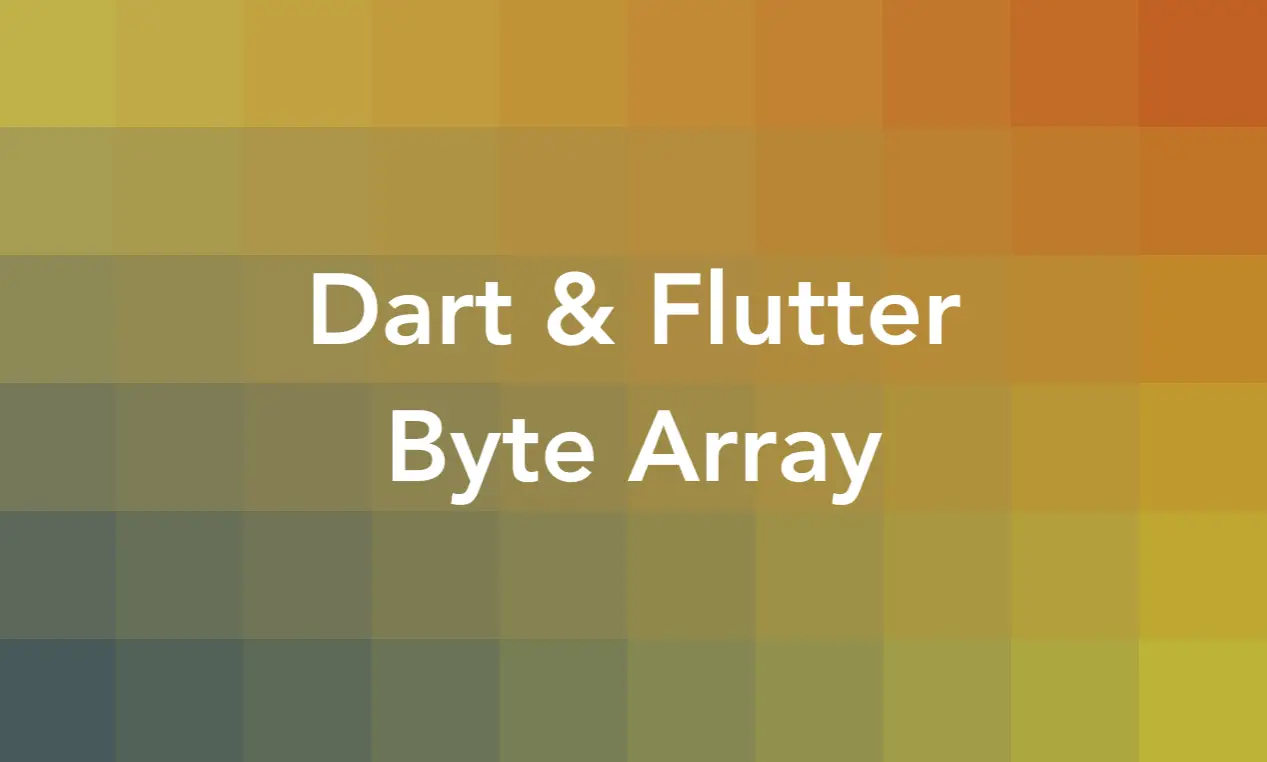
This concise article shows you how to convert a string or a number (integer or double) to a byte array (a list of bytes) in Dart (and Flutter as well).
Converting a String into a Byte Array
There is more than one way to turn a given string into a byte array in Dart.
Using the utf8.encode() method
The utf8.encode() method (which can be imported from dart:convert) takes a string and return a list of UTF-8 bytes.
Example:
// main.dart
import 'dart:convert';
void main() {
String text = 'KindaCode.com';
List<int> bytes = utf8.encode(text);
print(bytes);
}
Output:
[75, 105, 110, 100, 97, 67, 111, 100, 101, 46, 99, 111, 109]
Using the codeUnits property of the String class
The codeUnits property of the String class returns a list of UTF-16 code units.
Example:
// main.dart
void main() {
String text = 'KindaCode.com';
List<int> bytes = text.codeUnits;
print(bytes);
}
Output:
75, 105, 110, 100, 97, 67, 111, 100, 101, 46, 99, 111, 109]
Using the runes property of the String class
The runes property gives you an iterable of Unicode code points of a string.
Example:
// main.dart
void main() {
String text = 'Welcome to KindaCode.com';
Iterable<int> bytes = text.runes;
// convert the iterable to a list
List<int> list = bytes.toList();
print(list);
}
Output:
[87, 101, 108, 99, 111, 109, 101, 32, 116, 111, 32, 75, 105, 110, 100, 97, 67, 111, 100, 101, 46, 99, 111, 109]
Converting a Number into a Byte Array
To convert a number to a byte array, you can first convert it to a string and then use one of the approaches mentioned in the previous section to get the job done.
Example:
// main.dart
import "dart:convert";
void main() {
// integer to byte array
int myInt = 2023;
print(utf8.encode(myInt.toString()));
// double to byte array
double myDouble = 3.1416;
print(utf8.encode(myDouble.toString()));
}
Output:
[50, 48, 50, 51]
[51, 46, 49, 52, 49, 54]
That’s it. Further reading:
- Flutter & Dart: Convert a String/Integer to Hex
- Flutter & Dart: Convert Strings to Binary
- How to Create a Stopwatch in Flutter
- Flutter & SQLite: CRUD Example
- Using Provider for State Management in Flutter
- Flutter: Turn an Image into a Base64 String and Vice Versa
You can also tour around our Flutter topic page or Dart topic page for the most recent tutorials and examples.