This article is about the Algin widget in Flutter. We’ll have a glance at the fundamentals of the widget and then examine a couple of examples of implementing it in practice. Without any further bothersome (like talking about the history of Flutter), let’s get to the point.
Overview
The Align widget in Flutter is used to align a child widget within its parent. It allows you to control the positioning of a child widget relative to its parent by specifying the alignment property.
Align({
Key? key,
AlignmentGeometry alignment = Alignment.center,
double? widthFactor,
double? heightFactor,
Widget? child
})
This alignment property takes in an instance of a subclass (e.g., Alignment class) of the AlignmentGeometry class and specifies the position of the child widget within the parent. The default value of the alignment property is Alignment.center, which means the child widget will stay in the center area. You can change this by passing in an instance of Alignment or AlignmentDirectional to the alignment property.
The widthFactor and heightFactor properties set the width and height of the Align widget to child’s width * widthFactor and child’s height * heightFactor, respectively.
Words might be vague and confusing. The examples below will help you see the picture much more clearly.
Examples
Example 1: Align a Box
In this example, we align an amber box to the bottom-right corner of the screen.
Screenshot:

The code:
Scaffold(
appBar: AppBar(title: const Text('Kindacode.com')),
body: Align(
alignment: Alignment.bottomRight,
child: Container(
color: Colors.amber,
width: 150,
height: 150,
),
)
);
Example 2: Align Text
This example displays a Text widget in the center of a Container widget (by using the Align widget).
Screenshot:
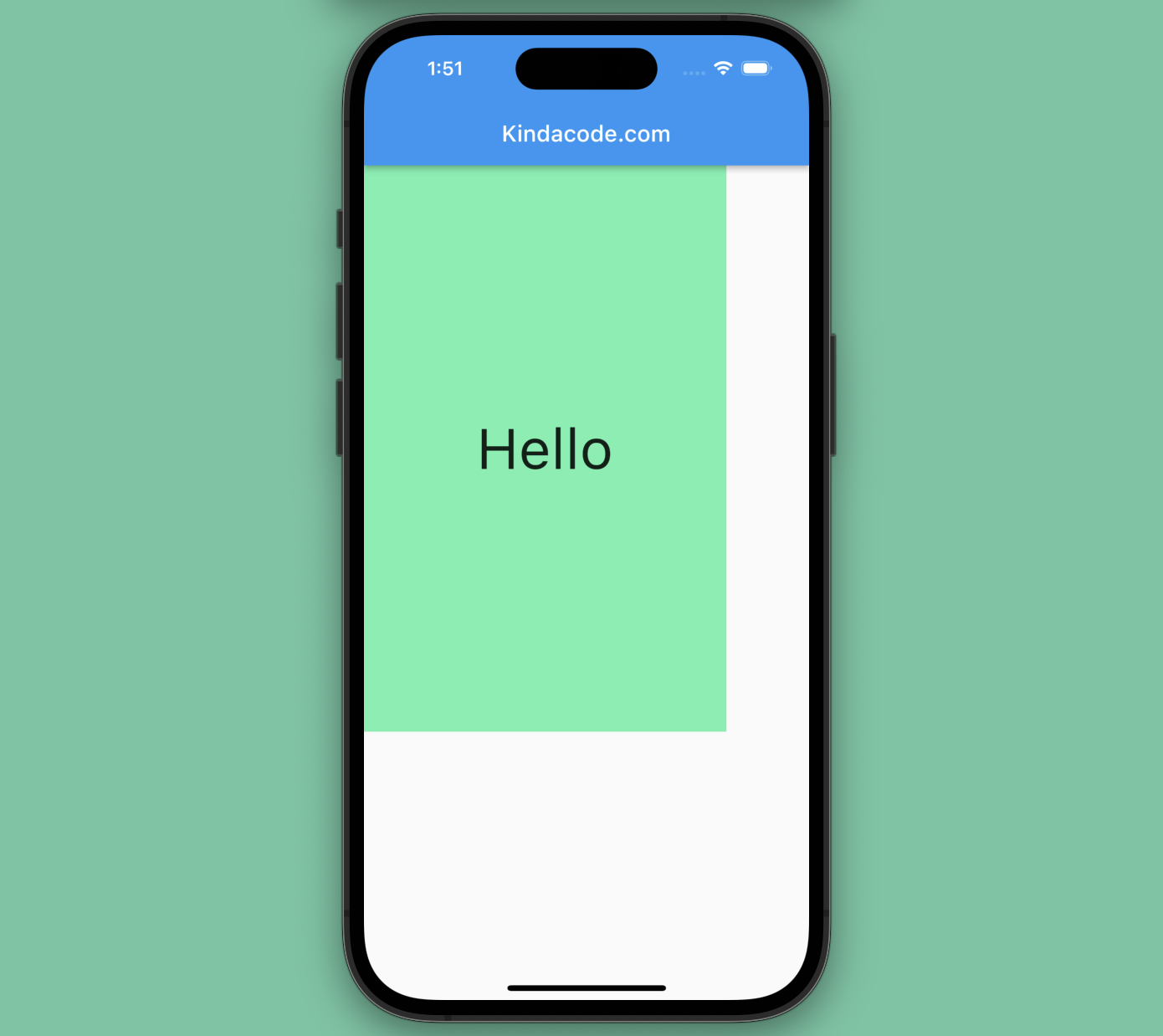
The code:
Scaffold(
appBar: AppBar(title: const Text('Kindacode.com')),
body: Container(
width: 320,
height: 500,
color: Colors.greenAccent,
child: const Align(
alignment: Alignment.center,
widthFactor: 5,
heightFactor: 10,
child: Text(
'Hello',
style: TextStyle(fontSize: 50),
)),
)
);
Example 3: Using Alignment(double x, double y)
You can use Alignment(x, y) to precisely align the child wherever you want (as long as it stays inside the parent). x and y are the distance fractions in the horizontal and vertical directions, respectively. For example, Alignment(0, 0) is equivalent to Alignment.center, Alignment(1, 1) is equivalent to Alignment.bottomRight. In order for the child widget not to be cut off any part, this condition must be satisfied:
-1 <= x <= 1 and -1 <= y <= 1
The example demonstrates how Alignment(1, 0.5) works.
Screenshot:

The code:
Scaffold(
appBar: AppBar(
title: const Text("Kindacode.com"),
),
body: Container(
color: Colors.amber,
width: double.infinity,
height: double.infinity,
child: Align(
alignment: const Alignment(0.5, 0.5),
child: Container(
width: 100,
height: 100,
color: Colors.pink,
)),
),
);
Conclusion
You’ve learned how to use the Align widget to arrange things and build layouts in Flutter. If you’d like to explore more new and interesting stuff about the awesome framework, take a look at the following articles:
- 2 Ways to Create Typewriter Effects in Flutter
- Flutter: Making a Tic Tac Toe Game from Scratch
- Flutter StreamBuilder: Tutorial & Examples (Updated)
- 3 Ways to create Random Colors in Flutter
- Creating Masonry Layout in Flutter with Staggered Grid View
- Flutter AnimatedList – Tutorial and Examples
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.