A few examples of implementing TweenAnimationBuilder in Flutter.
A Pulsing Star
What we are going to do here is to create a pulsing circle star in the lonely, limitless purple universe.
App Preview
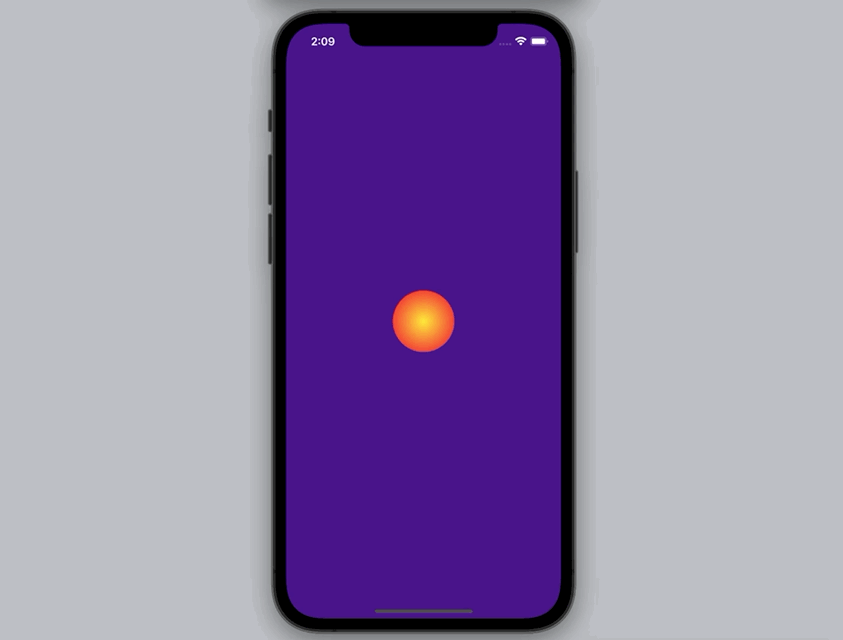
The complete code
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
double _targetSize = 300;
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
alignment: Alignment.center,
color: Colors.purple[900],
child: TweenAnimationBuilder(
tween: Tween<double>(
begin: 50,
end: _targetSize,
),
duration: const Duration(seconds: 2),
onEnd: () {
setState(() {
if (_targetSize == 50) {
_targetSize = 300;
} else {
_targetSize = 50;
}
});
},
curve: Curves.linear,
builder: (BuildContext _, double size, Widget? __) {
return Container(
width: size,
height: size,
decoration: const BoxDecoration(
gradient: RadialGradient(
center: Alignment.center,
colors: [Colors.yellow, Colors.red]),
shape: BoxShape.circle),
);
},
),
),
);
}
}
Creating an Elastic Box
This example app contains a floating button and 2 boxes. When the user presses the button, the width of the orange box switches between 100 and 300.
App Preview
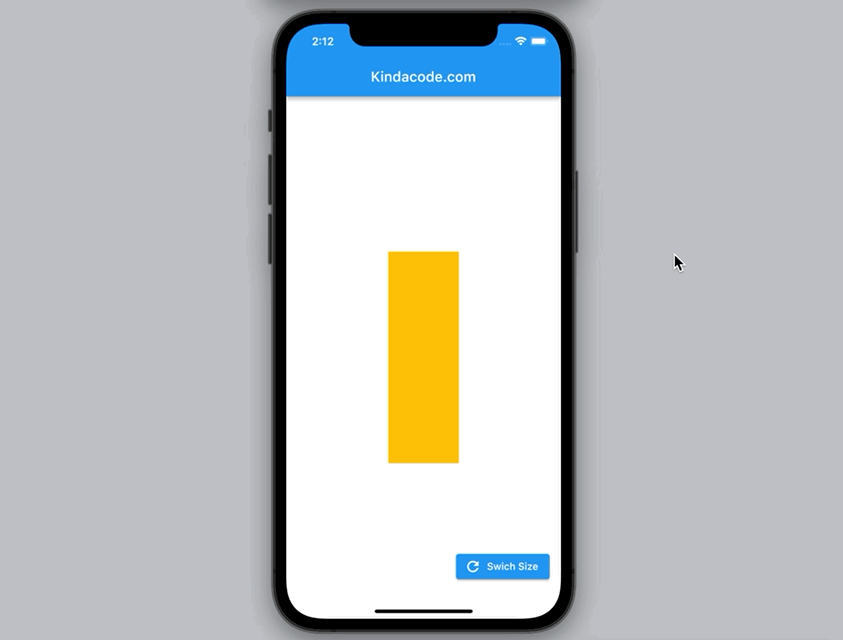
The full code
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return const MaterialApp(
// Hide the debug banner
debugShowCheckedModeBanner: false,
title: 'Kindacode.com',
home: HomePage(),
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
double _targetValue = 100;
void _changeSize() {
setState(() {
if (_targetValue == 100) {
_targetValue = 300;
} else {
_targetValue = 100;
}
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Kindacode.com'),
),
body: Center(
child: TweenAnimationBuilder(
tween: Tween<double>(
begin: 100,
end: _targetValue,
),
duration: const Duration(seconds: 1),
curve: Curves.linear,
builder: (BuildContext _, double value, Widget? __) {
return Container(
width: value,
height: 300,
color: Colors.amber,
alignment: Alignment.center,
);
},
child: Container(
width: 50,
height: 50,
color: Colors.purple,
),
),
),
floatingActionButton: ElevatedButton.icon(
onPressed: _changeSize,
icon: const Icon(Icons.refresh),
label: const Text('Swich Size')),
);
}
}
Wrapping Up
We’ve gone through a couple of examples of making cool animations by using TweenAnimationBuilder. If you’d like to explore more new and awesome features of Flutter, take a look at the following articles:
- Flutter Transform examples – Making fancy effects
- Flutter: ColorTween Example
- Flutter: How to Make Spinning Animation without Plugins
- Flutter AnimatedList – Tutorial and Examples
- Using GetX (Get) for Navigation and Routing in Flutter
- Flutter SliverAppBar Example (with Explanations)
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.