A quick example that demonstrates how to use the ColorTween widget in Flutter to create a smooth transition between two colors.
Preview
This small app displays a circle whose background color changes continuously from blue to amber and from amber to blue.
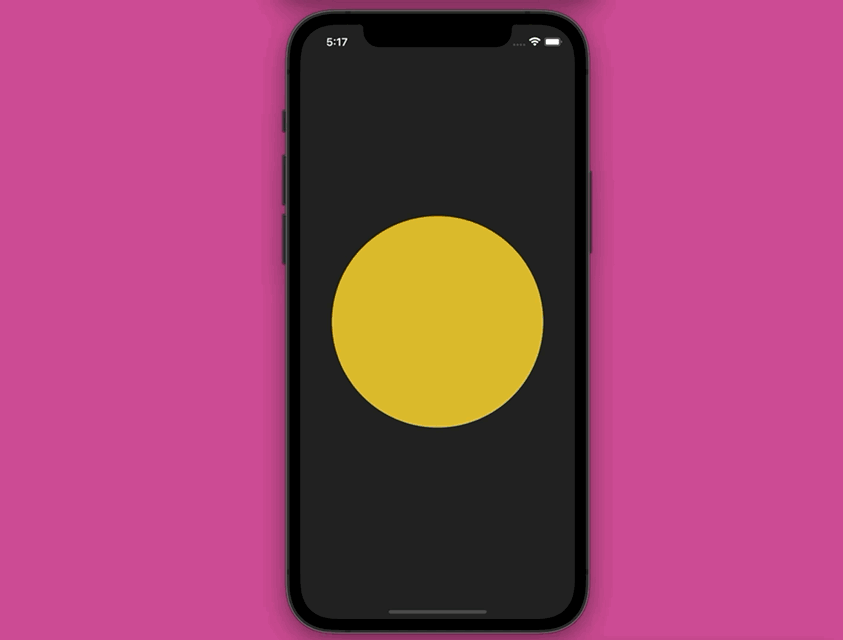
The complete code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> with TickerProviderStateMixin {
// animation controller
late AnimationController _controller;
// animation color
late Animation<Color?> _color;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(seconds: 5),
vsync: this,
)..repeat(reverse: true);
// color tween
_color =
ColorTween(begin: Colors.blue, end: Colors.amber).animate(_controller);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
alignment: Alignment.center,
color: Colors.black87,
child: AnimatedBuilder(
animation: _color,
builder: (BuildContext _, Widget? __) {
return Container(
width: 300,
height: 300,
decoration:
BoxDecoration(color: _color.value, shape: BoxShape.circle),
);
},
),
),
);
}
}
Further reading:
- Using AnimatedIcon in Flutter
- Flutter TweenAnimationBuilder Examples
- Flutter: FadeTransition example
- Flutter & SQLite: CRUD Example
- How to create Blur Effects in Flutter
- Flutter: 5 Ways to Add a Drop Shadow to a Widget
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.