This article walks you through a couple of examples of adding a search field to an app bar in Flutter. The first one uses the AppBar widget (most seen in entertainment apps) and the second one uses the SliverAppBar widget (commonly used in e-commerce apps). Without any further ado, let’s dive right in.
Example 1: Search Field inside AppBar
In general, many entertainment apps (including big ones like Facebook, Youtube, Spotify, etc) don’t show the search field by default but show a search icon button. When this button is pressed, the search field will be somehow displayed.
Preview
The demo app we are going to make contains 2 screens (pages): HomePage and SearchPage. The user can move from the HomePage to the SearchPage by tapping the search icon button. The search field will be implemented by using the title argument of the SearchPage’s AppBar.
Let’s see how it works in action:
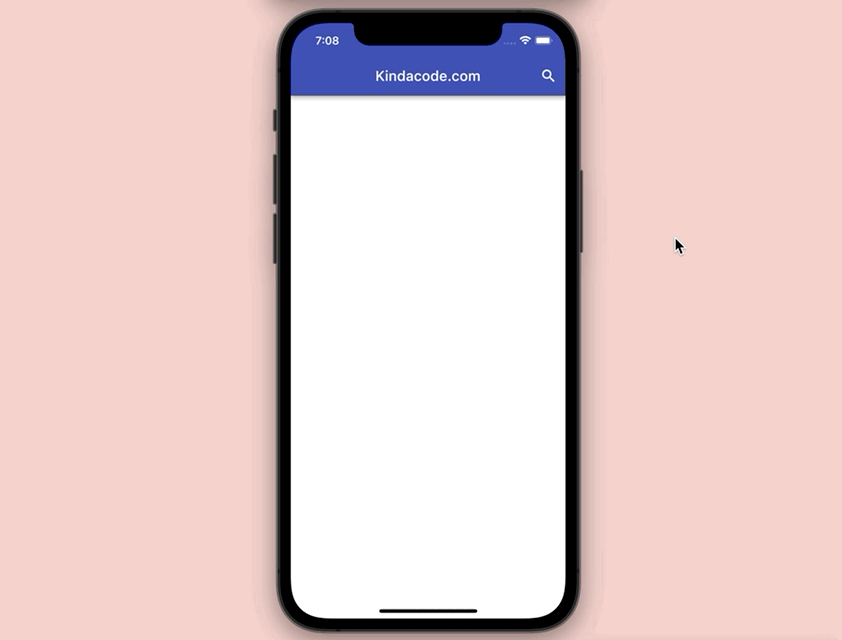
The Code
The complete source code in ./lib/main.dart with explanations:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const HomePage());
}
}
// Home Page
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
actions: [
// Navigate to the Search Screen
IconButton(
onPressed: () => Navigator.of(context)
.push(MaterialPageRoute(builder: (_) => const SearchPage())),
icon: const Icon(Icons.search))
],
),
);
}
}
// Search Page
class SearchPage extends StatelessWidget {
const SearchPage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
// The search area here
title: Container(
width: double.infinity,
height: 40,
decoration: BoxDecoration(
color: Colors.white, borderRadius: BorderRadius.circular(5)),
child: Center(
child: TextField(
decoration: InputDecoration(
prefixIcon: const Icon(Icons.search),
suffixIcon: IconButton(
icon: const Icon(Icons.clear),
onPressed: () {
/* Clear the search field */
},
),
hintText: 'Search...',
border: InputBorder.none),
),
),
)),
);
}
}
Example 2: Search Field and SliverAppBar
Searching is one of the most important features of many e-commerce apps so they usually display the search field in the most recognizable way and also take up a lot of space right from the start (Amazon, Shopee, etc).
Preview
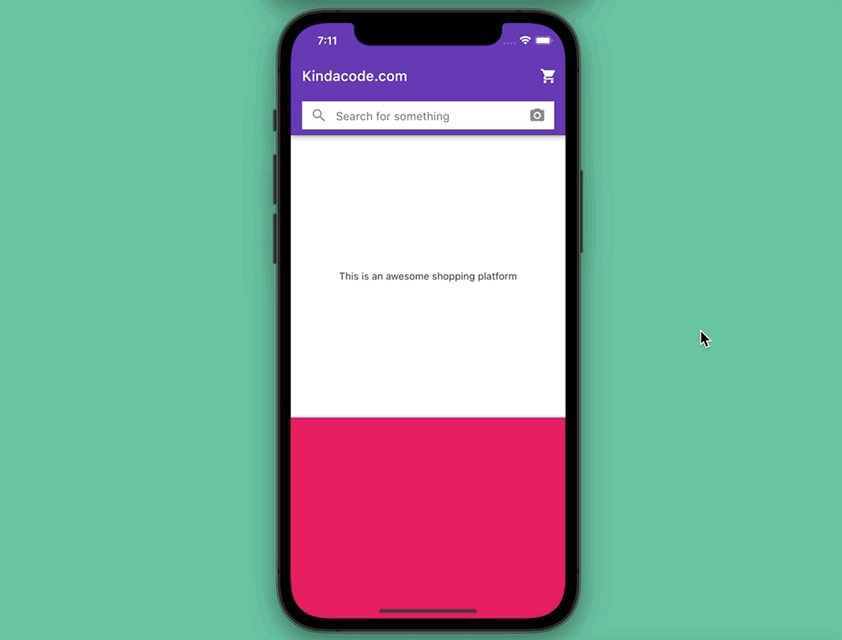
The Code
The full source:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
theme: ThemeData(
primarySwatch: Colors.deepPurple,
),
home: const HomePage());
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: CustomScrollView(
slivers: [
SliverAppBar(
floating: true,
pinned: true,
snap: false,
centerTitle: false,
title: const Text('Kindacode.com'),
actions: [
IconButton(
icon: const Icon(Icons.shopping_cart),
onPressed: () {},
),
],
bottom: AppBar(
title: Container(
width: double.infinity,
height: 40,
color: Colors.white,
child: const Center(
child: TextField(
decoration: InputDecoration(
hintText: 'Search for something',
prefixIcon: Icon(Icons.search),
suffixIcon: Icon(Icons.camera_alt)),
),
),
),
),
),
// Other Sliver Widgets
SliverList(
delegate: SliverChildListDelegate([
const SizedBox(
height: 400,
child: Center(
child: Text(
'This is an awesome shopping platform',
),
),
),
Container(
height: 1000,
color: Colors.pink,
),
]),
),
],
),
);
}
}
Recap
We’ve examined 2 common approaches to implementing a search bar in a mobile app. If you’d like to explore more new and interesting stuff about Flutter and Dart, take a look at the following articles:
- Flutter SliverList – Tutorial and Example
- Flutter & SQLite: CRUD Example
- Using GetX (Get) for State Management in Flutter
- Flutter and Firestore Database: CRUD example (null safety)
- Example of CupertinoSliverNavigationBar in Flutter
- Flutter SliverAppBar Example (with Explanations)
- Flutter: SliverGrid example
You can also take a tour around our Flutter topic page or Dart topic page for the latest tutorials and examples.