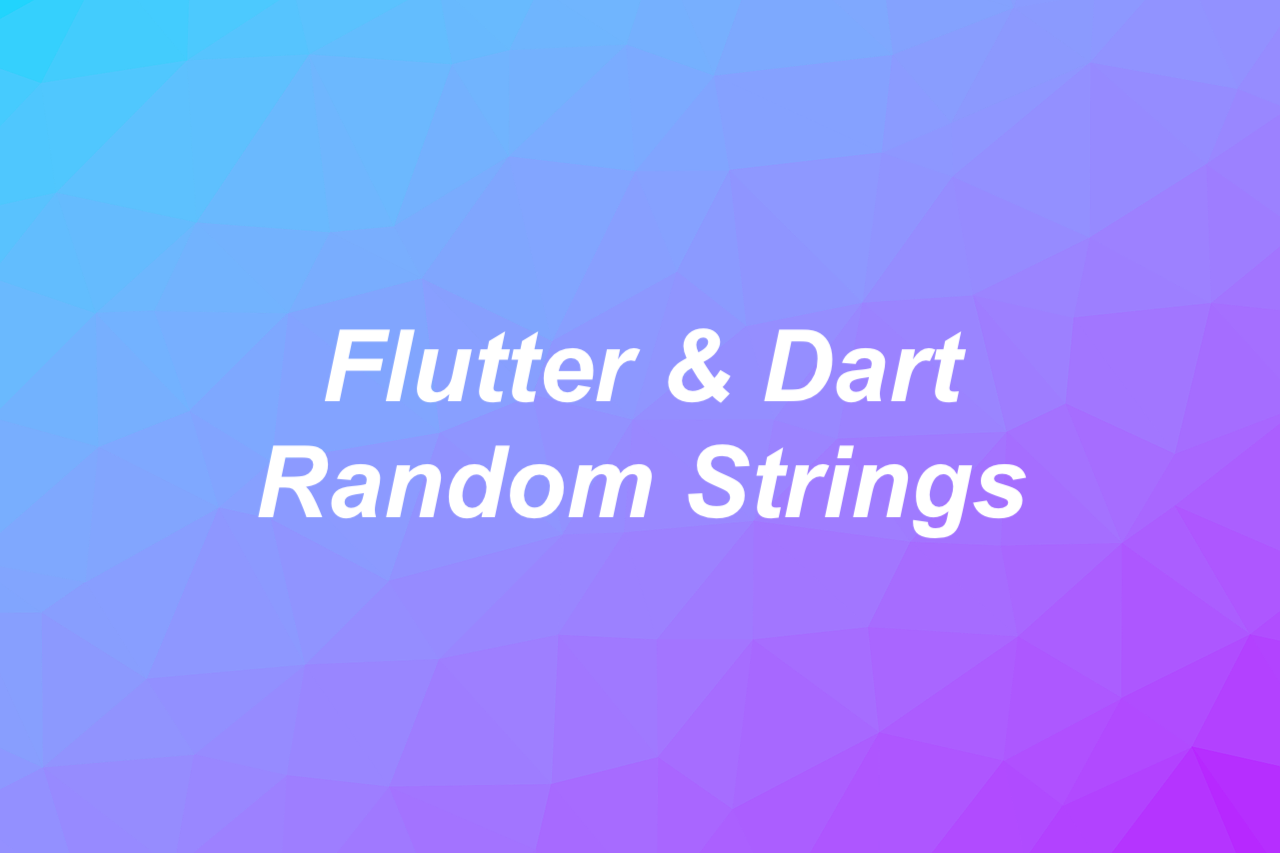
This article shows you 3 different approaches to generating random strings in Dart (and Flutter as well). Without any further ado, let’s dive into the code.
Joining Random Alphabet Letters and Numbers
The example below demonstrates how to produce a random string with a given length. The result will only contain alphabet letters and numbers (a-z, A-Z, 0-9).
// kindacode.com
// main.dart
import 'dart:math';
import 'package:flutter/foundation.dart';
// Define a reusable function
String generateRandomString(int length) {
final random = Random();
const availableChars =
'AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz1234567890';
final randomString = List.generate(length,
(index) => availableChars[random.nextInt(availableChars.length)]).join();
return randomString;
}
void main() {
debugPrint(generateRandomString(10));
debugPrint(generateRandomString(20));
}
The output will be different each time you run the preceding code due to the randomness:
tFKOzF6eOs
WsJ1tF6IyIBfMMXCQS1t
Using the Crypto library
This approach makes use of the crypto package published by the Dart team. The example below will use md5 hashing and sha1 hashing to generate random strings.
Example:
// kindacode.com
// main.dart
import 'package:flutter/foundation.dart';
import 'dart:math';
import 'dart:convert';
import 'package:crypto/crypto.dart';
// md5 hashing a random number
String md5RandomString() {
final randomNumber = Random().nextDouble();
final randomBytes = utf8.encode(randomNumber.toString());
final randomString = md5.convert(randomBytes).toString();
return randomString;
}
// sha1 hashing a random number
String sha1RandomString() {
final randomNumber = Random().nextDouble();
final randomBytes = utf8.encode(randomNumber.toString());
final randomString = sha1.convert(randomBytes).toString();
return randomString;
}
void main() {
debugPrint(md5RandomString());
debugPrint(sha1RandomString());
}
Output:
eb858fc63586fb81123d750fe82e39e0
35911da7d122bffeb75714cdde4c96b55c7fa8ef
Using Character Codes
This example will produce random strings with given lengths. The results will contain special characters.
// kindacode.com
// main.dart
import 'dart:math';
import 'package:flutter/foundation.dart';
// Declare a fucntion for reusable purpose
String generateRandomString(int len) {
final random = Random();
final result = String.fromCharCodes(
List.generate(len, (index) => random.nextInt(33) + 89));
return result;
}
void main() {
debugPrint(generateRandomString(50));
debugPrint(generateRandomString(20));
}
Output:
`[uvj][d\oZvymn]tYes]l]_gydf_uYmacjvgtanlinr_lougc
d__xvvvpclgfjqwqZZs\
Wrapping Up
We’ve walked through a couple of different ways to create random strings in Dart and Flutter programs. If you’d like to explore more new and exciting things about Dart and Flutter, take a look at the following articles:
- Flutter: Create a Password Strength Checker from Scratch
- Flutter: Drawing an N-Pointed Star with CustomClipper
- Flutter: Create a Button with a Loading Indicator Inside
- 2 Ways to Create Flipping Card Animation in Flutter
- Dart: Convert Map to Query String and vice versa
- Using Static Methods in Dart and Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.