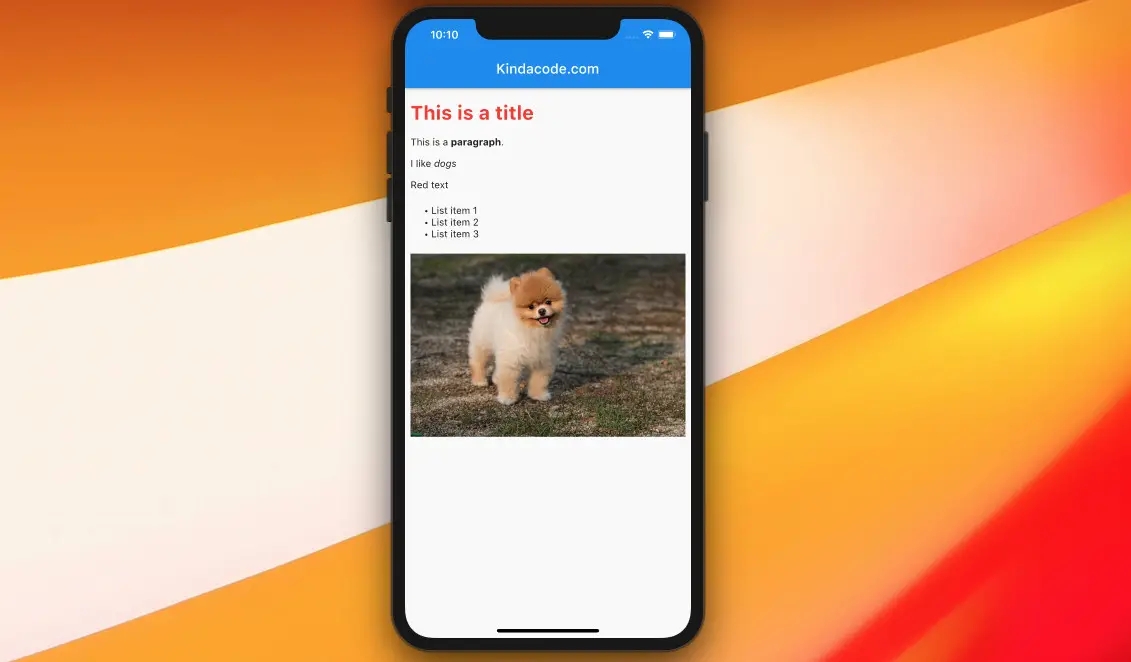
If you’re building a magazine/newspaper application (or something like that) with Flutter, you may want to render some HTML content (which usually is fetched from a remote server). This article shows you how to do so by using a plugin called flutter_html.
Sample HTML (this will be used later):
<div>
<h1>This is a title</h1>
<p>This is a <strong>paragraph</strong>.</p>
<p>I like <i>dogs</i></p>
<p style='color: red, font-size: 20px'>Red text</p>
<ul>
<li>List item 1</li>
<li>List item 2</li>
<li>List item 3</li>
</ul>
<img style='width: 300, height: 200' src='https://www.kindacode.com/wp-content/uploads/2020/11/my-dog.jpg' />
</div>
Installation
1. You can conveniently add flutter_html and its latest version to the dependencies section in your pubspec.yaml file by performing the following command:
flutter pub add flutter_html
2. Then execute the command below:
flutter pub get
Usage
The package provides a widget named Html. You can simply implement it like so:
import 'package:flutter_html/flutter_html.dart';
import 'package:flutter_html/style.dart'; // For using CSS
/* ... */
Html(
data: /* HTML source */,
// Styling with CSS (not real CSS)
style: {
/* ... */
},
)
For more clarity, see the end-to-end example below.
The Complete Example
Screenshot:

The code:
// main.dart
import 'package:flutter/material.dart';
import 'package:flutter_html/flutter_html.dart';
import 'package:flutter_html/style.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatelessWidget {
final _htmlContent = """
<div>
<h1>This is a title</h1>
<p>This is a <strong>paragraph</strong>.</p>
<p>I like <i>dogs</i></p>
<p>Red text</p>
<ul>
<li>List item 1</li>
<li>List item 2</li>
<li>List item 3</li>
</ul>
<img src='https://www.kindacode.com/wp-content/uploads/2020/11/my-dog.jpg' />
</div>
""";
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: SafeArea(
child: SingleChildScrollView(
child: Html(
data: _htmlContent,
// Styling with CSS (not real CSS)
style: {
'h1': Style(color: Colors.red),
'p': Style(color: Colors.black87, fontSize: FontSize.medium),
'ul': Style(margin: const EdgeInsets.symmetric(vertical: 20))
},
),
),
),
);
}
}
See also: How to use web view in Flutter.
Afterword
You’ve learned how to display HTML content in a Flutter app. Keep the ball rolling and continue moving forward on the mobile development journey by taking a look at the following articles:
- Viewing PDF files in Flutter
- How to encode/decode JSON in Flutter
- Flutter PaginatedDataTable Example
- How to implement Star Rating in Flutter
- How to save network images to the device in Flutter
- How to Create a Countdown Timer in Flutter
You can also check out our Flutter category page or Dart category page for the latest tutorials and examples.
THANK YOU. IM LOOKING FOR 6 HOURS OF THIS SHIT. YOU SAVED ME. THANK YOU.
getting error – Cannot run with sound null safety, because the following dependencies don’t support null safety. Any suggestion?
Make sure you are using the latest version of flutter_html (2.2.1)