaya}
orange
banana
It’s super important to know that If you try to modify the map, an error will occur:
Unhandled Exception: Unsupported operation: Cannot modify unmodifiable map
If you need to update the map without errors, see the following approaches.
Using a For loop or the List.forEach() method
This approach is much more flexible than the above one. You can decide what the keys and values of the map look like.
Example
Let’s say we have a list of users, and we want to create a map from this list. This map will use the ids of the users as the keys.
Using a for loop:
// kindacode.com
import 'package:flutter/foundation.dart';
void main() {
List list = [
{'_id': 'u1', 'name': 'John Doe', 'age': 30},
{'_id': 'u2', 'name': 'Robbin', 'age': 40},
{'_id': 'u3', 'name': 'Tom', 'age': 50}
];
Map<String, dynamic> map = {
for (var item in list)
item['_id']: {'name': item['name'], 'age': item['age']}
};
if (kDebugMode) {
print(map);
}
}
Output:
{
u1: {name: John Doe, age: 30},
u2: {name: Robbin, age: 40},
u3: {name: Tom, age: 50}
}
You can also use the List.forEach method to get the same results, as shown in the code snippet below:
// kindacode.com
import 'package:flutter/foundation.dart';
void main() {
List list = [
{'_id': 'u1', 'name': 'John Doe', 'age': 30},
{'_id': 'u2', 'name': 'Robbin', 'age': 40},
{'_id': 'u3', 'name': 'Tom', 'age': 50}
];
Map<String, dynamic> map = {};
list.forEach((item) {
map[item['_id']] = {'name': item['name'], 'age': item['age']};
});
if (kDebugMode) {
print(map);
}
}
Converting Maps to Lists
Dart supports several tools that can help us get the job done with ease.
Using Map.entries
Example:
// kindacode.com
void main() {
Map<String, dynamic> map = {
'key1': 'value1',
'key2': 'value2',
'key3': 'value3',
'key4': 'value4'
};
List list = []; // This list contains both keys and values
List listValues = []; // This list only keeps values
List listKeys = []; // This list only contains keys
map.entries.map((e) => list.add({e.key: e.value})).toList();
print(list);
map.entries.map((e) => listValues.add(e.value)).toList();
print(listValues);
map.entries.map((e) => listKeys.add(e.key)).toList();
print(listKeys);
}
Output:
[{key1: value1}, {key2: value2}, {key3: value3}, {key4: value4}]
[value1, value2, value3, value4]
[key1, key2, key3, key4]
Using Map.forEach method
Example:
// kindacode.com
void main() {
Map map = {'key1': 'value1', 'key2': 'value2', 'key3': 'value3'};
List list = [];
List keys = [];
List values = [];
map.forEach((key, value) {
// List of keys and values
list.add({key: value});
// List of keys
keys.add(key);
// List of values
values.add(value);
});
print(list);
print(keys);
print(values);
}
Output:
[{key1: value1}, {key2: value2}, {key3: value3}]
[key1, key2, key3]
[value1, value2, value3]
Conclusion
We’ve walked through a few examples of converting a list to a map and a map to a list in Dart. If you’d like to explore more new and interesting features of modern Dart and Flutter, take a look at the following articles:
- Dart: How to Add new Key/Value Pairs to a Map
- Dart: Sorting Entries of a Map by Its Values
- Dart: Calculate the Sum of all Values in a Map
- Dart: Convert Timestamp to DateTime and vice versa
- Dart & Flutter: 2 Ways to Count Words in a String
- Sorting Lists in Dart and Flutter (5 Examples)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.