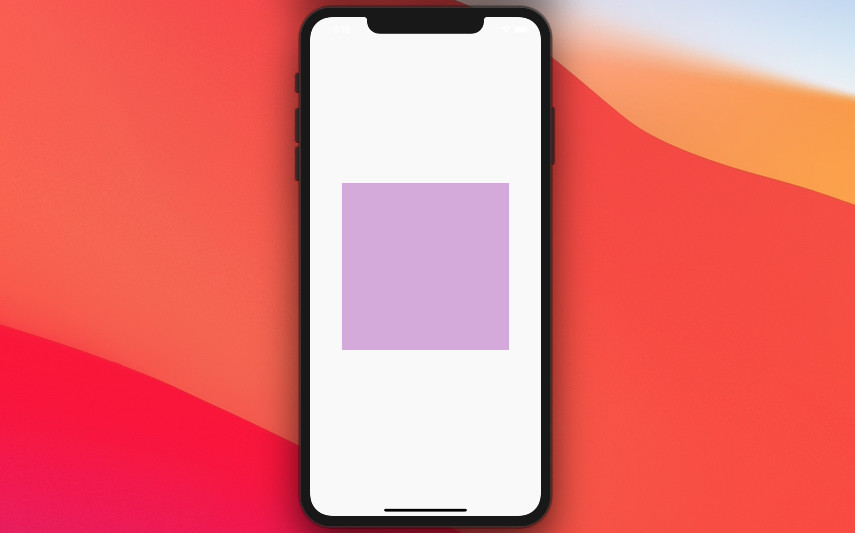
A simple example that demonstrates how to use the FadeTransition widget in Flutter to animate the opacity of its child.
App Preview
This example creates a purple box with an opacity that changes continuously over time.
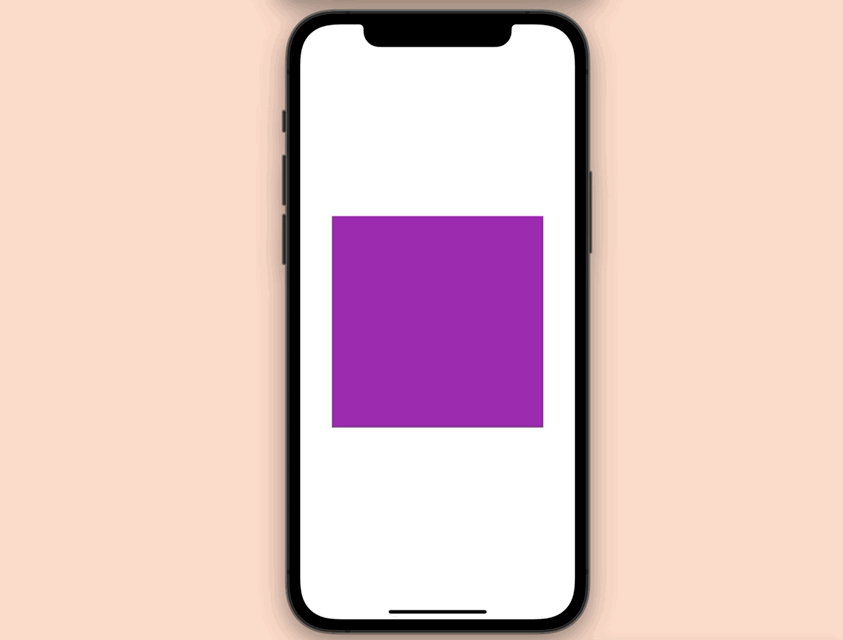
The Complete Code
// kindacode.com
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Remove the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> with TickerProviderStateMixin {
// controller
late AnimationController _controller;
// animation
late Animation<double> _animation;
@override
void initState() {
super.initState();
_controller =
AnimationController(duration: const Duration(seconds: 5), vsync: this)
..repeat(reverse: true);
_animation = Tween<double>(begin: 0, end: 1).animate(_controller);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
// implement the FadeTransiition widget
child: FadeTransition(
opacity: _animation,
child: Container(
width: 300,
height: 300,
color: Colors.purple,
),
),
));
}
}
That’s it. You can learn more about animation stuff in Flutter through the following articles:
- Using Animation Controller in Flutter
- Flutter TweenAnimationBuilder Examples
- Flutter DecoratedBoxTransition example
- Example of using AnimatedContainer in Flutter
- Flutter Transform examples – Making fancy effects
- Flutter: How to Make Spinning Animation without Plugins
- 2 Ways to Create Flipping Card Animation in Flutter
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.