The example below is about changing the AppBar title dynamically in Flutter.
App Preview
The demo app we’re going to make has an app bar and a text field. When you enter text in the text field, the title of the app bar will change accordingly.
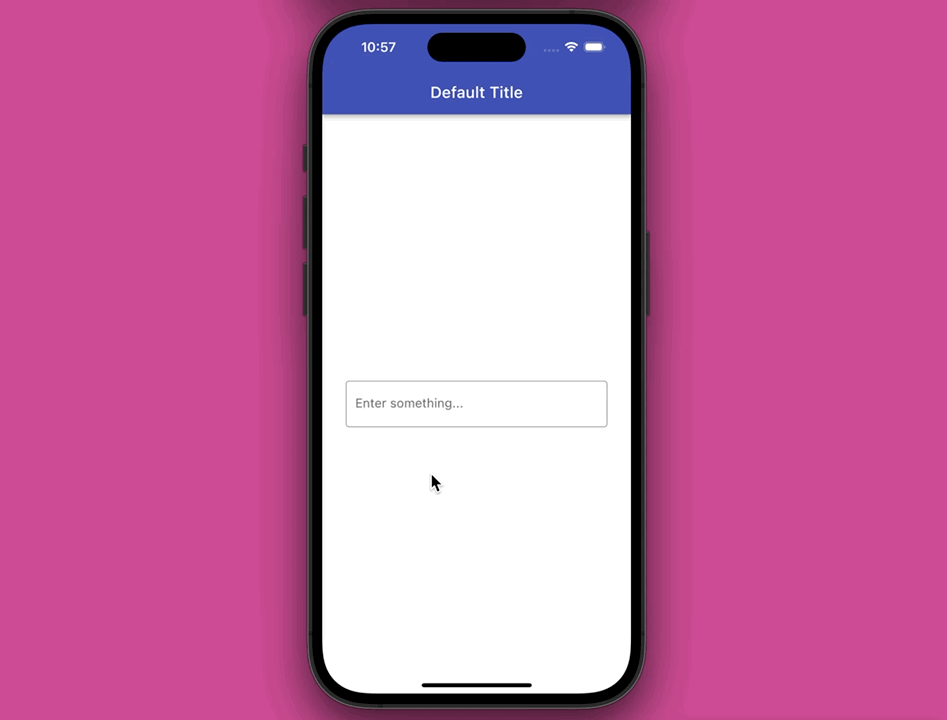
The complete code:
// main.dart
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'KindaCode.com',
theme: ThemeData(
primarySwatch: Colors.indigo,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
String _title = 'Default Title';
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(_title),
),
// the TextField widget lets the user enter text in
body: Center(
child: Padding(
padding: const EdgeInsets.all(30),
child: TextField(
onChanged: (enteredValue) {
setState(() {
_title = enteredValue;
});
},
decoration: const InputDecoration(
hintText: 'Enter something...', border: OutlineInputBorder()),
),
),
));
}
}
Further reading:
- Flutter: SliverGrid example
- Create a Custom NumPad (Number Keyboard) in Flutter
- Best Libraries for Making HTTP Requests in Flutter
- Creating Masonry Layout in Flutter with Staggered Grid View
- Flutter: Creating an Auto-Resize TextField
- Using BlockSemantics in Flutter: Tutorial & Example
You can also take a tour around our Flutter topic page and Dart topic page to see the latest tutorials and examples.