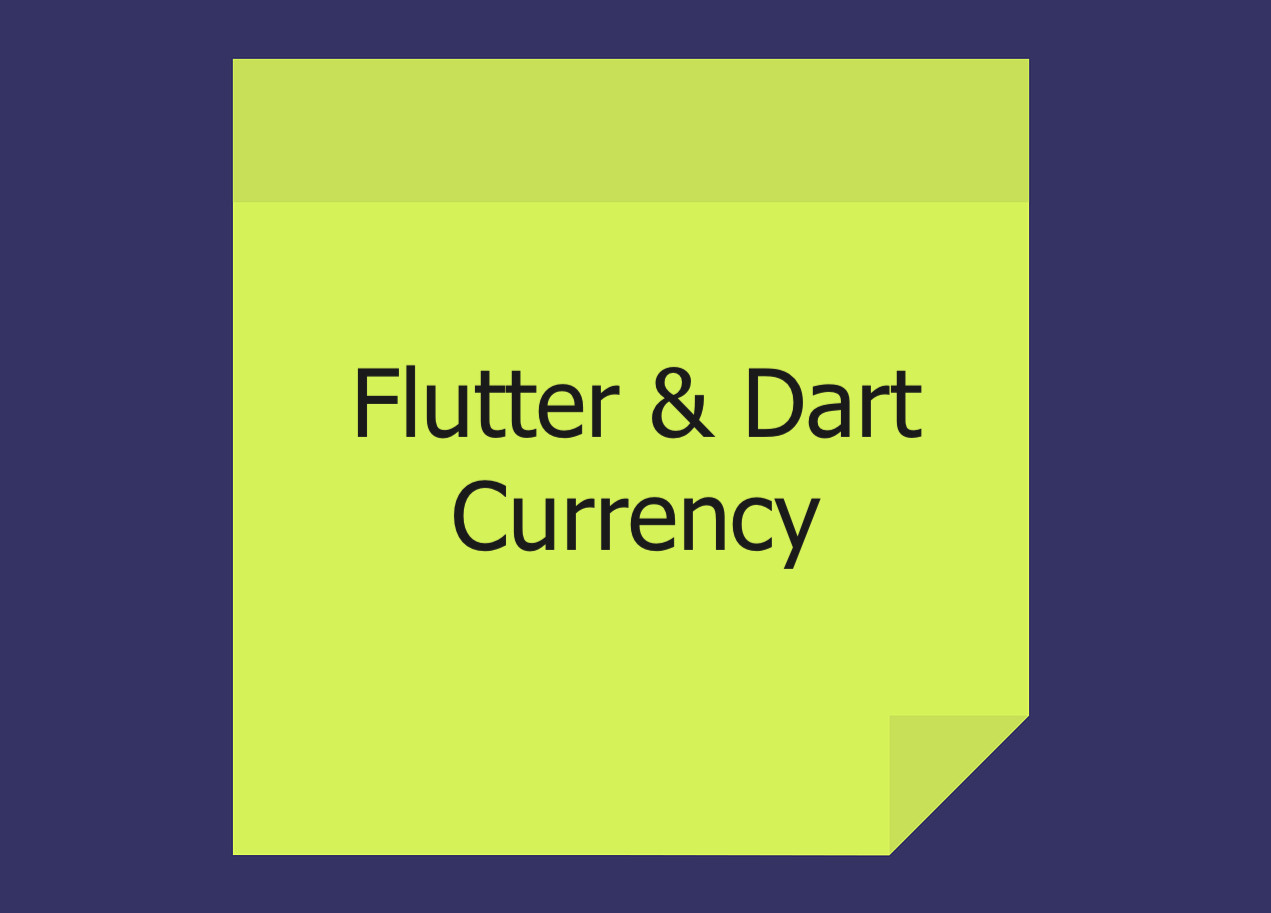
Overview
In order to format numbers as currency strings in Dart and Flutter, we can make use of the NumberFormat
class provided by a well-known, official package named intl. There are several constructors for the job.
If you want compact currency representations (like $9.99M), use this:
NumberFormat.compactCurrency({
String? locale,
String? name,
String? symbol,
int? decimalDigits
})
If you want compact currency representations (like $1k, $3M, etc.), which will automatically determine a currency symbol based on the currency name or the locale, use this:
NumberFormat.compactSimpleCurrency({
String? locale,
String? name,
int? decimalDigits
})
This constructor uses the simple symbol for the currency if one is available ($, €, ¥, ₹, đ, etc.):
NumberFormat.simpleCurrency({
String? locale,
String? name,
int? decimalDigits
})
If you want to get currency representations that use the locale currency pattern, use this:
NumberFormat.currency({
String? locale,
String? name,
String? symbol,
int? decimalDigits,
String? customPattern
})
Let’s examine a couple of examples to get a better understanding of these things.
The First Example
The code:
// kindacode.com
// main.dart
import 'package:flutter/foundation.dart' show kDebugMode;
import 'package:intl/intl.dart';
void main() {
if (kDebugMode) {
// United States
print(NumberFormat.simpleCurrency(locale: 'EN-us', decimalDigits: 2)
.format(99.999));
print(NumberFormat.simpleCurrency(name: 'USD').format(1000));
// United Kingdom
print(NumberFormat.simpleCurrency(locale: 'en-GB').format(1000));
// Japan
print(NumberFormat.simpleCurrency(locale: 'ja-JP', decimalDigits: 2)
.format(10000));
// India
print(NumberFormat.simpleCurrency(locale: 'hi-IN', decimalDigits: 2)
.format(10000));
// China
print(NumberFormat.simpleCurrency(locale: 'zh-CN', decimalDigits: 2)
.format(10000));
// Korea
print(NumberFormat.simpleCurrency(locale: 'ko-KR', decimalDigits: 2)
.format(10000));
// Vietnam
print(NumberFormat.simpleCurrency(locale: 'vi-VN', decimalDigits: 2)
.format(10000));
print(NumberFormat.simpleCurrency(name: 'VND', decimalDigits: 2)
.format(10000));
}
}
Output:
$100.00
$1,000.00
£1,000.00
¥10,000.00
₹10,000.00
¥10,000.00
₩10,000.00
10.000,00 ₫
₫10,000.00
The Second Example
Screenshot:
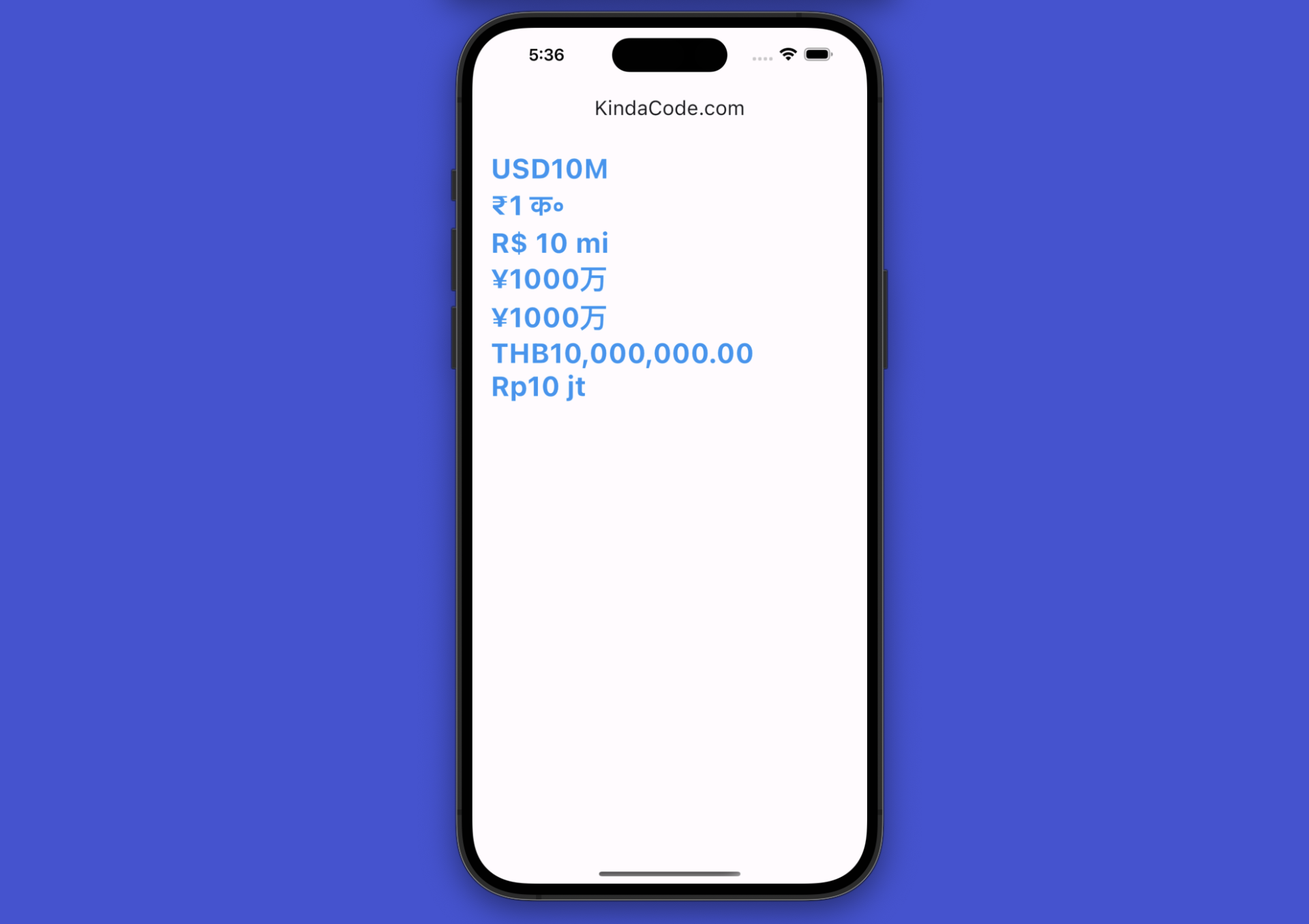
The code:
// main.dart
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(useMaterial3: true),
home: const HomeScreen(),
);
}
}
class HomeScreen extends StatelessWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('KindaCode.com')),
body: Padding(
padding: const EdgeInsets.all(20),
child: DefaultTextStyle(
style: const TextStyle(
fontSize: 30, color: Colors.blue, fontWeight: FontWeight.bold),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
// US
Text(NumberFormat.compactCurrency(locale: 'en-US')
.format(10000000)),
// India
Text(NumberFormat.compactSimpleCurrency(locale: 'hi-IN')
.format(10000000)),
// Brazil
Text(NumberFormat.compactSimpleCurrency(locale: 'pt-BR')
.format(10000000)),
// China Mainland
Text(NumberFormat.compactSimpleCurrency(locale: 'zh-CN')
.format(10000000)),
// Japan
Text(NumberFormat.compactSimpleCurrency(locale: 'ja')
.format(10000000)),
// Thailand
Text(NumberFormat.currency(locale: 'th-TH').format(10000000)),
// Indonesia
Text(NumberFormat.compactSimpleCurrency(locale: 'id-ID')
.format(10000000)),
],
),
),
),
);
}
}
The List of Locales
For your reference, here’s the list of locale codes:
[
"Cy-az-AZ",
"Cy-sr-SP",
"Cy-uz-UZ",
"Lt-az-AZ",
"Lt-sr-SP",
"Lt-uz-UZ",
"aa",
"ab",
"ae",
"af",
"af-ZA",
"ak",
"am",
"an",
"ar",
"ar-AE",
"ar-BH",
"ar-DZ",
"ar-EG",
"ar-IQ",
"ar-JO",
"ar-KW",
"ar-LB",
"ar-LY",
"ar-MA",
"ar-OM",
"ar-QA",
"ar-SA",
"ar-SY",
"ar-TN",
"ar-YE",
"as",
"av",
"ay",
"az",
"ba",
"be",
"be-BY",
"bg",
"bg-BG",
"bh",
"bi",
"bm",
"bn",
"bo",
"br",
"bs",
"ca",
"ca-ES",
"ce",
"ch",
"co",
"cr",
"cs",
"cs-CZ",
"cu",
"cv",
"cy",
"da",
"da-DK",
"de",
"de-AT",
"de-CH",
"de-DE",
"de-LI",
"de-LU",
"div-MV",
"dv",
"dz",
"ee",
"el",
"el-GR",
"en",
"en-AU",
"en-BZ",
"en-CA",
"en-CB",
"en-GB",
"en-IE",
"en-JM",
"en-NZ",
"en-PH",
"en-TT",
"en-US",
"en-ZA",
"en-ZW",
"eo",
"es",
"es-AR",
"es-BO",
"es-CL",
"es-CO",
"es-CR",
"es-DO",
"es-EC",
"es-ES",
"es-GT",
"es-HN",
"es-MX",
"es-NI",
"es-PA",
"es-PE",
"es-PR",
"es-PY",
"es-SV",
"es-UY",
"es-VE",
"et",
"et-EE",
"eu",
"eu-ES",
"fa",
"fa-IR",
"ff",
"fi",
"fi-FI",
"fj",
"fo",
"fo-FO",
"fr",
"fr-BE",
"fr-CA",
"fr-CH",
"fr-FR",
"fr-LU",
"fr-MC",
"fy",
"ga",
"gd",
"gl",
"gl-ES",
"gn",
"gu",
"gu-IN",
"gv",
"ha",
"he",
"he-IL",
"hi",
"hi-IN",
"ho",
"hr",
"hr-HR",
"ht",
"hu",
"hu-HU",
"hy",
"hy-AM",
"hz",
"ia",
"id",
"id-ID",
"ie",
"ig",
"ii",
"ik",
"io",
"is",
"is-IS",
"it",
"it-CH",
"it-IT",
"iu",
"ja",
"ja-JP",
"jv",
"ka",
"ka-GE",
"kg",
"ki",
"kj",
"kk",
"kk-KZ",
"kl",
"km",
"kn",
"kn-IN",
"ko",
"ko-KR",
"kr",
"ks",
"ku",
"kv",
"kw",
"ky",
"ky-KZ",
"la",
"lb",
"lg",
"li",
"ln",
"lo",
"lt",
"lt-LT",
"lu",
"lv",
"lv-LV",
"mg",
"mh",
"mi",
"mk",
"mk-MK",
"ml",
"mn",
"mn-MN",
"mr",
"mr-IN",
"ms",
"ms-BN",
"ms-MY",
"mt",
"my",
"na",
"nb",
"nb-NO",
"nd",
"ne",
"ng",
"nl",
"nl-BE",
"nl-NL",
"nn",
"nn-NO",
"no",
"nr",
"nv",
"ny",
"oc",
"oj",
"om",
"or",
"os",
"pa",
"pa-IN",
"pi",
"pl",
"pl-PL",
"ps",
"pt",
"pt-BR",
"pt-PT",
"qu",
"rm",
"rn",
"ro",
"ro-RO",
"ru",
"ru-RU",
"rw",
"sa",
"sa-IN",
"sc",
"sd",
"se",
"sg",
"si",
"sk",
"sk-SK",
"sl",
"sl-SI",
"sm",
"sn",
"so",
"sq",
"sq-AL",
"sr",
"ss",
"st",
"su",
"sv",
"sv-FI",
"sv-SE",
"sw",
"sw-KE",
"ta",
"ta-IN",
"te",
"te-IN",
"tg",
"th",
"th-TH",
"ti",
"tk",
"tl",
"tn",
"to",
"tr",
"tr-TR",
"ts",
"tt",
"tt-RU",
"tw",
"ty",
"ug",
"uk",
"uk-UA",
"ur",
"ur-PK",
"uz",
"ve",
"vi",
"vi-VN",
"vo",
"wa",
"wo",
"xh",
"yi",
"yo",
"za",
"zh",
"zh-CHS",
"zh-CHT",
"zh-CN",
"zh-HK",
"zh-MO",
"zh-SG",
"zh-TW",
"zu"
]
Afterword
You’ve learned how to display product prices in nice formats with the help of the NumberFormat
class. As of now, you’ll have a handy solution to get through that task. If you’d like to explore more new and exciting stuff in Flutter, take a look at the following articles:
- Flutter PaginatedDataTable Example
- Example of sortable DataTable in Flutter
- Flutter: Making a Dropdown Multiselect with Checkboxes
- Flutter: ValueListenableBuilder Example
- Flutter StreamBuilder examples (null safety)
- Flutter & Hive Database: CRUD Example
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.