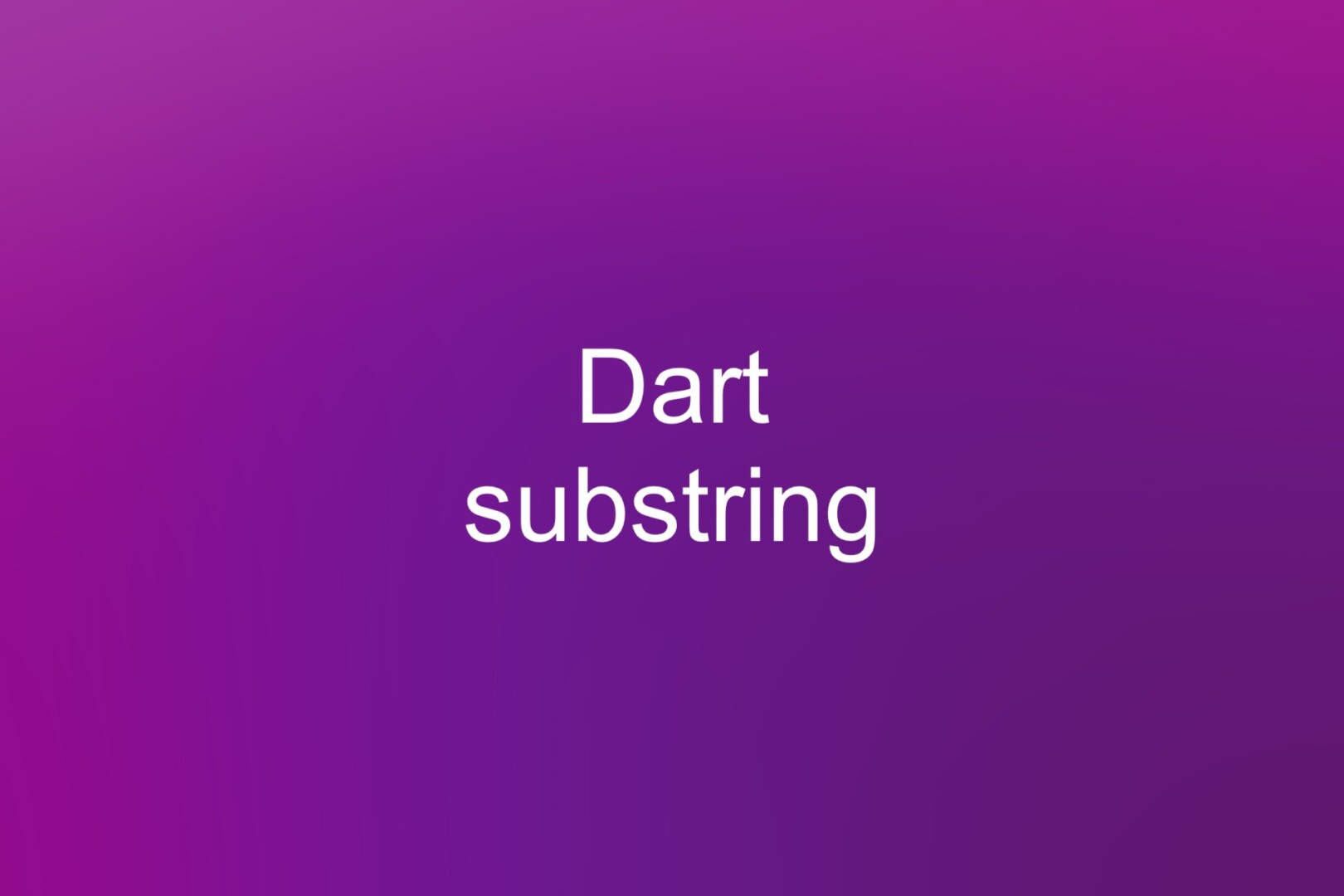
This article is about extracting a substring from a given string in Dart (and Flutter as well). We’ll cover the fundamentals, write a simple program, then walks through a few advanced use cases. Without any further ado, let’s dive right in.
Overview
The substring method returns a substring from the start index (inclusive) to the end index (exclusive):
String? result = parentString.substring([start index], [end index]);
The original string will not be changed. The result can be a string or null. The start index is required while the end index is optional.
If we know the start and end indices, things are easy. However, in real-world applications, it’s a little bit challenging since we have to calculate the indices ourselves most of the time.
Examples
Simple Example
This program will extract a substring with a given length from the parent string. The starting point is the first character. This scenario is quite common when you only want to display part of long content to save space.
The code:
// main.dart
void main() {
const String content =
'He thrusts his fists against the posts and still insists he sees the ghosts.';
const int length = 10;
final excerpt = content.substring(0, length);
print(excerpt);
}
Output:
He thrusts
The following examples are more complicated than this one.
Extracting URLs from a string
In this example, we will get all URLs from text by using regular expressions.
The code:
// main.dart
void main() {
const dummyString =
"Welcome to https://www.kindacode.com and learn new things. Contact us at https://www.kindacode.com/contact or via email. Happy coding and have a nice day!";
final RegExp exp = RegExp(r'(?:(?:https?):\/\/)?[\w/\-?=%.]+\.[\w/\-?=%.]+');
List<RegExpMatch> matches = exp.allMatches(dummyString).toList();
for (var match in matches) {
print(dummyString.substring(match.start, match.end));
}
}
Output:
https://www.kindacode.com
https://www.kindacode.com/contact
See also: Flutter & Dart: Regular Expression Examples
Getting a substring between two words
Sometimes you need to extract a substring from an existing string provided that the other substring must be between two given words.
The code:
// main.dart
void main() {
const input = "One two three four five six seven eight.";
const startWord = "two";
const endWord = "six";
final startIndex = input.indexOf(startWord);
final endIndex = input.indexOf(endWord, startIndex + startWord.length);
final String? output =
input.substring(startIndex + startWord.length, endIndex);
print(output);
}
Output:
three four five
Conclusion
We’ve gone through a few examples of making use of the substring method in Dart. If you’d like to explore more new and amazing things about modern mobile development with Flutter, take a look at the following articles:
- How to create a gradient background AppBar in Flutter
- Flutter: SliverGrid example
- Flutter and Firestore Database: CRUD example
- How to implement Star Rating in Flutter
- How to make an image carousel in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.