The example below shows you how to implement a sortable table in Flutter by using the DataTable widget.
Preview
This sample app contains a DataTable which displays a list of fiction products. In the beginning, the products are sorted in ascending ID order. However, the user can sort them in ascending or descending price order by touching the heading of the price column, as shown in the following screen capture:
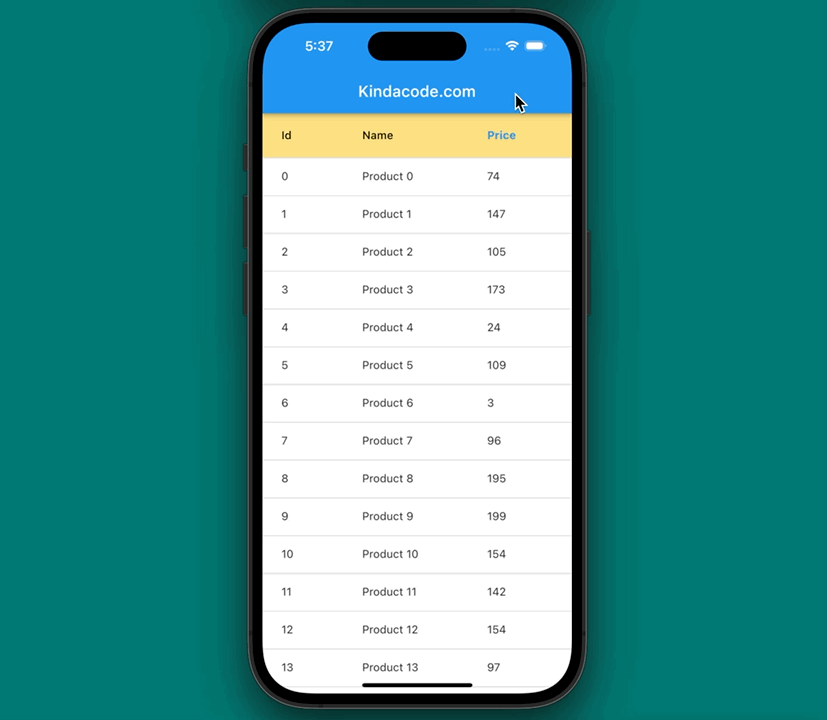
The code
The full code in main.dart with explanations:
import 'package:flutter/material.dart';
import 'dart:math';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
// Generate a list of fiction prodcts
final List<Map> _products = List.generate(30, (i) {
return {"id": i, "name": "Product $i", "price": Random().nextInt(200) + 1};
});
int _currentSortColumn = 0;
bool _isAscending = true;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: SizedBox(
width: double.infinity,
child: SingleChildScrollView(
child: DataTable(
sortColumnIndex: _currentSortColumn,
sortAscending: _isAscending,
headingRowColor: MaterialStateProperty.all(Colors.amber[200]),
columns: [
const DataColumn(label: Text('Id')),
const DataColumn(label: Text('Name')),
DataColumn(
label: const Text(
'Price',
style: TextStyle(
color: Colors.blue, fontWeight: FontWeight.bold),
),
// Sorting function
onSort: (columnIndex, _) {
setState(() {
_currentSortColumn = columnIndex;
if (_isAscending == true) {
_isAscending = false;
// sort the product list in Ascending, order by Price
_products.sort((productA, productB) =>
productB['price'].compareTo(productA['price']));
} else {
_isAscending = true;
// sort the product list in Descending, order by Price
_products.sort((productA, productB) =>
productA['price'].compareTo(productB['price']));
}
});
}),
],
rows: _products.map((item) {
return DataRow(cells: [
DataCell(Text(item['id'].toString())),
DataCell(Text(item['name'])),
DataCell(Text(item['price'].toString()))
]);
}).toList(),
),
),
));
}
}
Hope this example can help you a little. Besides DataTable, Flutter also provides a couple of other widgets to implement tables:
If you would like to learn more about Flutter, take a look at the following articles:
- Ways to Store Data Offline in Flutter
- Flutter SliverAppBar Example (with Explanations)
- 2 ways to remove duplicate items from a list in Dart
- Dart regular expressions to check people’s names
- Flutter & Dart Regular Expression Examples
You can also check out our Flutter topic page or Dart topic page for the latest tutorials and examples.